diff --git a/.env b/.env new file mode 100644 index 0000000..639b439 --- /dev/null +++ b/.env @@ -0,0 +1,2 @@ +PORT=8080 +MONGO_PASSWORD=Haiderali_560 \ No newline at end of file diff --git a/README.md b/README.md deleted file mode 100644 index 0eb1f61..0000000 --- a/README.md +++ /dev/null @@ -1,384 +0,0 @@ - - -# FULL STACK COURSE 2023 - -## React JS MasterClass - -Hi, This is course page of **CoderDost Youtube Channel** React JS 2023 Course [Course Link ](https://youtu.be/6l8RWV8D-Yo), - -### How to use this code : - -#### You can **download code** in 2 ways : - -1. **Git Commands** - - - use `git clone <repository_url>` - - - checkout branch according to Chapter number `git checkout react-1` - - - run `npm install` inside the root directory before running the code - - - -2. If you are not comfortable with git, directly **download the branch as Zip**. - - - Choose branch related to the Chapter e.g. `react-1` - - - run `npm install` inside the root directory before running the code - - - - -# React JS Series - - - - -## Chapter 1 - Introduction to React & Setup - - -- **Assignment 1** : If we delete `node_modules`. How to run our app again successfully ? - - -- **Assignment 2** : How to remove double `console.logs` from React ? [ it is not needed in real life to remove them, its just an assignment problem ]. [ *Hint: Some special Component at top level is of App is causing it* ]. We explore more about - why this is needed in later videos. - -### Special Assignments ============== - -- **Assignment 3** : Create a Page with multiple React Apps. Both React Apps should be independent of each other. - -- **Assignment 4** : Try to build a react app using other toolchains like `Vite` - - - -### Related Videos : -1. De-structuring Assignment : [Long Video](https://youtu.be/sNhqFofQhFs) | [Object De-structure Short Video](https://youtube.com/shorts/H3MKXE69_c8) | [Array De-structure Short Video](https://youtube.com/shorts/ONGyMq49kZY) -2. Import/Export : [Long Video](https://youtu.be/7P5JUMc1cI4) | [Short Video](https://youtube.com/shorts/sUUOSWlwfM8) - - - -## Chapter 2 - Components - JSX and Props - - -- **Assignment 1** : Create a simple React app for **RESUME Builder**. It will be static website. You have to make components like **Resume** as top level and under it - **Skills**, **Education**, **Experience** etc as components. All resume data will be under 1 big JavaScript object like which you can us in components via props. You can fix the number of items in **Skills**, **Education**, **Experience** or any section. Example you can say that only 3 experience items is allowed. - - ``` - resume = { - experience : [ { year:2012, company:'xyz', role:'something' }], - education:[ ], - skills : [ 'react js', 'node js'] - ..... - ... - } - ``` - - > You can choose any simple HTML layout and convert it to React Components - - Example Link : [ Resume HTML ](https://codepen.io/emzarts/pen/OXzmym) - - ### Special Assignments ============== - -- **Assignment 2** : Create a Parent Component called **Border** which can provide some CSS border to any component nested into it. [Hint : You will need to use `children` props here - < Border> - < Component > - < Border /> - - -### Related Videos : -1. De-structuring Assignment : [Long Video](https://youtu.be/sNhqFofQhFs) | [Object De-structure Short Video](https://youtube.com/shorts/H3MKXE69_c8) | [Array De-structure Short Video](https://youtube.com/shorts/ONGyMq49kZY) -2. Import/Export : [Long Video](https://youtu.be/7P5JUMc1cI4) | [Short Video](https://youtube.com/shorts/sUUOSWlwfM8) -3. Spread Operator : [Long Video](https://youtu.be/X4Iv0TBHDQ4) | [Short Video](https://youtube.com/shorts/5dBZGyXutx8) - - -## Chapter 3 - Conditional Rendering & Lists - - -- **Assignment 1** : Make a simple component which can conditionally render a list with **number** or **alphabets** or ***bullets*** in HTML for number. e..g. use a prop like `layout` for this. Also use a prop `items` for array of items in list. - ``` -< List layout="numbered" items={items}/> -< List layout="alpha" items={items}/> -< List layout="bullet" items={items}/> - ``` - -- **Assignment 2** : This is continuation of previous assignment **RESUME Builder** - 1. In this part you have to make some conditional rendering. Suppose if any section doesn't exist you have to remove that section from **Resume**. Example if `skills` is empty array than don't display `skills` section in Resume. - 2. You have to use `map` in most places where there are arrays. Like **Skills**, **Education**, **Experience** if there are 3 entries, use `map` to display 3 experience items. You don't need fix number of items. Any array can have 1 to 10(or some Limit) any number of items. You can put some Limit, so that your layout is not affected. - 3. Conditionally put some styling to **Resume**. Like `light` theme or `dark` theme or any other way you can switch the CSS layouts. - - ``` - resume = { - experience : [ { year:2012, company:'xyz', role:'something' }], - education:[ ], - skills : [ 'react js', 'node js'] - ..... - ... - } - ``` - - > You can choose any simple HTML layout and convert it to React Components - - Example Link : [ Resume HTML ](https://codepen.io/emzarts/pen/OXzmym) - - - - -### Related Videos : -1. Ternary Operator : [Video](https://youtu.be/F_95TPUIA2c) -2. Logical AND (&&) : [Video](https://youtu.be/7dXLYWWTRXI) -3. Higher Order functions (map, filter, reduce) : [Video](https://youtu.be/oGpA98nNx6Y) -4. Import/Export : [Long Video](https://youtu.be/7P5JUMc1cI4) | [Short Video](https://youtube.com/shorts/sUUOSWlwfM8) - - -## Chapter 4 - Events && Event Bubbling - - -- **Assignment 1** : Make a simple page with 1 Image, 1button, 1 form Input text box and try to apply these events . - - - Image : **onMouseOver** : when you hover on image. Image should increase in