|
1 |
| -# ⚫ OOP in Tech Interviews 2024: 39 Must-Know Questions & Answers |
| 1 | +# 52 Important OOP Interview Questions |
2 | 2 |
|
3 |
| -**Object-Oriented Programming** is a paradigm centered on "objects" with data (attributes) and code (methods). Key principles include encapsulation, inheritance, polymorphism, and abstraction. In interviews, OOP questions assess candidate's understanding of design patterns, class relationships, and software organization. |
| 3 | +<div> |
| 4 | +<p align="center"> |
| 5 | +<a href="https://devinterview.io/questions/web-and-mobile-development/"> |
| 6 | +<img src="https://firebasestorage.googleapis.com/v0/b/dev-stack-app.appspot.com/o/github-blog-img%2Fweb-and-mobile-development-github-img.jpg?alt=media&token=1b5eeecc-c9fb-49f5-9e03-50cf2e309555" alt="web-and-mobile-development" width="100%"> |
| 7 | +</a> |
| 8 | +</p> |
4 | 9 |
|
5 |
| -Check out our carefully selected list of **basic** and **advanced** OOP questions and answers to be well-prepared for your tech interviews in 2024. |
| 10 | +#### You can also find all 52 answers here 👉 [Devinterview.io - OOP](https://devinterview.io/questions/web-and-mobile-development/oop-interview-questions) |
6 | 11 |
|
7 |
| -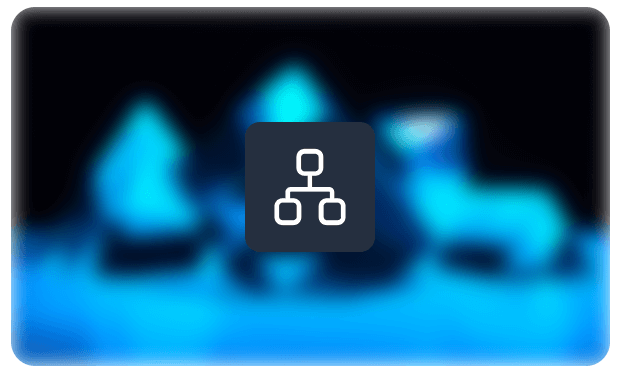 |
| 12 | +<br> |
8 | 13 |
|
9 |
| -👉🏼 You can also find all answers here: [Devinterview.io - OOP](https://devinterview.io/dev/oop-interview-questions) |
10 |
| - |
11 |
| ---- |
12 |
| - |
13 |
| -## 🔹 1. What is _Object-Oriented Programming_ (OOP)? |
14 |
| - |
15 |
| -### Answer |
| 14 | +## 1. What is _Object-Oriented Programming (OOP)_? |
16 | 15 |
|
17 | 16 | **Object-Oriented Programming** is a programming paradigm that organizes code into **self-contained objects**.
|
18 | 17 |
|
@@ -146,12 +145,9 @@ In the example:
|
146 | 145 | - **Inheritance**: Both `Lion` and `Parrot` classes inherit from the `Animal` class.
|
147 | 146 | - **Polymorphism**: Both `Lion` and `Parrot` provide their own implementation of the `makeSound()` method, even though they are treated as `Animal` objects.
|
148 | 147 | - **Abstraction**: The `Animal` class contains the abstract method `makeSound()`, ensuring derived classes provide their own implementation.
|
| 148 | +<br> |
149 | 149 |
|
150 |
| ---- |
151 |
| - |
152 |
| -## 🔹 2. What is the difference between _Procedural_ and _Object-Oriented_ programming? |
153 |
| - |
154 |
| -### Answer |
| 150 | +## 2. What is the difference between _procedural_ and _Object-Oriented_ programming? |
155 | 151 |
|
156 | 152 | **Procedural** and **Object-Oriented Programming** (OOP) are distinct programming paradigms. While procedural programming is linear and task-centric, OOP emphasizes an organic model, where data is encapsulated in objects.
|
157 | 153 |
|
@@ -236,201 +232,164 @@ public class Main {
|
236 | 232 | }
|
237 | 233 | }
|
238 | 234 | ```
|
| 235 | +<br> |
239 | 236 |
|
240 |
| ---- |
| 237 | +## 3. What is _encapsulation_? |
241 | 238 |
|
242 |
| -## 🔹 3. What is a _Class_? |
| 239 | +**Encapsulation** is an object-oriented programming principle that combines data and methods that act on that data within a single unit, known as an **object**. |
243 | 240 |
|
244 |
| -### Answer |
| 241 | +### Key Concepts |
245 | 242 |
|
246 |
| -**Class** in Object-Oriented Programming represents a blueprint for creating objects (instances). It encapsulates attributes (data) and behaviors (methods) under a unified structure. |
| 243 | +- **Information Hiding**: Encapsulation hides internal state and implementation details. |
247 | 244 |
|
248 |
| -When instantiated, each **object** can carry individual data, adhering to the blueprint provided by its class. |
| 245 | +- **Access ControL**: Data is made available to external code only through defined methods: **getters** - for reading and **setters** - for writing. |
249 | 246 |
|
250 |
| -### Key Components of a Class |
| 247 | +- **Constraining Behavior**: Access methods can ensure that data adheres to specific rules (e.g., range checks or formatting standards). |
251 | 248 |
|
252 |
| -- **Attributes**: They store the state of an object and can have varying data types. |
253 | 249 |
|
254 |
| -- **Methods**: These are functions defined within the class, designed to operate on attributes or perform specific behaviors. |
| 250 | +### Benefits of Encapsulation |
255 | 251 |
|
256 |
| -### OOP Principles in Classes |
| 252 | +- **Security**: Offers controlled access to object data, reducing the risk of data corruption or unauthorized modifications. |
257 | 253 |
|
258 |
| -- **Inheritance**: Allows a subclass (child class) to inherit attributes and methods from a superclass (parent class). |
| 254 | +- **Simplicity**: Objects abstract complex systems, presenting a simple interface for interaction. |
259 | 255 |
|
260 |
| -- **Encapsulation**: Attributes and methods are bundled within a class, limiting direct access to ensure data safety. |
261 |
| - |
262 |
| -- **Polymorphism**: Allows objects of different classes that implement the same interface to be used in a consistent manner. |
| 256 | +- **Flexibility**: Encapsulation promotes loose coupling, making it easier to modify or replace object internals without impacting the external code. |
263 | 257 |
|
264 |
| -- **Abstraction**: Hides internal details, providing a simplified interface. Achieved in classes by exposing only essential methods. |
| 258 | +### Practical Applications |
265 | 259 |
|
266 |
| -### Code Example: The Car Class |
| 260 | +- **Class Construction**: Modern programming languages like Java and C# follow an "encapsulation first" approach, utilizing access specifiers like `public`, `private`, and `protected`. |
267 | 261 |
|
268 |
| -Here is the Python code: |
| 262 | +- **API Design**: As a software developer, encapsulating classes and modules helps create intuitive and focused APIs. It allows hiding internal strategies while exposing the desired functionality. |
269 | 263 |
|
270 |
| -```python |
271 |
| -class Car: |
272 |
| - """A class to represent a car.""" |
