|
1 | 1 | # Encapsulation
|
| 2 | + |
2 | 3 | Encapsulation is a process of wraping/combine/bind properties and methods (behavior) in a single unit or single container.
|
3 | 4 |
|
4 | 5 | 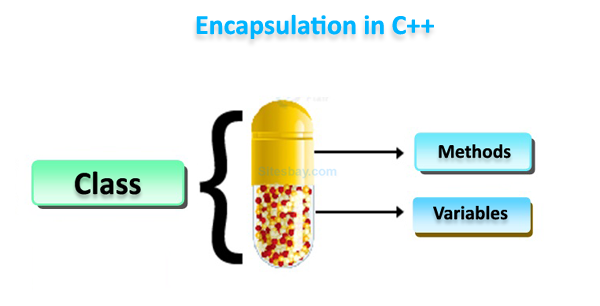
|
5 | 6 |
|
6 |
| -all state is private , cannot change the state. Only can change with public function. |
| 7 | +All state are private , cannot change state. Only can change with public function. |
7 | 8 |
|
8 |
| -~ Encapsulation or information hiding |
| 9 | +# key points of encapsulation. |
9 | 10 |
|
10 |
| -~ Hide internal states and values inside a class, (safe the modification of state) |
| 11 | +* Information hiding |
| 12 | +* Hide internal states and behavior from external world |
| 13 | +* Protect the modification of state |
11 | 14 |
|
12 |
| -```php |
13 |
| -class Cat |
14 |
| -{ |
15 |
| - private hungry; |
| 15 | +# Example of encapsulation (php) |
16 | 16 |
|
17 |
| - public function feedNow() |
18 |
| - { |
19 |
| - $this->hungry = 'feed'; |
| 17 | +```php |
| 18 | +class BankAccount { |
| 19 | + private $balance; |
| 20 | + |
| 21 | + public function __construct($initialBalance) { |
| 22 | + $this->balance = $initialBalance; |
| 23 | + } |
| 24 | + |
| 25 | + public function deposit($amount) { |
| 26 | + $this->balance += $amount; |
| 27 | + } |
| 28 | + |
| 29 | + public function withdraw($amount) { |
| 30 | + if ($this->balance >= $amount) { |
| 31 | + $this->balance -= $amount; |
| 32 | + } else { |
| 33 | + throw new Exception("Insufficient funds"); |
| 34 | + } |
| 35 | + } |
| 36 | + |
| 37 | + public function getBalance() { |
| 38 | + return $this->balance; |
20 | 39 | }
|
21 | 40 | }
|
| 41 | + |
| 42 | +$bankAccount = new BankAccount(1000); |
| 43 | + |
| 44 | +// Make a deposit |
| 45 | +$bankAccount->deposit(500); |
| 46 | + |
| 47 | +// Make a withdrawal |
| 48 | +try { |
| 49 | + $bankAccount->withdraw(2000); |
| 50 | +} catch (Exception $e) { |
| 51 | + echo $e->getMessage(); // Output: Insufficient funds |
| 52 | +} |
| 53 | + |
| 54 | +echo $bankAccount->getBalance(); // Output: 1500 |
22 | 55 | ```
|
23 | 56 |
|
24 |
| -# encapsulation vs abstraction |
| 57 | +# Difference between encapsulation and abstraction |
25 | 58 |
|
26 | 59 | * https://stackoverflow.com/questions/15176356/difference-between-encapsulation-and-abstraction
|
27 | 60 |
|
28 |
| -# resources |
| 61 | +# Resources |
29 | 62 |
|
30 | 63 | * https://www.sitesbay.com/cpp/cpp-encapsulation
|
31 | 64 | * https://www.freecodecamp.org/news/object-oriented-programming-concepts-21bb035f7260/
|
|
0 commit comments