size. Think of any way where you can do it. Also, try that when you "move out" the mouse, it should be back to normal size. - - Button : **onDoubleClick**: when you doubleClick a button. show some alert box. Also make a console.log for single click event. Is console.log printed twice on double click ? check this ? - - Input Textbox : **onFocus**, **onBlur** are 2 events which occur when you enter or exit an input text box by click of mouse etc. Use it to display some console.log, which print "focused on the textbox", "out of textbox". - - **onCopy, onCut, onPaste** are 3 events which you can use on any text value etc. try to use it on a paragraph and just alert the user that something is copied, cut or pasted. - - - -- **Assignment 2** : Make a form using `` < Form> `` tag and put an textbox and button inside this form. try to click the button after entering into textbox. Does form reloads ? Can you try to stop is using `e.preventDefault`. Try it. - -- **Assignment 3** : use an Input Textbox : after you enter some text. try to press **ENTER** button and show the an alert or console.log. You can capture the **onKeyPress** event, button how you will you make it work only for "Enter" ? It should not work on pressing of other keys. [*Hint: Explore the synthetic event object* ] - - -- **Assignment 4** : This is continuation of previous assignment **RESUME Builder**. - - Add a **print** button to print the current resume. You can use a DOM method ``window.print`` to print the PDF. - - -- **Assignment 5** : Can you try the challenge of passing the function in one Prop like `onPlay` and the message inside that function to be accessed from other prop `message` [ As shown in Chapter Video ] - -### Special Assignments ============== - -- **Assignment 6** : Using **event bubbling** concept print the name of Parents to Child of any clicked element. It should be order in "**GrandParent >Parent > Child**" this kind of order. Where "Child" represents the current clicked element. - -- **Assignment 7** : Make a custom event called **onClose**. this event should close the current browser tab. you can apply it to a button on click or anywhere. - -### Related Videos : - -1. HTML DOM Elements and manipulation [Video](https://youtu.be/kwfbaHNZ_aU) -2. HTML DOM Events and manipulation : [Video](https://youtu.be/5kpe_tg_Cis) - - - - -## Chapter 5 - State, useState Hooks - -- **Assignment 1** : Make a digital **CLOCK** Component using useEffect Hook. We need to only update the time Upto seconds in it. HH:MM:SS format can be used. Can you make it send a Console.log at end of every minute ? - -- **Assignment 2** : Implement a simple **TIMER** that displays the elapsed time since the start button was pressed, and it can be stopped and reset. Like a stopwatch. - - - - -## Chapter 6 - Form , Synthetic Event Object & Lifting State up - - -- **Assignment 1** : Create a Dropdown (< Select >) menu which is formed by a `nations` array. You can push to this array new items using a 2 **input textbox** (for country name and code) and **button**. -On selection of the any option from dropdown, its value should be displayed on console.log - ``` - const nations = [ -{ name: 'India', value: 'IN' }, -{ name: 'Pak', value: 'PK' }, -{ name: 'Bangladesh', value: 'BG' }, -] - ``` - - -- **Assignment 2** : **FILTERED LIST** : Make a **List** of something using an Array (a list can of cricket player /countries/ movie name etc). Now make this list it searchable, you have to put a **input textbox** at top of list. When you type in **textbox** it should only show you items matching from text typed. For example - -If you type only "in" it should show things like "India" / "China" as both have `in` in it. -- **Assignment 2.1** : **FILTERED LIST** : Make above **List** as separate components for List, Input form and pass the states from each other using concepts learnt till now. - - -- **Assignment 3** : - - This is continuation of previous assignment **RESUME Builder**. Now you have to make a separate component **ResumeEditor** which has a **FORM**. This form will have many **input boxes**. Each one related to one section. For example you can have one input box or **experience** section. Another input box for **skill** section and like this. Every input box should have an **Add** button in front of it. Once you press this add button that information is stored in the state , which you can update at top of the App level. Now this state should update the **Resume** Component and its child you have built. - - first component will be your **RESUME** document which is only for reading purpose. -- second component will be this **FORM** -- you have to manage the state in between -- only Add functionality is required in this assignment -- you can change input boxes according to your need depending on your format of Resume. You can have multiple textboxes also for same section. Like for date + experience item etc. - -- **Assignment 4** : Try this challenge : https://beta.reactjs.org/learn/state-a-components-memory#challenges - -### Related Videos : - -1. HTML Forms-1 [Video](https://youtu.be/DUJEpSkzrVA) -2. HTML Forms-2 : [Video](https://youtu.be/Nj6Omw6zOok) - - - -## MINI PROJECT - - -# Project 1 - TODO App - -Todo app can be used to maintain a list of your pending daily items. A Simple todo list must have these features - - -* You can add any new item to TODO list -* You can click on any item to mark it as done, if you have done that by mistake - you can click again to undo. -* You can delete any item (completed or pending) -* You get a total of completed items and overall items on the list. -* You can move list items "Up" or "Down" using buttons. - - -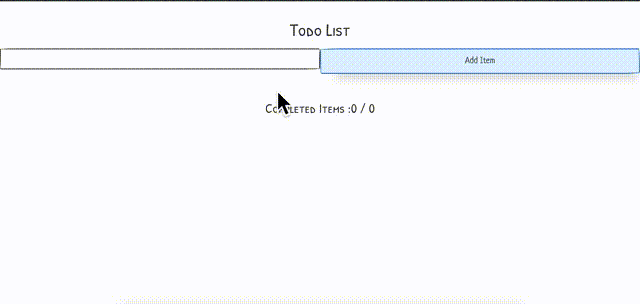 - - -## Additional Features - - **KEYBOARD BASED Features** : - -- use **ENTER** key on keyboard to add a new item. -- when