273 |
| - |
274 |
| - def __init__(self, make, model, year): |
275 |
| - """Initialize car attributes.""" |
276 |
| - self.make = make |
277 |
| - self.model = model |
278 |
| - self.year = year |
279 |
| - self.fuel = 0 |
| 264 | +- **Testing**: Data hiding helps prevent direct access to object internals during testing, ensuring proper evaluation of object behavior through its public interface. |
280 | 265 |
|
281 |
| - def fill_tank(self, gallons): |
282 |
| - """Add fuel to the tank. Ensure the amount is positive.""" |
283 |
| - if gallons > 0: |
284 |
| - self.fuel += gallons |
285 |
| - else: |
286 |
| - print("Invalid fuel amount.") |
| 266 | +### Code Example: Encapsulation |
287 | 267 |
|
288 |
| - def drive(self, distance=1): |
289 |
| - """Drive the car, consuming fuel based on distance.""" |
290 |
| - if self.fuel >= distance: |
291 |
| - self.fuel -= distance |
292 |
| - print(f"Car drove {distance} unit(s). Remaining fuel: {self.fuel} units.") |
293 |
| - else: |
294 |
| - print("Insufficient fuel.") |
295 |
| - |
296 |
| -# Create a Car object |
297 |
| -my_car = Car("Honda", "Civic", 2022) |
298 |
| - |
299 |
| -# Access its attributes and methods |
300 |
| -my_car.fill_tank(10) |
301 |
| - |
302 |
| -for _ in range(15): |
303 |
| - my_car.drive() |
304 |
| -``` |
| 268 | +Here is the Java code: |
305 | 269 |
|
| 270 | +```java |
| 271 | +public class Car { |
| 272 | + private int fuel; // private ensures that fuel can't be accessed directly from outside the class |
306 | 273 |
|
307 |
| ---- |
| 274 | + public Car() { |
| 275 | + this.fuel = 100; // Initialize with 100 units of fuel |
| 276 | + } |
308 | 277 |
|
309 |
| -## 🔹 4. What is an _Object_? |
| 278 | + // Getter method for fuel |
| 279 | + public int getFuel() { |
| 280 | + return fuel; |
| 281 | + } |
310 | 282 |
|
311 |
| -### Answer |
| 283 | + // Setter method for fuel with encapsulation enforcing constraints |
| 284 | + public void setFuel(int fuel) { |
| 285 | + if (fuel >= 0 && fuel <= 100) { |
| 286 | + this.fuel = fuel; |
| 287 | + } else { |
| 288 | + System.out.println("Invalid fuel amount."); |
| 289 | + } |
| 290 | + } |
312 | 291 |
|
313 |
| -An **object** represents a specific instance of a **class**. It encapsulates both **data** (attributes) and **behavior** (methods) within a single unit. |
| 292 | + public void drive() { |
| 293 | + if (fuel > 0) { |
| 294 | + fuel--; |
| 295 | + System.out.println("Vroom!"); |
| 296 | + } else { |
| 297 | + System.out.println("Out of fuel!"); |
| 298 | + } |
| 299 | + } |
314 | 300 |
|
315 |
| -### Core Characteristics |
| 301 | + public static void main(String[] args) { |
| 302 | + Car myCar = new Car(); |
| 303 | + myCar.drive(); |
| 304 | + System.out.println("Fuel remaining: " + myCar.getFuel()); |
| 305 | + myCar.setFuel(120); // This will print "Invalid fuel amount." |
| 306 | + } |
| 307 | +} |
| 308 | +``` |
| 309 | +<br> |
316 | 310 |
|
317 |
| -- **Identity**: Each object has a unique identity that distinguishes it from others. |
318 |
| -- **State**: Defined by its attributes, an object's state can change throughout its existence. |
319 |
| -- **Behavior**: The methods associated with the object describe its possible actions or operations. |
| 311 | +## 4. What is _polymorphism_? Explain _overriding_ and _overloading_. |
320 | 312 |
|
321 |
| -### Lifecycle of an Object |
| 313 | +**Polymorphism** in object-oriented programming allows objects of different types to be treated as if they belong to the same type through a **shared interface**. It abstracts method details, promoting code flexibility and reusability. |
322 | 314 |
|
323 |
| -1. **Creation**: Objects are created from a class through a process called instantiation. |
324 |
| -2. **Manipulation**: They may undergo state changes as attributes are modified, and methods are invoked. |
325 |
| -3. **Destruction**: Terminates the object's existence, often handled automatically by the programming language ("garbage collection") or explicitly through code. |
| 315 | +### Core Concepts |
326 | 316 |
|
327 |
| -### Code Example: Object Instantiation |
| 317 | +#### Overloading (Compile-time Polymorphism) |
328 | 318 |
|
329 |
| -Here is the Python code: |
| 319 | +**Multiple methods** in the same class can have the same name but different parameters, allowing them to coexist. The compiler selects the appropriate method based on the method signature. |
330 | 320 |
|
331 |
| -``` python |
332 |
| -class Dog: |
333 |
| - def __init__(self, name, breed): |
334 |
| - self.name = name |
335 |
| - self.breed = breed |
| 321 | +#### Code Example: Overloading |
336 | 322 |
|
337 |
| - def bark(self): |
338 |
| - print("Woof!") |
| 323 | +Here is the Java code: |
339 | 324 |
|
340 |
| -myDog = Dog("Buddy", "Golden Retriever") |
| 325 | +```java |
| 326 | +public class Sum { |
| 327 | + public int add(int a, int b) { |
| 328 | + return a + b; |
| 329 | + } |
| 330 | + |
| 331 | + public double add(double a, double b) { |
| 332 | + return a + b; |
| 333 | + } |
| 334 | +} |
341 | 335 | ```
|
342 | 336 |
|
343 |
| ---- |
344 |
| - |
345 |
| -## 🔹 5. Explain the _Hierarchical Relationship_ between classes. |
346 |
| - |
347 |
| -### Answer |
| 337 | +#### Overriding (Runtime Polymorphism) |
348 | 338 |
|
349 |
| -In OOP, **classes** are often organized in a **hierarchical** manner through inheritance. This hierarchy establishes relationships where specific classes are built upon more general ones, refining or extending their characteristics and behaviors. |
350 |
| - |
351 |
| -### Key Concepts |
| 339 | +A **subclass** provides a specific implementation of a method that is already defined in its parent class, effectively replacing the parent's version. The method to be called is determined during the program's execution. |
352 | 340 |
|
353 |
| -- **Base and Derived Classes**: The base (or parent) class provides a foundational set of attributes and methods. Derived (or child) classes inherit from the base class, adapting or expanding upon its defined characteristics. |