you click on an item, it should be selected (you can change style to show it is selected) -- If you press the **DELETE** key on the keyboard after selecting the list item it should delete that list item. If you have not selected any item the last item should be deleted. -- You can select list item and press **UP** arrow key to Move It Up. And you can press the **DOWN** key to move it down. - - -**Other Features** : - -* **Pin element to Top of List** : On double click make element reach top of list. You can show a different color also to show that element is pinned. -* **Show the date & time** at which list item was added. -* **Order** by : Todo Item names, Date added, Completed. -* **Due date feature** : Add a due date of task of any todo item. You will need to add another input box for this at top. Whenever you are in 24 hour limit of due date - Task outline will be shown in ORANGE color. e.g if a task is due on 23 May - from 22nd May it should show in ORANGE outline color. If a due date is passed task should show RED Outline. -* Use some component like `https://github.com/react-component/progress` to show a **progress bar** at top of list. This progress bar will show how much of total percent of tasks are completed. -* **Delete item via swipe gesture** - like swipe to right on mobile phone. [*Hint: You have to find an event type for this* ] - -**Advanced Features** : - -* **Use localStorage** in browser using libraries like `https://github.com/localForage/localForage` to make your todo list permanent in browser. This will have your list stored in browser database and will not delete it on refresh or closing of browser. -[LocalStorage Video](https://youtu.be/OTkQVPVYW7w) -[LocalForage Video](https://youtu.be/Rqyu9qzydoc) - - **ANIMATION BASED Features [optional]** : - -* Enter Animation : Animate list item on adding. -* Exit Animation : Animate list item at removal. - - - - - - - -## Chapter 7 - More State & useEffect hooks - -- **Assignment 1** : The method shown in this video was just to introduce **useEffect** hook. However that was not the correct use of useEffect hook. Can you change the code to remove useEffect and still have the editVideo functionality. [ *Hint : use the concept that Component is rendered every time prop changes* ] - -- **Assignment 2** : This is continuation of previous assignment **RESUME Builder**. - - Add functionality to **delete** the items from resume. - - Add functionality to **update** the items from resume. - - you have to manage the state in between - - you can change input boxes according to your need depending on your format of Resume. You can have multiple textboxes also for same section. Like for date + experience item etc. - - Check the output can be printed perfectly in PDF. - - -## Chapter 8 - useReducer - -- **Assignment 1** : Try this challenge : https://beta.reactjs.org/learn/extracting-state-logic-into-a-reducer#challenges - -- **Assignment 2** : Convert your **RESUME BUILDER** Application from `useState` to `useReducer` by converting states logic to a common reducer. Your reducer can have as many switch cases as you want. You can also divide them based on sections. `ADD_SKILL`, `ADD_EXPERIENCE` etc. to make logic even simpler for developer. - -### Related Videos : - -1. REDUX - Understand it in Simple way [Video](https://youtu.be/WdEQNzUMP_M) - - - -## Chapter 9 - Context API & useContext - -- **Assignment 1** : Try this challenge : -https://beta.reactjs.org/learn/passing-data-deeply-with-context#challenges - -- **Assignment 2** : Add a Context to your **RESUME BUILDER** to change font-size, font-color and some other font-properties. Also add a form to changed these property at top of App. - -- **Assignment 3** : Add a Context to your **RESUME BUILDER** to change Dark Mode and Light Mode. You can also use a `React Switch` kind of library to make it more user friendly to switch. - - -## Chapter 10 - Context API with useReducer [Redux architecture] - -### Special Assignments - -- **Assignment 1** : Make a **useCounter** Hook: To keep track of a number that can be incremented or decremented. -``` const [count, increment, decrement] = useCounter(0);``` - - -## Chapter 11 - useRef - -- **Assignment 1** : Try this challenge: - -https://beta.reactjs.org/learn/referencing-values-with-refs#challenges - -- **Assignment 2** : Try this challenge: -https://beta.reactjs.org/learn/manipulating-the-dom-with-refs#challenges - -- **Assignment 3** : Make a **useWindowSize** Hook: which returns size of current browser window. -``` const [width, height] = useWindowSize();``` - - - -### Related Videos : - -1. Complete DOM Course playlist [Video](https://bit.ly/35nMKB7) - - - -## Chapter 12 - useEffect and API calling - -- **Assignment 1** : Try this challenge : - -https://beta.reactjs.org/learn/synchronizing-with-effects#challenges - -- **Assignment 2** : Try this challenge : - -https://beta.reactjs.org/learn/removing-effect-dependencies#challenges - -- **Assignment 3** : Try this challenge : - -https://beta.reactjs.org/learn/reusing-logic-with-custom-hooks#challenges - -- **Assignment 4** Use **JSON Placeholder API** (link given below). - - You have to create a button which can get some posts and show them in a List. - - You have to a **show comments** button on each list item. On click of show comments, Post's comments should be fetched below that list item. [ Comments are available for each post in API] - - When you click on a particular list item's show comments, it should expand and **show** comments, otherwise it should collapse and **hide** the comments. - - Try to optimize by : - - - Only getting comments of required Post items (not all at a time) - - Storing somehow the old comments of closed list items. So you will not fetch them again, when your show comments again. - -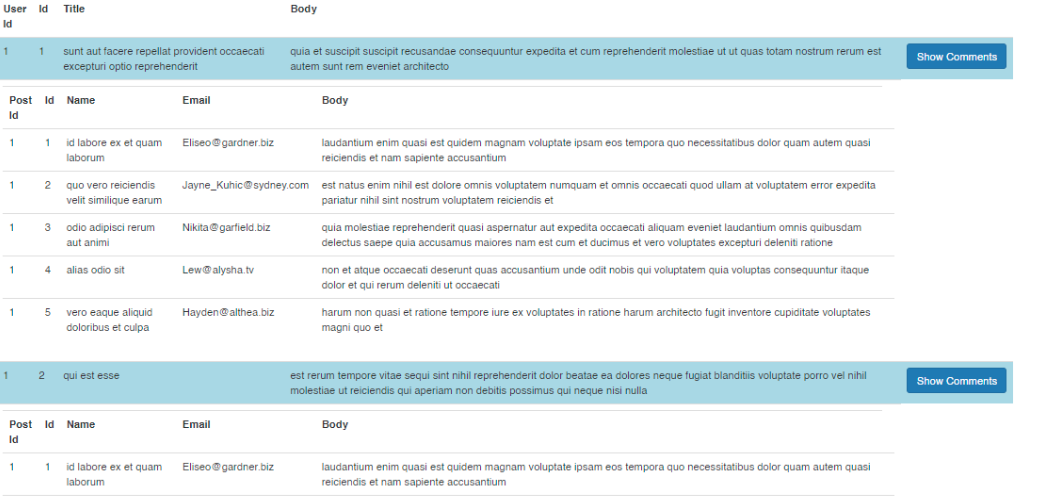 - -### Related Links : - -1. Mockaroo fake data APIs [Link](https://www.mockaroo.com/) -2. JSON placeholder API [Link](https://jsonplaceholder.typicode.com/) - - - -## Chapter 13 - Memoization - useMemo, useCallback, memo - -- **Assignment 1** : Implement a component that displays a list of items. The component should memoize the list of items to prevent unnecessary re-rendering. - -- **Assignment 2**: How to use memoization in the **JSON Placeholder API** assignment in previous problem. Can you try to **optimize** it using **useMemo/useCallback** ? - -- **Assignment 3**: **useMemo** and **useCallback** are same hook. useCallback is just a convenient hook way to write **useMemo** for functions. Prove this using **useMemo** in place of **useCallback** in any previous problem. *[ Hint : you will have to change the useMemo callback and return the function definition ]* - - - -## Chapter 14 - Advanced React - Part 1 - - -- **Assignment 1** : -Try to apply `useDeferredValue` and `useTransistion` hooks on API based components. Either make a new one or use any existing code you have from other assignments. You can use `Chrome Network throttling` from **Devtools** > **Performance** Tabs and use **Slow 3G** option to see the effects - - - -## Chapter 15 - Advanced React - Part 2 - -- **Assignment 1** : -Try to use `window.print` functionality as show in code without flushSync and see if really make a difference. Also, try the same on `alert` functionality, can it also work ? - diff --git a/controller/product.js b/controller/product.js new file mode 100644 index 0000000..50f9ed4 --- /dev/null +++ b/controller/product.js @@ -0,0 +1,69 @@ +const model = require('../model/product') + +console.log(model.Product); +const Product = model.Product; + +exports.createProduct = async (req, res) => { + try { + const product = new Product(req.body); + const dbResponse = await product.save(); + res.status(201).json(dbResponse); + } + catch (error) { + res.status(201).json(error); + } +} + +exports.getAllProducts = async (req, res) => { + try { + const dbResponse = await Product.find(); + res.status(201).json(dbResponse); + } + catch (error) { + res.status(201).json(error); + } +}; + +exports.getProduct = async (req, res) => { + try { + const id = req.params.id; + const dbResponse = await Product.findById(id); + res.status(201).json(dbResponse); + } + catch (error) { + res.status(201).json(error); + } +}; + +exports.replaceProduct = async (req, res) => { + try { + const id = req.params.id; + const dbResponse = await Product.findOneAndReplace({ _id: id }, req.body, { new: true }); + res.status(201).json(dbResponse); + } + catch (error) { + res.status(400).json(error); + } +}; + +exports.updateProduct = async (req, res) => { + try { + const id = req.params.id; + const dbResponse = await Product.findOneAndUpdate({ _id: id }, req.body, { new: true }); + res.status(201).json(dbResponse); + } + catch (error) { + res.status(400).json(error); + } +}; + +exports.deleteProduct = async (req, res) => { + try { + const id = req.params.id; + const dbResponse = await Product.findOneAndDelete({ _id: id }); + res.status(201).json(dbResponse); + } + catch (error) { + res.status(400).json(error); + } +}; diff --git a/controller/user.js b/controller/user.js new file mode 100644 index 0000000..bc0d2fe --- /dev/null +++ b/controller/user.js @@ -0,0 +1,40 @@ +const fs = require('fs'); +// const index = fs.readFileSync('index.html', 'utf-8'); +const data = JSON.parse(fs.readFileSync('data.json', 'utf-8')); +const users = data.users; + +exports.createUser = (req, res) => { + console.log(req.body); + users.push(req.body); + res.status(201).json(req.body); +}; + +exports.getAllUsers = (req, res) => { + res.json(users); +}; + +exports.getUser = (req, res) => { + const id = +req.params.id; + const user = users.find((p) => p.id === id); + res.json(user); +}; +exports.replaceUser = (req, res) => { + const id = +req.params.id; + const userIndex = users.findIndex((p) => p.id === id); + users.splice(userIndex, 1, { ...req.body, id: id }); + res.status(201).json(); +}; +exports.updateUser = (req, res) => { + const id = +req.params.id; + const userIndex = users.findIndex((p) => p.id === id); + const user = users[userIndex]; + users.splice(userIndex, 1, { ...user, ...req.body }); + res.status(201).json(); +}; +exports.deleteUser = (req, res) => { + const id = +req.params.id; + const userIndex = users.findIndex((p) => p.id === id); + const user = users[userIndex]; + users.splice(userIndex, 1); + res.status(201).json(user); +}; diff --git a/data.json b/data.json new file mode 100644 index 0000000..55cc92c --- /dev/null +++ b/data.json @@ -0,0 +1,2115 @@ +{ + "products": [ + { + "id": 1, + "title": "iPhone 9", + "description": "An apple mobile which is nothing like apple", + "price": 549, + "discountPercentage": 12.96, + "rating": 4.69, + "stock": 94, + "brand": "Apple", + "category": "smartphones", + "thumbnail": "https://i.dummyjson.com/data/products/1/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/1/1.jpg", + "https://i.dummyjson.com/data/products/1/2.jpg", + "https://i.dummyjson.com/data/products/1/3.jpg", + "https://i.dummyjson.com/data/products/1/4.jpg", + "https://i.dummyjson.com/data/products/1/thumbnail.jpg" + ] + }, + { + "id": 2, + "title": "iPhone X", + "description": "SIM-Free, Model A19211 6.5-inch Super Retina HD display with OLED technology A12 Bionic chip with ...", + "price": 899, + "discountPercentage": 17.94, + "rating": 4.44, + "stock": 34, + "brand": "Apple", + "category": "smartphones", + "thumbnail": "https://i.dummyjson.com/data/products/2/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/2/1.jpg", + "https://i.dummyjson.com/data/products/2/2.jpg", + "https://i.dummyjson.com/data/products/2/3.jpg", + "https://i.dummyjson.com/data/products/2/thumbnail.jpg" + ] + }, + { + "id": 3, + "title": "Samsung Universe 9", + "description": "Samsung's new variant which goes beyond Galaxy to the Universe", + "price": 1249, + "discountPercentage": 15.46, + "rating": 4.09, + "stock": 36, + "brand": "Samsung", + "category": "smartphones", + "thumbnail": "https://i.dummyjson.com/data/products/3/thumbnail.jpg", + "images": ["https://i.dummyjson.com/data/products/3/1.jpg"] + }, + { + "id": 4, + "title": "OPPOF19", + "description": "OPPO F19 is officially announced on April 2021.", + "price": 280, + "discountPercentage": 17.91, + "rating": 4.3, + "stock": 123, + "brand": "OPPO", + "category": "smartphones", + "thumbnail": "https://i.dummyjson.com/data/products/4/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/4/1.jpg", + "https://i.dummyjson.com/data/products/4/2.jpg", + "https://i.dummyjson.com/data/products/4/3.jpg", + "https://i.dummyjson.com/data/products/4/4.jpg", + "https://i.dummyjson.com/data/products/4/thumbnail.jpg" + ] + }, + { + "id": 5, + "title": "Huawei P30", + "description": "Huawei’s re-badged P30 Pro New Edition was officially unveiled yesterday in Germany and now the device has made its way to the UK.", + "price": 499, + "discountPercentage": 10.58, + "rating": 4.09, + "stock": 32, + "brand": "Huawei", + "category": "smartphones", + "thumbnail": "https://i.dummyjson.com/data/products/5/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/5/1.jpg", + "https://i.dummyjson.com/data/products/5/2.jpg", + "https://i.dummyjson.com/data/products/5/3.jpg" + ] + }, + { + "id": 6, + "title": "MacBook Pro", + "description": "MacBook Pro 2021 with mini-LED display may launch between September, November", + "price": 1749, + "discountPercentage": 11.02, + "rating": 4.57, + "stock": 83, + "brand": "Apple", + "category": "laptops", + "thumbnail": "https://i.dummyjson.com/data/products/6/thumbnail.png", + "images": [ + "https://i.dummyjson.com/data/products/6/1.png", + "https://i.dummyjson.com/data/products/6/2.jpg", + "https://i.dummyjson.com/data/products/6/3.png", + "https://i.dummyjson.com/data/products/6/4.jpg" + ] + }, + { + "id": 7, + "title": "Samsung Galaxy Book", + "description": "Samsung Galaxy Book S (2020) Laptop With Intel Lakefield Chip, 8GB of RAM Launched", + "price": 1499, + "discountPercentage": 4.15, + "rating": 4.25, + "stock": 50, + "brand": "Samsung", + "category": "laptops", + "thumbnail": "https://i.dummyjson.com/data/products/7/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/7/1.jpg", + "https://i.dummyjson.com/data/products/7/2.jpg", + "https://i.dummyjson.com/data/products/7/3.jpg", + "https://i.dummyjson.com/data/products/7/thumbnail.jpg" + ] + }, + { + "id": 8, + "title": "Microsoft Surface Laptop 4", + "description": "Style and speed. Stand out on HD video calls backed by Studio Mics. Capture ideas on the vibrant touchscreen.", + "price": 1499, + "discountPercentage": 10.23, + "rating": 4.43, + "stock": 68, + "brand": "Microsoft Surface", + "category": "laptops", + "thumbnail": "https://i.dummyjson.com/data/products/8/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/8/1.jpg", + "https://i.dummyjson.com/data/products/8/2.jpg", + "https://i.dummyjson.com/data/products/8/3.jpg", + "https://i.dummyjson.com/data/products/8/4.jpg", + "https://i.dummyjson.com/data/products/8/thumbnail.jpg" + ] + }, + { + "id": 9, + "title": "Infinix INBOOK", + "description": "Infinix Inbook X1 Ci3 10th 8GB 256GB 14 Win10 Grey – 1 Year Warranty", + "price": 1099, + "discountPercentage": 11.83, + "rating": 4.54, + "stock": 96, + "brand": "Infinix", + "category": "laptops", + "thumbnail": "https://i.dummyjson.com/data/products/9/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/9/1.jpg", + "https://i.dummyjson.com/data/products/9/2.png", + "https://i.dummyjson.com/data/products/9/3.png", + "https://i.dummyjson.com/data/products/9/4.jpg", + "https://i.dummyjson.com/data/products/9/thumbnail.jpg" + ] + }, + { + "id": 10, + "title": "HP Pavilion 15-DK1056WM", + "description": "HP Pavilion 15-DK1056WM Gaming Laptop 10th Gen Core i5, 8GB, 256GB SSD, GTX 1650 4GB, Windows 10", + "price": 1099, + "discountPercentage": 6.18, + "rating": 4.43, + "stock": 89, + "brand": "HP Pavilion", + "category": "laptops", + "thumbnail": "https://i.dummyjson.com/data/products/10/thumbnail.jpeg", + "images": [ + "https://i.dummyjson.com/data/products/10/1.jpg", + "https://i.dummyjson.com/data/products/10/2.jpg", + "https://i.dummyjson.com/data/products/10/3.jpg", + "https://i.dummyjson.com/data/products/10/thumbnail.jpeg" + ] + }, + { + "id": 11, + "title": "perfume Oil", + "description": "Mega Discount, Impression of Acqua Di Gio by GiorgioArmani concentrated attar perfume Oil", + "price": 13, + "discountPercentage": 8.4, + "rating": 4.26, + "stock": 65, + "brand": "Impression of Acqua Di Gio", + "category": "fragrances", + "thumbnail": "https://i.dummyjson.com/data/products/11/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/11/1.jpg", + "https://i.dummyjson.com/data/products/11/2.jpg", + "https://i.dummyjson.com/data/products/11/3.jpg", + "https://i.dummyjson.com/data/products/11/thumbnail.jpg" + ] + }, + { + "id": 12, + "title": "Brown Perfume", + "description": "Royal_Mirage Sport Brown Perfume for Men & Women - 120ml", + "price": 40, + "discountPercentage": 15.66, + "rating": 4, + "stock": 52, + "brand": "Royal_Mirage", + "category": "fragrances", + "thumbnail": "https://i.dummyjson.com/data/products/12/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/12/1.jpg", + "https://i.dummyjson.com/data/products/12/2.jpg", + "https://i.dummyjson.com/data/products/12/3.png", + "https://i.dummyjson.com/data/products/12/4.jpg", + "https://i.dummyjson.com/data/products/12/thumbnail.jpg" + ] + }, + { + "id": 13, + "title": "Fog Scent Xpressio Perfume", + "description": "Product details of Best Fog Scent Xpressio Perfume 100ml