354 |
| - |
355 |
| -- **Inheritance Chain**: This represents the sequence of derived classes from a base class. A derived class can itself be a base class for another, leading to multiple layers in the hierarchy. |
356 |
| - |
357 |
| -### Visual Representation |
358 |
| - |
359 |
| -.jpg?alt=media&token=f14cea00-085b-4cb8-b440-860302f0fa16&_gl=1*16lln2r*_ga*OTYzMjY5NTkwLjE2ODg4NDM4Njg.*_ga_CW55HF8NVT*MTY5ODA3NDc5OC4xNzMuMS4xNjk4MDc1MDcxLjU2LjAuMA..) |
360 |
| - |
361 |
| -### Code Example: Class Hierarchy |
| 341 | +#### Code Example: Overriding |
362 | 342 |
|
363 | 343 | Here is the Python code:
|
364 | 344 |
|
365 | 345 | ```python
|
366 | 346 | class Animal:
|
367 |
| - def __init__(self, name, age): |
368 |
| - self.name = name |
369 |
| - self.age = age |
370 |
| - |
371 |
| - def eat(self): |
372 |
| - print(f"{self.name} is eating.") |
373 |
| - |
374 |
| -class Mammal(Animal): |
375 |
| - def sleep(self): |
376 |
| - print(f"{self.name} is sleeping.") |
377 |
| - |
378 |
| -class Bird(Animal): |
379 |
| - def fly(self): |
380 |
| - print(f"{self.name} is flying.") |
381 |
| - |
382 |
| -class Lion(Mammal): |
383 |
| - def __init__(self, name, age, gender): |
384 |
| - super().__init__(name, age) |
385 |
| - self.gender = gender |
386 |
| - |
387 |
| - def roar(self): |
388 |
| - print(f"{self.name} is roaring.") |
389 |
| - |
390 |
| -class Whale(Mammal): |
391 |
| - def swim(self): |
392 |
| - print(f"{self.name} is swimming.") |
| 347 | + def speak(self): |
| 348 | + return "Animal speaks" |
| 349 | + |
| 350 | +class Cat(Animal): |
| 351 | + def speak(self): |
| 352 | + return "Cat meows" |
393 | 353 |
|
394 |
| -class Penguin(Bird): |
395 |
| - def walk(self): |
396 |
| - print(f"{self.name} is walking on land.") |
| 354 | +obj_cat = Cat() |
| 355 | +print(obj_cat.speak()) # Output: Cat meows |
397 | 356 | ```
|
398 | 357 |
|
399 |
| ---- |
| 358 | +#### Virtual Methods (in languages like C++) |
400 | 359 |
|
401 |
| -## 🔹 6. What is the difference between a _Method_ and a _Function_ in the context of _OOP_? |
| 360 | +Methods marked `virtual` in the base class can be overridden in derived classes. They enable **dynamic dispatch**, ensuring the correct method is called, even through base class references. |
402 | 361 |
|
403 |
| -### Answer |
| 362 | +#### Code Example: Virtual Methods |
404 | 363 |
|
405 |
| -**Methods** and **functions** are both means to group code for reuse in OOP, but they serve different roles based on where and how they are used. |
| 364 | +Here is the C++ code: |
406 | 365 |
|
407 |
| -While all methods are functions in a broader sense, not all functions are methods. The distinction lies in their relationship (or lack thereof) to class instances. |
| 366 | +```cpp |
| 367 | +#include <iostream> |
| 368 | +using namespace std; |
408 | 369 |
|
409 |
| -### Methods: Object-Linked Behavior |
| 370 | +class Animal { |
| 371 | +public: |
| 372 | + virtual void speak() { |
| 373 | + cout << "Animal speaks"; |
| 374 | + } |
| 375 | +}; |
410 | 376 |
|
411 |
| -- **What**: Methods are functions that are defined within a **class** and are associated with object behavior. They are designed to manipulate or interact with the class's instance data (attributes). |
412 |
| - |
413 |
| -- **Invocation**: Methods are invoked on an instance of a class using dot notation, e.g., `my_object.my_method()`. |
414 |
| - |
415 |
| -- **Access**: Methods inherently have access to the object's data and other methods. In many OOP languages, this is done through a self-reference (like `self` in Python or `this` in Java). |
416 |
| - |
417 |
| -- **Example**: Consider a `Car` class. The actions `start_engine` and `change_gear` would be methods, tailored to the state and behavior of specific car instances. |
| 377 | +class Cat : public Animal { |
| 378 | +public: |
| 379 | + void speak() override { |
| 380 | + cout << "Cat meows"; |
| 381 | + } |
| 382 | +}; |
| 383 | +``` |
418 | 384 |
|
419 |
| -### Functions: Class-Agnostic Procedures |
| 385 | +### Dynamic Dispatch and Late Binding |
420 | 386 |
|
421 |
| -- **What**: In the OOP context, functions can be defined within a class but aren't tied to an instance's behavior or data. Such functions might be static methods in some languages, meaning they relate to the class rather than any specific instance. |
422 |
| - |
423 |
| -- **Invocation**: Functions, even if part of a class, are typically called without requiring an instance. In some languages, this might require special notation, like using the `@staticmethod` decorator in Python. |
424 |
| - |
425 |
| -- **Access**: Functions within a class context don't typically access or modify instance-specific data unless passed that data explicitly. |
426 |
| - |
427 |
| -- **Example**: Inside a `Math` class, a function `square_root` would be a general utility that computes the square root of a number. It doesn't operate on instance data and doesn't need to know about specific `Math` objects. |
| 387 | +Polymorphism often leverages **dynamic dispatch**, a mechanism that determines at runtime which specific method to invoke. |
428 | 388 |
|
429 |
| ---- |
| 389 | +This is also known as **late binding**. This feature enables generic code that can handle multiple object types, enriching flexibility and adaptability. |
| 390 | +<br> |
430 | 391 |
|
431 |
| -## 🔹 7. What is _Inheritance_? Name some _Types of Inheritance_. |
432 |
| - |
433 |
| -### Answer |
| 392 | +## 5. What is _inheritance_? Name some _types of inheritance_. |
434 | 393 |
|
435 | 394 | **Inheritance** is a fundamental concept in object-oriented programming that allows for the creation of **new classes** based on existing ones.
|
436 | 395 |
|
@@ -524,302 +483,633 @@ class J extends H { } // Class J also inherits from class H
|
524 | 483 | // Java does not directly support hybrid inheritance through classes.
|
525 | 484 | // However, you can achieve something similar using interfaces, as shown with multiple inheritance.