For Men cool long lasting perfumes for Men", + "price": 13, + "discountPercentage": 8.14, + "rating": 4.59, + "stock": 61, + "brand": "Fog Scent Xpressio", + "category": "fragrances", + "thumbnail": "https://i.dummyjson.com/data/products/13/thumbnail.webp", + "images": [ + "https://i.dummyjson.com/data/products/13/1.jpg", + "https://i.dummyjson.com/data/products/13/2.png", + "https://i.dummyjson.com/data/products/13/3.jpg", + "https://i.dummyjson.com/data/products/13/4.jpg", + "https://i.dummyjson.com/data/products/13/thumbnail.webp" + ] + }, + { + "id": 14, + "title": "Non-Alcoholic Concentrated Perfume Oil", + "description": "Original Al Munakh® by Mahal Al Musk | Our Impression of Climate | 6ml Non-Alcoholic Concentrated Perfume Oil", + "price": 120, + "discountPercentage": 15.6, + "rating": 4.21, + "stock": 114, + "brand": "Al Munakh", + "category": "fragrances", + "thumbnail": "https://i.dummyjson.com/data/products/14/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/14/1.jpg", + "https://i.dummyjson.com/data/products/14/2.jpg", + "https://i.dummyjson.com/data/products/14/3.jpg", + "https://i.dummyjson.com/data/products/14/thumbnail.jpg" + ] + }, + { + "id": 15, + "title": "Eau De Perfume Spray", + "description": "Genuine Al-Rehab spray perfume from UAE/Saudi Arabia/Yemen High Quality", + "price": 30, + "discountPercentage": 10.99, + "rating": 4.7, + "stock": 105, + "brand": "Lord - Al-Rehab", + "category": "fragrances", + "thumbnail": "https://i.dummyjson.com/data/products/15/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/15/1.jpg", + "https://i.dummyjson.com/data/products/15/2.jpg", + "https://i.dummyjson.com/data/products/15/3.jpg", + "https://i.dummyjson.com/data/products/15/4.jpg", + "https://i.dummyjson.com/data/products/15/thumbnail.jpg" + ] + }, + { + "id": 16, + "title": "Hyaluronic Acid Serum", + "description": "L'Oréal Paris introduces Hyaluron Expert Replumping Serum formulated with 1.5% Hyaluronic Acid", + "price": 19, + "discountPercentage": 13.31, + "rating": 4.83, + "stock": 110, + "brand": "L'Oreal Paris", + "category": "skincare", + "thumbnail": "https://i.dummyjson.com/data/products/16/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/16/1.png", + "https://i.dummyjson.com/data/products/16/2.webp", + "https://i.dummyjson.com/data/products/16/3.jpg", + "https://i.dummyjson.com/data/products/16/4.jpg", + "https://i.dummyjson.com/data/products/16/thumbnail.jpg" + ] + }, + { + "id": 17, + "title": "Tree Oil 30ml", + "description": "Tea tree oil contains a number of compounds, including terpinen-4-ol, that have been shown to kill certain bacteria,", + "price": 12, + "discountPercentage": 4.09, + "rating": 4.52, + "stock": 78, + "brand": "Hemani Tea", + "category": "skincare", + "thumbnail": "https://i.dummyjson.com/data/products/17/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/17/1.jpg", + "https://i.dummyjson.com/data/products/17/2.jpg", + "https://i.dummyjson.com/data/products/17/3.jpg", + "https://i.dummyjson.com/data/products/17/thumbnail.jpg" + ] + }, + { + "id": 18, + "title": "Oil Free Moisturizer 100ml", + "description": "Dermive Oil Free Moisturizer with SPF 20 is specifically formulated with ceramides, hyaluronic acid & sunscreen.", + "price": 40, + "discountPercentage": 13.1, + "rating": 4.56, + "stock": 88, + "brand": "Dermive", + "category": "skincare", + "thumbnail": "https://i.dummyjson.com/data/products/18/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/18/1.jpg", + "https://i.dummyjson.com/data/products/18/2.jpg", + "https://i.dummyjson.com/data/products/18/3.jpg", + "https://i.dummyjson.com/data/products/18/4.jpg", + "https://i.dummyjson.com/data/products/18/thumbnail.jpg" + ] + }, + { + "id": 19, + "title": "Skin Beauty Serum.", + "description": "Product name: rorec collagen hyaluronic acid white face serum riceNet weight: 15 m", + "price": 46, + "discountPercentage": 10.68, + "rating": 4.42, + "stock": 54, + "brand": "ROREC White Rice", + "category": "skincare", + "thumbnail": "https://i.dummyjson.com/data/products/19/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/19/1.jpg", + "https://i.dummyjson.com/data/products/19/2.jpg", + "https://i.dummyjson.com/data/products/19/3.png", + "https://i.dummyjson.com/data/products/19/thumbnail.jpg" + ] + }, + { + "id": 20, + "title": "Freckle Treatment Cream- 15gm", + "description": "Fair & Clear is Pakistan's only pure Freckle cream which helpsfade Freckles, Darkspots and pigments. Mercury level is 0%, so there are no side effects.", + "price": 70, + "discountPercentage": 16.99, + "rating": 4.06, + "stock": 140, + "brand": "Fair & Clear", + "category": "skincare", + "thumbnail": "https://i.dummyjson.com/data/products/20/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/20/1.jpg", + "https://i.dummyjson.com/data/products/20/2.jpg", + "https://i.dummyjson.com/data/products/20/3.jpg", + "https://i.dummyjson.com/data/products/20/4.jpg", + "https://i.dummyjson.com/data/products/20/thumbnail.jpg" + ] + }, + { + "id": 21, + "title": "- Daal Masoor 500 grams", + "description": "Fine quality Branded Product Keep in a cool and dry place", + "price": 20, + "discountPercentage": 4.81, + "rating": 4.44, + "stock": 133, + "brand": "Saaf & Khaas", + "category": "groceries", + "thumbnail": "https://i.dummyjson.com/data/products/21/thumbnail.png", + "images": [ + "https://i.dummyjson.com/data/products/21/1.png", + "https://i.dummyjson.com/data/products/21/2.jpg", + "https://i.dummyjson.com/data/products/21/3.jpg" + ] + }, + { + "id": 22, + "title": "Elbow Macaroni - 400 gm", + "description": "Product details of Bake Parlor Big Elbow Macaroni - 400 gm", + "price": 14, + "discountPercentage": 15.58, + "rating": 4.57, + "stock": 146, + "brand": "Bake Parlor