|
526 | 485 | ```
|
| 486 | +<br> |
527 | 487 |
|
528 |
| ---- |
| 488 | +## 6. What is an _abstraction_? Name some _abstraction techniques_. |
529 | 489 |
|
530 |
| -## 🔹 8. Compare _Inheritance_ vs. _Mixin_ vs. _Composition_. |
| 490 | +**Abstraction** in object-oriented programming (OOP) is the concept of focusing on essential characteristics and hiding unnecessary details. |
531 | 491 |
|
532 |
| -### Answer |
| 492 | +It enables clear separation between high-level concepts and their specific implementations, promoting **code reusability**, **maintainability**, and **security**. |
533 | 493 |
|
534 |
| -**Inheritance**, **mixins**, and **composition** are all techniques in object-oriented programming that deal with code reuse and the relationship between objects or classes. |
| 494 | +### Abstraction Techniques |
535 | 495 |
|
536 |
| -Let's look into the details and compare them: |
| 496 | +#### Abstract Classes |
537 | 497 |
|
538 |
| -### Inheritance |
| 498 | +- **Definition**: An abstract class is a class that cannot be instantiated on its own. It serves as a foundation for other classes. |
| 499 | +- **Purpose**: Abstract classes allow you to define base behaviors that can be overridden by derived classes, while also allowing you to provide some default implementations. This means that while you define certain methods as abstract (no implementation), others can have actual code. |
| 500 | +- **Use Case**: If you have a set of functionalities where some methods have a default behavior but can be overridden, and others must be defined by any child class, you'd use an abstract class. |
539 | 501 |
|
540 |
| -- **Definition**: A class (subclass) inherits properties and behaviors from another class (superclass). |
541 |
| -- **Relationship**: "is-a" (e.g., a `Car` is a `Vehicle`). |
542 |
| -- **Pros**: Direct way to reuse code; establishes intuitive relationships. |
543 |
| -- **Cons**: Can lead to complex hierarchies; potential for over-generalization. |
| 502 | +#### Interfaces |
544 | 503 |
|
545 |
| -### Mixin |
| 504 | +- **Definition**: An interface is a contract or blueprint that classes adhere to. Unlike abstract classes, interfaces have no implementation details; they only declare method and property signatures. |
| 505 | +- **Purpose**: Interfaces ensure a certain set of methods or properties exist in the classes that implement them. They guarantee a specific structure or behavior without dictating how that behavior is achieved. |
| 506 | +- **Use Case**: When you want multiple classes to adhere to a specific contract, ensuring they all have the same methods (but possibly different implementations), you'd use an interface. |
546 | 507 |
|
547 |
| -- **Definition**: A class that offers methods to other classes without being a standalone entity. Common in languages without multiple inheritance, such as Python. |
548 |
| -- **Relationship**: "kind-of-a" or "can-do-this" (e.g., a `Helicopter` can fly like a `Bird`). |
549 |
| -- **Pros**: Reuses code across classes; avoids deep inheritance issues. |
550 |
| -- **Cons**: Method source can be unclear; potential name clashes. |
| 508 | +### Code Example: Abstraction Techniques |
551 | 509 |
|
552 |
| -### Composition |
| 510 | +Here is the Java code: |
553 | 511 |
|
554 |
| -- **Definition**: Building objects by combining simpler ones. Emphasizes a "has-a" relationship. |
555 |
| -- **Relationship**: "has-a" (e.g., a `Car` has an `Engine`). |
556 |
| -- **Pros**: Encourages modular design; components can be easily swapped. |
557 |
| -- **Cons**: May need more design upfront; can require more boilerplate code. |
| 512 | +```java |
| 513 | +// Abstract Class |
| 514 | +abstract class Vehicle { |
| 515 | + // Abstract method (no body) |
| 516 | + public abstract String start(); |
| 517 | + |
| 518 | + // Regular method |
| 519 | + public String stop() { |
| 520 | + return "Vehicle stopped!"; |
| 521 | + } |
| 522 | +} |
558 | 523 |
|
559 |
| -Many modern OOP design guidelines, like the **composition over inheritance principle**, suggest that unless there's a clear "is-a" relationship, it's often better to use **composition** as it's more flexible and leads to a more modular and maintainable design. |
| 524 | +// Implementing the abstract class |
| 525 | +class Car extends Vehicle { |
| 526 | + @Override |
| 527 | + public String start() { |
| 528 | + return "Car started!"; |
| 529 | + } |
| 530 | +} |
560 | 531 |
|
561 |
| ---- |
| 532 | +// Interface |
| 533 | +interface Drivable { |
| 534 | + // All methods in an interface are implicitly abstract |
| 535 | + void drive(); |
| 536 | +} |
562 | 537 |
|
563 |
| -## 🔹 9. Can you inherit _Private Members_ of a _Class_? |
| 538 | +// Implementing both the abstract class and the interface |
| 539 | +class Bike extends Vehicle implements Drivable { |
| 540 | + @Override |
| 541 | + public String start() { |
| 542 | + return "Bike started!"; |
| 543 | + } |
564 | 544 |
|
565 |
| -### Answer |
| 545 | + @Override |
| 546 | + public void drive() { |
| 547 | + System.out.println("Bike is being driven!"); |
| 548 | + } |
| 549 | +} |
| 550 | + |
| 551 | +public class Main { |
| 552 | + public static void main(String[] args) { |
| 553 | + Car car = new Car(); |
| 554 | + System.out.println(car.start()); // Output: Car started! |
| 555 | + System.out.println(car.stop()); // Output: Vehicle stopped! |
| 556 | + |
| 557 | + Bike bike = new Bike(); |
| 558 | + System.out.println(bike.start()); // Output: Bike started! |
| 559 | + bike.drive(); // Output: Bike is being driven! |
| 560 | + } |
| 561 | +} |
| 562 | +``` |
| 563 | +<br> |
| 564 | + |
| 565 | +## 7. What is a _class_ in OOP? |
| 566 | + |
| 567 | +**Class** in Object-Oriented Programming represents a blueprint for creating objects (instances). It encapsulates attributes (data) and behaviors (methods) under a unified structure. |
566 | 568 |
|
567 |
| -While both **public** and **protected** members can be inherited by derived classes in **OOP**, this is **not** the case for **private** members. |
| 569 | +When instantiated, each **object** can carry individual data, adhering to the blueprint provided by its class. |
568 | 570 |
|
569 |
| -In most OOP languages these members are **not accessible** outside their declaring class. |
| 571 | +### Key Components of a Class |
| 572 | + |
| 573 | +- **Attributes**: They store the state of an object and can have varying data types. |
570 | 574 |
|
571 |
| ---- |
| 575 | +- **Methods**: These are functions defined within the class, designed to operate on attributes or perform specific behaviors. |
572 | 576 |
|
573 |
| -## 🔹 10. Can a _Class_ inherit the _Constructor_ of its _Base Class_? |
| 577 | +### OOP Principles in Classes |
574 | 578 |
|
575 |
| -### Answer |
| 579 | +- **Inheritance**: Allows a subclass (child class) to inherit attributes and methods from a superclass (parent class). |
576 | 580 |
|
577 |
| -While traditionally **constructors are not inherited** by derived classes, some languages offer mechanisms to manage constructor behavior, such as **constructor chaining**. |
| 581 | +- **Encapsulation**: Attributes and methods are bundled within a class, limiting direct access to ensure data safety. |
578 | 582 |
|
579 |
| -### Constructor Inheritance in Different Languages |
| 583 | +- **Polymorphism**: Allows objects of different classes that implement the same interface to be used in a consistent manner. |
580 | 584 |
|
581 |
| -- **C++**: Constructors aren't inherited, but you can use constructor initializer lists to invoke a base class constructor. |
| 585 | +- **Abstraction**: Hides internal details, providing a simplified interface. Achieved in classes by exposing only essential methods. |
| 586 | + |
| 587 | +### Code Example: The Car Class |
| 588 | + |
| 589 | +Here is the Python code: |
| 590 | + |
| 591 | +```python |
| 592 | +class Car: |
| 593 | + """A class to represent a car.""" |
| 594 | + |
| 595 | + def __init__(self, make, model, year): |
| 596 | + """Initialize car attributes.""" |
| 597 | + self.make = make |
| 598 | + self.model = model |
| 599 | + self.year = year |
| 600 | + self.fuel = 0 |
| 601 | + |
| 602 | + def fill_tank(self, gallons): |
| 603 | + """Add fuel to the tank. Ensure the amount is positive.""" |
| 604 | + if gallons > 0: |
| 605 | + self.fuel += gallons |
| 606 | + else: |
| 607 | + print("Invalid fuel amount.") |
| 608 | + |
| 609 | + def drive(self, distance=1): |
| 610 | + """Drive the car, consuming fuel based on distance.""" |
| 611 | + if self.fuel >= distance: |
| 612 | + self.fuel -= distance |
| 613 | + print(f"Car drove {distance} unit(s). Remaining fuel: {self.fuel} units.") |
| 614 | + else: |
| 615 | + print("Insufficient fuel.") |
| 616 | + |
| 617 | +# Create a Car object |
| 618 | +my_car = Car("Honda", "Civic", 2022) |
| 619 | + |
| 620 | +# Access its attributes and methods |
| 621 | +my_car.fill_tank(10) |
| 622 | + |
| 623 | +for _ in range(15): |
| 624 | + my_car.drive() |
| 625 | +``` |
| 626 | + |
| 627 | +<br> |
| 628 | + |
| 629 | +## 8. What is an _object_ in OOP? |
| 630 | + |
| 631 | +An **object** represents a specific instance of a **class**. It encapsulates both **data** (attributes) and **behavior** (methods) within a single unit. |
| 632 | + |
| 633 | +### Core Characteristics |
| 634 | + |
| 635 | +- **Identity**: Each object has a unique identity that distinguishes it from others. |
| 636 | +- **State**: Defined by its attributes, an object's state can change throughout its existence. |
| 637 | +- **Behavior**: The methods associated with the object describe its possible actions or operations. |
| 638 | + |
| 639 | +### Lifecycle of an Object |
| 640 | + |
| 641 | +1. **Creation**: Objects are created from a class through a process called instantiation. |
| 642 | +2. **Manipulation**: They may undergo state changes as attributes are modified, and methods are invoked. |
| 643 | +3. **Destruction**: Terminates the object's existence, often handled automatically by the programming language ("garbage collection") or explicitly through code. |
| 644 | + |
| 645 | +### Code Example: Object Instantiation |
| 646 | + |
| 647 | +Here is the Python code: |
| 648 | + |
| 649 | +``` python |
| 650 | +class Dog: |
| 651 | + def __init__(self, name, breed): |
| 652 | + self.name = name |
| 653 | + self.breed = breed |
| 654 | + |
| 655 | + def bark(self): |
| 656 | + print("Woof!") |
| 657 | + |
| 658 | +myDog = Dog("Buddy", "Golden Retriever") |
| 659 | +``` |
| 660 | +<br> |
| 661 | + |
| 662 | +## 9. How do _access specifiers_ work and what are they typically? |
| 663 | + |
| 664 | +In Object-Oriented Programming (OOP), **access specifiers** define the level of visibility and accessibility of class members. |
| 665 | + |
| 666 | +### Types of Access Specifiers |
| 667 | + |
| 668 | +1. **Private**: Members are only accessible within the defining class, ensuring data encapsulation. |
| 669 | +2. **Protected**: Members are accessible within the defining class and its subclasses. |
| 670 | +3. **Public**: Members are globally accessible to all classes. |
| 671 | + |
| 672 | +### Code Example: Access Specifiers |
| 673 | + |
| 674 | +Here is the Java code: |
| 675 | + |
| 676 | +```java |
| 677 | +public class Car { |
| 678 | + private String make; // Accessible only within class |
| 679 | + protected int year; // Accessible within class and subclasses |
| 680 | + double price; // Default visibility: package-private |
| 681 | + |
| 682 | + public void setMake(String make) { |
| 683 | + this.make = make; |
| 684 | + } |
| 685 | + |
| 686 | + public String getMake() { |
| 687 | + return make; |
| 688 | + } |
| 689 | + |
| 690 | + protected void startEngine() { |
| 691 | + System.out.println("Engine started!"); |
| 692 | + } |
| 693 | +} |
| 694 | +``` |
| 695 | + |
| 696 | +### Key Concepts |
| 697 | + |
| 698 | +- **Data Encapsulation**: It ensures data integrity by hiding the class's internal state and **exposing it through methods**. This enables better control over the data and its access. |
| 699 | + |
| 700 | +- **Inheritance and Abstraction**: Access levels regulate visibility in the context of inheritance, allowing classes to interact while preserving encapsulation. |
| 701 | + |
| 702 | +### Common Mistakes |
| 703 | + |
| 704 | +- **Excessive Use of Getter/Setter Pairs**: While they are useful for protecting data, avoid introducing methods that do not provide added functionality. |
582 | 705 |
|
583 |
| -- **Java**: Constructors aren't inherited, but if a derived class constructor doesn't explicitly invoke a base class constructor, the no-argument base class constructor is called automatically. |
| 706 | +- **Needless Use of Public Members**: If a member does not require global access, consider using more restrictive access levels for better encapsulation. |
584 | 707 |
|
585 |
| -- **Python**: Making use of the `super()` mechanism ensures appropriate base class constructor invocations across the inheritance hierarchy. |
| 708 | +### Best Practices |
586 | 709 |
|
587 |
| -- **C#**: Constructors are not inherited, but derived classes can use the `base` keyword to call a constructor from the base class. If a derived class does not explicitly define any constructors, the default constructor of the base class is automatically invoked. |
| 710 | +- **Favor Composition Over Inheritance**: If classes are loosely related, hiding details and components ensures better code maintenance. |
588 | 711 |
|
589 |
| -- **Swift**: Constructors aren't inherited. However, Swift introduces designated and convenience initializers for class construction and proper initialization across inheritance. |
| 712 | +- **Private by Default**: Encapsulate whenever possible. By default, members should be private, and their visibility be broadened only if necessary. |
590 | 713 |
|
591 |
| -- **Kotlin**: Constructors aren't inherited, but derived classes need to invoke the base class constructor using the `super` keyword. |
| 714 | +- **Minimal Use of Public Members**: Use them sparingly for elements that genuinely need global access. |
592 | 715 |
|
593 |
| ---- |
594 |
| -## 🔹 11. How to prevent a _Class_ to be _Inherited_ further? |
| 716 | +- **Consistent Visibility**: Aim for uniform visibility within a class to avoid confusion. |
| 717 | +<br> |
595 | 718 |
|
596 |
| -### Answer |
| 719 | +## 10. Name some ways to _overload_ a _method_. |
597 | 720 |
|
598 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 721 | +Let's look at three common techniques to **overload** a method. |
599 | 722 |
|
600 |
| ---- |
| 723 | +### 1. Changing Parameter Number |
601 | 724 |
|
602 |
| -## 🔹 12. What is _Encapsulation_? |
| 725 | +This method involves altering the number of parameters in different method signatures. |
603 | 726 |
|
604 |
| -### Answer |
| 727 | +Here is an example in Java: |
605 | 728 |
|