Big", + "category": "groceries", + "thumbnail": "https://i.dummyjson.com/data/products/22/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/22/1.jpg", + "https://i.dummyjson.com/data/products/22/2.jpg", + "https://i.dummyjson.com/data/products/22/3.jpg" + ] + }, + { + "id": 23, + "title": "Orange Essence Food Flavou", + "description": "Specifications of Orange Essence Food Flavour For Cakes and Baking Food Item", + "price": 14, + "discountPercentage": 8.04, + "rating": 4.85, + "stock": 26, + "brand": "Baking Food Items", + "category": "groceries", + "thumbnail": "https://i.dummyjson.com/data/products/23/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/23/1.jpg", + "https://i.dummyjson.com/data/products/23/2.jpg", + "https://i.dummyjson.com/data/products/23/3.jpg", + "https://i.dummyjson.com/data/products/23/4.jpg", + "https://i.dummyjson.com/data/products/23/thumbnail.jpg" + ] + }, + { + "id": 24, + "title": "cereals muesli fruit nuts", + "description": "original fauji cereal muesli 250gm box pack original fauji cereals muesli fruit nuts flakes breakfast cereal break fast faujicereals cerels cerel foji fouji", + "price": 46, + "discountPercentage": 16.8, + "rating": 4.94, + "stock": 113, + "brand": "fauji", + "category": "groceries", + "thumbnail": "https://i.dummyjson.com/data/products/24/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/24/1.jpg", + "https://i.dummyjson.com/data/products/24/2.jpg", + "https://i.dummyjson.com/data/products/24/3.jpg", + "https://i.dummyjson.com/data/products/24/4.jpg", + "https://i.dummyjson.com/data/products/24/thumbnail.jpg" + ] + }, + { + "id": 25, + "title": "Gulab Powder 50 Gram", + "description": "Dry Rose Flower Powder Gulab Powder 50 Gram • Treats Wounds", + "price": 70, + "discountPercentage": 13.58, + "rating": 4.87, + "stock": 47, + "brand": "Dry Rose", + "category": "groceries", + "thumbnail": "https://i.dummyjson.com/data/products/25/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/25/1.png", + "https://i.dummyjson.com/data/products/25/2.jpg", + "https://i.dummyjson.com/data/products/25/3.png", + "https://i.dummyjson.com/data/products/25/4.jpg", + "https://i.dummyjson.com/data/products/25/thumbnail.jpg" + ] + }, + { + "id": 26, + "title": "Plant Hanger For Home", + "description": "Boho Decor Plant Hanger For Home Wall Decoration Macrame Wall Hanging Shelf", + "price": 41, + "discountPercentage": 17.86, + "rating": 4.08, + "stock": 131, + "brand": "Boho Decor", + "category": "home-decoration", + "thumbnail": "https://i.dummyjson.com/data/products/26/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/26/1.jpg", + "https://i.dummyjson.com/data/products/26/2.jpg", + "https://i.dummyjson.com/data/products/26/3.jpg", + "https://i.dummyjson.com/data/products/26/4.jpg", + "https://i.dummyjson.com/data/products/26/5.jpg", + "https://i.dummyjson.com/data/products/26/thumbnail.jpg" + ] + }, + { + "id": 27, + "title": "Flying Wooden Bird", + "description": "Package Include 6 Birds with Adhesive Tape Shape: 3D Shaped Wooden Birds Material: Wooden MDF, Laminated 3.5mm", + "price": 51, + "discountPercentage": 15.58, + "rating": 4.41, + "stock": 17, + "brand": "Flying Wooden", + "category": "home-decoration", + "thumbnail": "https://i.dummyjson.com/data/products/27/thumbnail.webp", + "images": [ + "https://i.dummyjson.com/data/products/27/1.jpg", + "https://i.dummyjson.com/data/products/27/2.jpg", + "https://i.dummyjson.com/data/products/27/3.jpg", + "https://i.dummyjson.com/data/products/27/4.jpg", + "https://i.dummyjson.com/data/products/27/thumbnail.webp" + ] + }, + { + "id": 28, + "title": "3D Embellishment Art Lamp", + "description": "3D led lamp sticker Wall sticker 3d wall art light on/off button cell operated (included)", + "price": 20, + "discountPercentage": 16.49, + "rating": 4.82, + "stock": 54, + "brand": "LED Lights", + "category": "home-decoration", + "thumbnail": "https://i.dummyjson.com/data/products/28/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/28/1.jpg", + "https://i.dummyjson.com/data/products/28/2.jpg", + "https://i.dummyjson.com/data/products/28/3.png", + "https://i.dummyjson.com/data/products/28/4.jpg", + "https://i.dummyjson.com/data/products/28/thumbnail.jpg" + ] + }, + { + "id": 29, + "title": "Handcraft Chinese style", + "description": "Handcraft Chinese style art luxury palace hotel villa mansion home decor ceramic vase with brass fruit plate", + "price": 60, + "discountPercentage": 15.34, + "rating": 4.44, + "stock": 7, + "brand": "luxury palace", + "category": "home-decoration", + "thumbnail": "https://i.dummyjson.com/data/products/29/thumbnail.webp", + "images": [ + "https://i.dummyjson.com/data/products/29/1.jpg", + "https://i.dummyjson.com/data/products/29/2.jpg", + "https://i.dummyjson.com/data/products/29/3.webp", + "https://i.dummyjson.com/data/products/29/4.webp", + "https://i.dummyjson.com/data/products/29/thumbnail.webp" + ] + }, + { + "id": 30, + "title": "Key Holder", + "description": "Attractive DesignMetallic materialFour key hooksReliable & DurablePremium Quality", + "price": 30, + "discountPercentage": 2.92, + "rating": 4.92, + "stock": 54, + "brand": "Golden", + "category": "home-decoration", + "thumbnail": "https://i.dummyjson.com/data/products/30/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/30/1.jpg", + "https://i.dummyjson.com/data/products/30/2.jpg", + "https://i.dummyjson.com/data/products/30/3.jpg", + "https://i.dummyjson.com/data/products/30/thumbnail.jpg" + ] + } + ], + "users": [ + { + "id": 1, + "firstName": "Terry", + "lastName": "Medhurst", + "maidenName": "Smitham", + "age": 50, + "gender": "male", + "email": "atuny0@sohu.com", + "phone": "+63 791 675 8914", + "username": "atuny0", + "password": "9uQFF1Lh", + "birthDate": "2000-12-25", + "image": "https://robohash.org/hicveldicta.png", + "bloodGroup": "A−", + "height": 189, + "weight": 