606 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 729 | +```java |
| 730 | +public int calculateSum(int a, int b) { // Two parameters |
| 731 | + return a + b; |
| 732 | +} |
607 | 733 |
|
608 |
| ---- |
| 734 | +public int calculateSum(int a, int b, int c) { // Three parameters |
| 735 | + return a + b + c; |
| 736 | +} |
| 737 | +``` |
609 | 738 |
|
610 |
| -## 🔹 13. What is _Polymorphism_? Explain _Overriding_ and _Overloading_. |
| 739 | +### 2. Adapting Parameter Order |
611 | 740 |
|
612 |
| -### Answer |
| 741 | +Another overloading technique involves rearranging the **order of parameters** in methods to create unique signatures. |
613 | 742 |
|
614 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 743 | +Here is an example in Java: |
615 | 744 |
|
616 |
| ---- |
| 745 | +```java |
| 746 | +public double calculateArea(double length, double width) { // Length, then width |
| 747 | + return length * width; |
| 748 | +} |
617 | 749 |
|
618 |
| -## 🔹 14. Name some ways to _Overload_ a _Method_. |
| 750 | +public double calculateArea(double radius) { // Just radius for a circle |
| 751 | + return Math.PI * radius * radius; |
| 752 | +} |
| 753 | +``` |
619 | 754 |
|
620 |
| -### Answer |
| 755 | +### 3. Varying Parameter Type |
621 | 756 |
|
622 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 757 | +You can define methods with different types of parameters to enable **overloading**. |
623 | 758 |
|
624 |
| ---- |
| 759 | +Here is an example in Java: |
625 | 760 |
|
626 |
| -## 🔹 15. What is an _Abstraction_? Name some _Abstraction Techniques_. |
| 761 | +```java |
| 762 | +public void printDetails(String name, int age) { |
| 763 | + System.out.println("Name: " + name + " , Age: " + age); |
| 764 | +} |
| 765 | + |
| 766 | +public void printDetails(int id) { |
| 767 | + System.out.println("ID: " + id); |
| 768 | +} |
| 769 | + |
| 770 | +public void printDetails(double salary) { |
| 771 | + System.out.println("Salary: " + salary); |
| 772 | +} |
| 773 | +``` |
| 774 | +<br> |
| 775 | + |
| 776 | +## 11. What is _cohesion_ in OOP? |
| 777 | + |
| 778 | +**Cohesion** in OOP refers to how closely the methods and data within a single class are related to one another. A highly cohesive class is focused on a specific task or responsibility, making it easier to maintain, understand, and ensure reliability. |
| 779 | + |
| 780 | +**High cohesion** is a desired attribute because it means that methods and properties within a class work together in a unified manner. In contrast, **low cohesion** indicates that a class has multiple, often unrelated responsibilities, making it harder to understand and maintain. |
627 | 781 |
|
628 |
| -### Answer |
| 782 | +### Levels of Cohesion |
629 | 783 |
|
630 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 784 | +1. **Coincidental**: Methods and properties within the class have no meaningful relationship. |
| 785 | +2. **Logical**: Methods are grouped based on some logic but lack a clear theme. |
| 786 | +3. **Temporal**: Methods are related by when they are executed, e.g., initialization methods. |
| 787 | +4. **Procedural**: Methods are executed in a specific sequence. |
| 788 | +5. **Communicational**: Methods work on the same set of data. |
| 789 | +6. **Sequential**: The output of one method serves as the input for another. |
| 790 | +7. **Functional**: All methods in the class contribute to a single well-defined task. |
631 | 791 |
|
632 |
| ---- |
| 792 | +Of these, functional cohesion is the most desirable, as it closely aligns with the **Single Responsibility Principle**. |
633 | 793 |
|
634 |
| -## 🔹 16. What is an _Abstract Class_? |
| 794 | +### Code Example: Low Cohesion Levels |
635 | 795 |
|
636 |
| -### Answer |
| 796 | +Here is the Java code: |
637 | 797 |
|
638 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 798 | +```java |
| 799 | +public class FileUtility { |
639 | 800 |
|
640 |
| ---- |
| 801 | + public String readFile(String fileName) { |
| 802 | + // Read a file |
| 803 | + return content; |
| 804 | + } |
641 | 805 |
|
642 |
| -## 🔹 17. What is an _Interface_? |
| 806 | + public void writeToDatabase(String data) { |
| 807 | + // Write content to a database |
| 808 | + } |
643 | 809 |
|
644 |
| -### Answer |
| 810 | + public void clearCache() { |
| 811 | + // Clear application cache |
| 812 | + } |
645 | 813 |
|
646 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 814 | + public List<String> parseFile(String content) { |
| 815 | + // Parse file content |
| 816 | + return parsedData; |
| 817 | + } |
| 818 | +} |
| 819 | +``` |
647 | 820 |
|
648 |
| ---- |
| 821 | +This `FileUtility` class exhibits low cohesion as it mixes file operations, database writing, and cache management. |
649 | 822 |
|
650 |
| -## 🔹 18. When to use _Interface_ vs. _Base Class_? |
| 823 | +### Recommendations for Improving Cohesion |
651 | 824 |
|
652 |
| -### Answer |
| 825 | +1. **Single Responsibility Principle (SRP)**: Each class should have only one reason to change. This principle suggests that a class should focus on one task or responsibility. |
| 826 | +2. **Encapsulation**: Encourage data hiding, and expose data only through focused and related methods. |
| 827 | +<br> |
653 | 828 |
|
654 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 829 | +## 12. What is _coupling_ in OOP? |
655 | 830 |
|
656 |
| ---- |
| 831 | +**Coupling** in OOP describes the degree of interdependence between classes or modules. It determines how closely different modules or classes are linked to each other, impacting the system's flexibility, maintainability, and testability. |
657 | 832 |
|
658 |
| -## 🔹 19. Why can't _Static Methods_ in _C#_ implement an _Interface_? |
| 833 | +**Loose coupling** is generally preferred in software design. |
659 | 834 |
|
660 |
| -### Answer |
| 835 | +### Types of Coupling |
661 | 836 |
|
662 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 837 | +1. **Content Coupling**: This is the strongest form of coupling where one module directly accesses or modifies another module's internal data. |
663 | 838 |
|
664 |
| ---- |
| 839 | +2. **Common Coupling**: Multiple modules share access to common global data. Any change to this shared resource can affect all the modules that depend on it. |
665 | 840 |
|
666 |
| -## 🔹 20. Can a _Non-static_ method be overridden as _Static_? |
| 841 | +3. **Control Coupling**: One module controls the flow of another by passing it control information, such as using flags. |
667 | 842 |
|
668 |
| -### Answer |
| 843 | +4. **External Coupling**: Classes or modules are linked by external factors, such as configuration files or data schemas. |
669 | 844 |
|
670 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 845 | +5. **Stamp (or Data) Coupling**: Modules share data structures and use only parts of them, requiring knowledge about the structure of the data being passed. |
671 | 846 |
|
672 |
| ---- |
| 847 | +6. **Message Coupling**: The lowest form of coupling where modules communicate only through standard interfaces, such as method calls or messages. |
673 | 848 |
|
674 |
| -## 🔹 21. Can you set _Accessibility Modifiers_ for methods within an _Interface_? |
| 849 | +### Relationship with SOLID Principles |
675 | 850 |
|
676 |
| -### Answer |
| 851 | +- **Single Responsibility Principle (SRP)**: Adhering to SRP typically results in **low coupling** since classes have a singular focus, thereby minimizing dependencies. |
677 | 852 |
|
678 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 853 | +- **Open-Closed Principle (OCP)**: Emphasizing extensibility without modification, OCP reduces the risk of **tight coupling** as extensions are typically made through interfaces or abstract classes. |
679 | 854 |
|
680 |
| ---- |
| 855 | +- **Liskov Substitution Principle (LSP)**: When derived classes can replace their base classes without side effects, there's often a **reduction in coupling**, ensuring modules can operate independently of the specific derived class in use. |
681 | 856 |
|
682 |
| -## 🔹 22. Explain the concepts of _Constructor_ and _Destructor_. |
| 857 | +- **Interface Segregation Principle (ISP)**: By endorsing focused interfaces rather than "one-size-fits-all" ones, ISP naturally leads to **decreased coupling** as classes aren't forced to depend on methods they don't use. |
683 | 858 |
|
684 |
| -### Answer |
| 859 | +- **Dependency Inversion Principle (DIP)**: By relying on abstractions rather than concrete implementations, DIP promotes **low coupling**, making systems more modular and adaptable. |
| 860 | +<br> |
685 | 861 |
|
686 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 862 | +## 13. What is a _constructor_ and how is it used? |
687 | 863 |
|
688 |
| ---- |
| 864 | +A **constructor** is a special method in **object-oriented programming** used for initializing objects. It ensures that **newly created** objects are set up with appropriate initial state and any required resources. |
689 | 865 |
|
690 |
| -## 🔹 23. What is a _Static Constructor_? |
| 866 | +### Key Concepts |
691 | 867 |
|
692 |
| -### Answer |
| 868 | +#### Purpose |
693 | 869 |
|
694 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 870 | +- A constructor ensures that an object is in a consistent state when created. |
| 871 | +- It initializes and configures the object's attributes or fields. |
695 | 872 |
|
696 |
| ---- |
| 873 | +#### Characteristics |
697 | 874 |
|
698 |
| -## 🔹 24. Why might a _Destructor_ in a _C#_ class not execute? |
| 875 | +- **Matches Object Definitions**: Each class defines one or more contructors for its objects. |
| 876 | +- **Has a Class Name**: Constructors are named after the class, making them easy to identify. |
| 877 | +- **No Return Type**: They don't return a value, not even `void`. |
| 878 | +- **Automatic Invocation**: They are invoked or 'called' when an object is created. |
699 | 879 |
|
700 |
| -### Answer |
| 880 | +#### Types of Constructors |
701 | 881 |
|
702 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 882 | +1. **Default Constructors**: |
| 883 | + - No parameters. |
| 884 | + - Automatically provided by the language if no constructor is defined. |
| 885 | + |
| 886 | +2. **Parameterized Constructors**: |
| 887 | + - Accept parameters for custom initialization. |
| 888 | + - Often used to provide initial values to internal attributes or fields. |
703 | 889 |
|
704 |
| ---- |
| 890 | +3. **Copy Constructors**: |
| 891 | + - Accept another object of the same type and initialize the current object using values from it. |
| 892 | + - Commonly used for deep copying in languages like C++. |
705 | 893 |
|
706 |
| -## 🔹 25. What is the difference between a _Class_ and a _Structure_? |
| 894 | +4. **Static Constructors**: |
| 895 | + - Used to initialize static member variables or for other class-level actions. |
| 896 | + - They don't take part in the creation of objects and can be absent in many OOP languages. |
707 | 897 |
|
708 |
| -### Answer |
| 898 | +#### Code Examples: Constructors |
709 | 899 |
|
710 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 900 | +Here is the Java code: |
711 | 901 |
|
712 |
| ---- |
| 902 | +```java |
| 903 | +class Vehicle { |
| 904 | + private String vehicleType; |
| 905 | + private int wheels; |
| 906 | + |
| 907 | + // Default Constructor |
| 908 | + public Vehicle() { |
| 909 | + vehicleType = "Car"; |
| 910 | + wheels = 4; |
| 911 | + } |
713 | 912 |
|
714 |
| -## 🔹 26. What is the difference between _Virtual method_ and _Abstract method_? |
| 913 | + // Parameterized Constructor |
| 914 | + public Vehicle(String type, int wheelCount) { |
| 915 | + vehicleType = type; |
| 916 | + wheels = wheelCount; |
| 917 | + } |
715 | 918 |
|
716 |
| -### Answer |
| 919 | + public void showDetails() { |
| 920 | + System.out.println("Type of Vehicle: " + vehicleType); |
| 921 | + System.out.println("Number of Wheels: " + wheels); |
| 922 | + } |
717 | 923 |
|
718 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 924 | + public static void main(String[] args) { |
| 925 | + // Calling Default Constructor |
| 926 | + Vehicle car = new Vehicle(); |
| 927 | + car.showDetails(); |
719 | 928 |
|
720 |
| ---- |
| 929 | + // Calling Parameterized Constructor |
| 930 | + Vehicle bike = new Vehicle("Bike", 2); |
| 931 | + bike.showDetails(); |
| 932 | + } |
| 933 | +} |
| 934 | +``` |
721 | 935 |
|
722 |
| -## 🔹 27. What is the purpose of a _Virtual keyword_? |
| 936 | +Here is a C++ code: |
723 | 937 |
|
724 |
| -### Answer |
| 938 | +```cpp |
| 939 | +#include <iostream> |
| 940 | +using namespace std; |
725 | 941 |
|
726 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 942 | +class Person { |
| 943 | + private: |
| 944 | + string name; |
| 945 | + int age; |
727 | 946 |
|
728 |
| ---- |
| 947 | + public: |
| 948 | + // Parameterized Constructor |
| 949 | + Person(string n, int a) { |
| 950 | + name = n; |
| 951 | + age = a; |
| 952 | + } |
729 | 953 |
|
730 |
| -## 🔹 28. What is _Cohesion_ in OOP? |
| 954 | + void displayInfo() { |
| 955 | + cout << "Name: " << name << ", Age: " << age << endl; |
| 956 | + } |
| 957 | +}; |
731 | 958 |
|
732 |
| -### Answer |
| 959 | +int main() { |
| 960 | + // Calling Parameterized Constructor |
| 961 | + Person p1 = Person("Alice", 25); |
| 962 | + p1.displayInfo(); |
733 | 963 |
|
734 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 964 | + return 0; |
| 965 | +} |
| 966 | +``` |
735 | 967 |
|
736 |
| ---- |
| 968 | +Here is a C# code: |
737 | 969 |
|
738 |
| -## 🔹 29. What is _Coupling_ in OOP? |
| 970 | +```csharp |
| 971 | +using System; |
739 | 972 |
|
740 |
| -### Answer |
| 973 | +class Car { |
| 974 | + private string model; |
741 | 975 |
|
742 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 976 | + // Parameterized Constructor |
| 977 | + public Car(string m) { |
| 978 | + model = m; |
| 979 | + } |
743 | 980 |
|
744 |
| ---- |
| 981 | + static void Main() { |
| 982 | + // Calling Parameterized Constructor |
| 983 | + Car c = new Car("Toyota"); |
| 984 | + Console.WriteLine("Car Model: " + c.model); |
| 985 | + } |
| 986 | +} |
| 987 | +``` |
| 988 | +<br> |
745 | 989 |
|
746 |
| -## 🔹 30. What is the difference between _Cohesion_ and _Coupling_? |
| 990 | +## 14. Describe the concept of _destructor_ or _finalizer_ in OOP. |
747 | 991 |
|
748 |
| -### Answer |
| 992 | +**Destructors**, or class **finalizers**, are used in **object-oriented programming** to perform cleanup actions before an object is destroyed. |
749 | 993 |
|
750 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 994 | +### Object Destruction |
751 | 995 |
|
752 |
| ---- |
| 996 | +Objects can be **explicitly** or **implicitly** destroyed: |
753 | 997 |
|
754 |
| -## 🔹 31. How do _Association_, _Aggregation_, and _Composition_ differ in OOP? |
| 998 | +- **Explicit**: The programmer calls a destructing function or method. Languages like C++ use a destructor. |
| 999 | +- **Implicit**: The language or environment manages object destruction. Garbage collectors in Java, C#, and Python are examples. |
755 | 1000 |
|
756 |
| -### Answer |
| 1001 | +### Destructors |
757 | 1002 |
|
758 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 1003 | +A **destructor** is a special method that is automatically called right before an object goes out of scope or is explicitly destroyed. Its primary purpose is to release resources or perform other cleanup tasks, like closing files or releasing memory. |
759 | 1004 |
|
760 |
| ---- |
| 1005 | +#### Use Cases |
761 | 1006 |
|
762 |
| -## 🔹 32. What is _Liskov Substitution Principle_ (LSP)? Provide some examples of _Violation_ and _Adherence_. |
| 1007 | +- **Resource Management**: Ensuring timely release of system resources. |
| 1008 | +- **Memory Management**: Helpful in non-garbage collected languages to prevent memory leaks. |
| 1009 | +- **Logging Exit or Cleanup Actions**: Commonly used to log an object's destruction or clean-up steps. |
763 | 1010 |
|
764 |
| -### Answer |
| 1011 | +### Garbage Collection |
765 | 1012 |
|
766 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 1013 | +For memory management, garbage collection is a mechanism that specifically targets automatic memory reclamation. In languages like Java and C#, destructors may not be necessary due to reliable garbage collectors. |
767 | 1014 |
|
768 |
| ---- |
| 1015 | +#### Note for Java Developers |
769 | 1016 |
|
770 |
| -## 🔹 33. Whait is _Repository Pattern_? Name some of it's benefits. |
| 1017 | +Java introduced the `finalize()` method, which is similar to a destructor. It's often discouraged in favor of using the `AutoCloseable` interface and the `try-with-resources` statement for enhanced resource management. |
771 | 1018 |
|
772 |
| -### Answer |
| 1019 | +#### Code Example: C++ |
773 | 1020 |
|
774 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 1021 | +Here is the C++ code: |
775 | 1022 |
|
776 |
| ---- |
| 1023 | +```cpp |
| 1024 | +class Resource { |
| 1025 | +public: |
| 1026 | + Resource() { std::cout << "Resource Acquired\n"; } |
| 1027 | + ~Resource() { std::cout << "Resource Destroyed\n"; } |
| 1028 | +}; |
777 | 1029 |
|
778 |
| -## 🔹 34. How _Monads_ can be useful in _OOP_? |
| 1030 | +int main() { |
| 1031 | + Resource r; |
| 1032 | +} // Destructs r here |
| 1033 | +``` |
779 | 1034 |
|
780 |
| -### Answer |
| 1035 | +### Non-Memory Resources |
781 | 1036 |
|
782 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 1037 | +Destructors can manage a variety of resources in addition to memory, such as: |
783 | 1038 |
|
784 |
| ---- |
| 1039 | +- **Files**: Ensuring files are closed to avoid data loss. |
| 1040 | +- **Network Connections**: Closing sockets to prevent resource leaks. |
| 1041 | +- **Database Connections**: Helpful in releasing database locks or sessions. |
| 1042 | +- **Hardware Devices**: For devices like cameras or sensors, freeing resources ensures they are available for other applications. |
785 | 1043 |
|
786 |
| -## 🔹 35. What is a _Unit Of Work_? |
| 1044 | +#### Code Example: Resource Management |
787 | 1045 |
|
788 |
| -### Answer |
| 1046 | +Here is the C++ code: |
789 | 1047 |
|
790 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 1048 | +```cpp |
| 1049 | +#include <iostream> |
| 1050 | +#include <fstream> |
791 | 1051 |
|
792 |
| ---- |
| 1052 | +class FileHandler { |
| 1053 | +public: |
| 1054 | + std::ofstream file; |
| 1055 | + FileHandler(std::string fileName) { |
| 1056 | + file.open(fileName); |
| 1057 | + if (file.is_open()) { |
| 1058 | + std::cout << "File Opened\n"; |
| 1059 | + } |
| 1060 | + } |
| 1061 | + ~FileHandler() { |
| 1062 | + if (file.is_open()) { |
| 1063 | + file.close(); |
| 1064 | + std::cout << "File Closed\n"; |
| 1065 | + } |
| 1066 | + } |
| 1067 | +}; |
793 | 1068 |
|
794 |
| -## 🔹 36. Does _.NET_ support _Multiple Inheritance_? |
| 1069 | +int main() { |
| 1070 | + FileHandler fh("sample.txt"); |
| 1071 | +} // Destructor called on fh, closes the file |
| 1072 | +``` |
| 1073 | +<br> |
795 | 1074 |
|
796 |
| -### Answer |
| 1075 | +## 15. Compare _inheritance_ vs. _mixin_ vs. _composition_. |
797 | 1076 |
|
798 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 1077 | +**Inheritance**, **mixins**, and **composition** are all techniques in object-oriented programming that deal with code reuse and the relationship between objects or classes. |
799 | 1078 |
|
800 |
| ---- |
| 1079 | +Let's look into the details and compare them: |
801 | 1080 |
|
802 |
| -## 🔹 37. Why use _Getters_ and _Setters_ for simple operations instead of _Public Fields_? |
| 1081 | +### Inheritance |
803 | 1082 |
|
804 |
| -### Answer |
| 1083 | +- **Definition**: A class (subclass) inherits properties and behaviors from another class (superclass). |
| 1084 | +- **Relationship**: "is-a" (e.g., a `Car` is a `Vehicle`). |
| 1085 | +- **Pros**: Direct way to reuse code; establishes intuitive relationships. |
| 1086 | +- **Cons**: Can lead to complex hierarchies; potential for over-generalization. |
805 | 1087 |
|
806 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 1088 | +### Mixin |
807 | 1089 |
|
808 |
| ---- |
| 1090 | +- **Definition**: A class that offers methods to other classes without being a standalone entity. Common in languages without multiple inheritance, such as Python. |
| 1091 | +- **Relationship**: "kind-of-a" or "can-do-this" (e.g., a `Helicopter` can fly like a `Bird`). |
| 1092 | +- **Pros**: Reuses code across classes; avoids deep inheritance issues. |
| 1093 | +- **Cons**: Method source can be unclear; potential name clashes. |
809 | 1094 |
|
810 |
| -## 🔹 38. What is _Circular Reference_? How to solve it? |
| 1095 | +### Composition |
811 | 1096 |
|
812 |
| -### Answer |
| 1097 | +- **Definition**: Building objects by combining simpler ones. Emphasizes a "has-a" relationship. |
| 1098 | +- **Relationship**: "has-a" (e.g., a `Car` has an `Engine`). |
| 1099 | +- **Pros**: Encourages modular design; components can be easily swapped. |
| 1100 | +- **Cons**: May need more design upfront; can require more boilerplate code. |
813 | 1101 |
|
814 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 1102 | +Many modern OOP design guidelines, like the **composition over inheritance principle**, suggest that unless there's a clear "is-a" relationship, it's often better to use **composition** as it's more flexible and leads to a more modular and maintainable design. |
| 1103 | +<br> |
815 | 1104 |
|
816 |
| ---- |
817 | 1105 |
|
818 |
| -## 🔹 39. Is it possible to declare a _Private Class_ within a _Namespace_? |
819 | 1106 |
|
820 |
| -### Answer |
| 1107 | +#### Explore all 52 answers here 👉 [Devinterview.io - OOP](https://devinterview.io/questions/web-and-mobile-development/oop-interview-questions) |
821 | 1108 |
|
822 |
| -👉🏼 Check out all 39 answers here: [Devinterview.io - OOP](https://devinterview.io/data/oop-interview-questions) |
| 1109 | +<br> |
823 | 1110 |
|
824 |
| ---- |
| 1111 | +<a href="https://devinterview.io/questions/web-and-mobile-development/"> |
| 1112 | +<img src="https://firebasestorage.googleapis.com/v0/b/dev-stack-app.appspot.com/o/github-blog-img%2Fweb-and-mobile-development-github-img.jpg?alt=media&token=1b5eeecc-c9fb-49f5-9e03-50cf2e309555" alt="web-and-mobile-development" width="100%"> |
| 1113 | +</a> |
| 1114 | +</p> |
825 | 1115 |
|
0 commit comments