75.4, + "eyeColor": "Green", + "hair": { "color": "Black", "type": "Strands" }, + "domain": "slashdot.org", + "ip": "117.29.86.254", + "address": { + "address": "1745 T Street Southeast", + "city": "Washington", + "coordinates": { "lat": 38.867033, "lng": -76.979235 }, + "postalCode": "20020", + "state": "DC" + }, + "macAddress": "13:69:BA:56:A3:74", + "university": "Capitol University", + "bank": { + "cardExpire": "06/22", + "cardNumber": "50380955204220685", + "cardType": "maestro", + "currency": "Peso", + "iban": "NO17 0695 2754 967" + }, + "company": { + "address": { + "address": "629 Debbie Drive", + "city": "Nashville", + "coordinates": { "lat": 36.208114, "lng": -86.58621199999999 }, + "postalCode": "37076", + "state": "TN" + }, + "department": "Marketing", + "name": "Blanda-O'Keefe", + "title": "Help Desk Operator" + }, + "ein": "20-9487066", + "ssn": "661-64-2976", + "userAgent": "Mozilla/5.0 (Windows NT 6.1) AppleWebKit/534.24 (KHTML, like Gecko) Chrome/12.0.702.0 Safari/534.24" + }, + { + "id": 2, + "firstName": "Sheldon", + "lastName": "Quigley", + "maidenName": "Cole", + "age": 28, + "gender": "male", + "email": "hbingley1@plala.or.jp", + "phone": "+7 813 117 7139", + "username": "hbingley1", + "password": "CQutx25i8r", + "birthDate": "2003-08-02", + "image": "https://robohash.org/doloremquesintcorrupti.png", + "bloodGroup": "O+", + "height": 187, + "weight": 74, + "eyeColor": "Brown", + "hair": { "color": "Blond", "type": "Curly" }, + "domain": "51.la", + "ip": "253.240.20.181", + "address": { + "address": "6007 Applegate Lane", + "city": "Louisville", + "coordinates": { "lat": 38.1343013, "lng": -85.6498512 }, + "postalCode": "40219", + "state": "KY" + }, + "macAddress": "13:F1:00:DA:A4:12", + "university": "Stavropol State Technical University", + "bank": { + "cardExpire": "10/23", + "cardNumber": "5355920631952404", + "cardType": "mastercard", + "currency": "Ruble", + "iban": "MD63 L6YC 8YH4 QVQB XHIK MTML" + }, + "company": { + "address": { + "address": "8821 West Myrtle Avenue", + "city": "Glendale", + "coordinates": { "lat": 33.5404296, "lng": -112.2488391 }, + "postalCode": "85305", + "state": "AZ" + }, + "department": "Services", + "name": "Aufderhar-Cronin", + "title": "Senior Cost Accountant" + }, + "ein": "52-5262907", + "ssn": "447-08-9217", + "userAgent": "Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/534.30 (KHTML, like Gecko) Ubuntu/11.04 Chromium/12.0.742.112 Chrome/12.0.742.112 Safari/534.30" + }, + { + "id": 3, + "firstName": "Terrill", + "lastName": "Hills", + "maidenName": "Hoeger", + "age": 38, + "gender": "male", + "email": "rshawe2@51.la", + "phone": "+63 739 292 7942", + "username": "rshawe2", + "password": "OWsTbMUgFc", + "birthDate": "1992-12-30", + "image": "https://robohash.org/consequunturautconsequatur.png", + "bloodGroup": "A−", + "height": 200, + "weight": 105.3, + "eyeColor": "Gray", + "hair": { "color": "Blond", "type": "Very curly" }, + "domain": "earthlink.net", + "ip": "205.226.160.3", + "address": { + "address": "560 Penstock Drive", + "city": "Grass Valley", + "coordinates": { "lat": 39.213076, "lng": -121.077583 }, + "postalCode": "95945", + "state": "CA" + }, + "macAddress": "F2:88:58:64:F7:76", + "university": "University of Cagayan Valley", + "bank": { + "cardExpire": "10/23", + "cardNumber": "3586082982526703", + "cardType": "jcb", + "currency": "Peso", + "iban": "AT24 1095 9625 1434 9703" + }, + "company": { + "address": { + "address": "18 Densmore Drive", + "city": "Essex", + "coordinates": { "lat": 44.492953, "lng": -73.101883 }, + "postalCode": "05452", + "state": "VT" + }, + "department": "Marketing", + "name": "Lindgren LLC", + "title": "Mechanical Systems Engineer" + }, + "ein": "48-3951994", + "ssn": "633-89-1926", + "userAgent": "Mozilla/5.0 (Windows NT 6.2; Win64; x64; rv:21.0.0) Gecko/20121011 Firefox/21.0.0" + }, + { + "id": 4, + "firstName": "Miles", + "lastName": "Cummerata", + "maidenName": "Maggio", + "age": 49, + "gender": "male", + "email": "yraigatt3@nature.com", + "phone": "+86 461 145 4186", + "username": "yraigatt3", + "password": "sRQxjPfdS", + "birthDate": "1969-01-16", + "image": "https://robohash.org/facilisdignissimosdolore.png", + "bloodGroup": "B+", + "height": 157, + "weight": 95.9, + "eyeColor": "Gray", + "hair": { "color": "Blond", "type": "Very curly" }, + "domain": "homestead.com", + "ip": "243.20.78.113", + "address": { + "address": "150 Carter Street", + "city": "Manchester", + "coordinates": { "lat": 41.76556000000001, "lng": -72.473091 }, + "postalCode": "06040", + "state": "CT" + }, + "macAddress": "03:45:58:59:5A:7B", + "university": "Shenyang Pharmaceutical University", + "bank": { + "cardExpire": "07/24", + "cardNumber": "3580047879369323", + "cardType": "jcb", + "currency": "Yuan Renminbi", + "iban": "KZ43 658B M6VS TZOU OXSO" + }, + "company": { + "address": { + "address": "210 Green Road", + "city": "Manchester", + "coordinates": { "lat": 41.7909099, "lng": -72.51195129999999 }, + "postalCode": "06042", + "state": "CT" + }, + "department": "Business Development", + "name": "Wolff and Sons", + "title": "Paralegal" + }, + "ein": "71-3644334", + "ssn": "487-28-6642", + "userAgent": "Mozilla/5.0 (Windows NT 6.2; WOW64) AppleWebKit/537.11 (KHTML, like Gecko) Chrome/23.0.1271.17 Safari/537.11" + }, + { + "id": 5, + "firstName": "Mavis", + "lastName": "Schultz", + "maidenName": "Yundt", + "age": 38, + "gender": "male", + "email": "kmeus4@upenn.edu", + "phone": "+372 285 771 1911", + "username": "kmeus4", + "password": "aUTdmmmbH", + "birthDate": "1968-11-03", + "image": "https://robohash.org/adverovelit.png", + "bloodGroup": "O+", + "height": 188, + "weight": 106.3, + "eyeColor": "Brown", + "hair": { "color": "Brown", "type": "Curly" }, + "domain": "columbia.edu", + "ip": "103.72.86.183", + "address": { + "address": "2721 Lindsay Avenue", + "city": "Louisville", + "coordinates": { "lat": 38.263793, "lng": -85.700243 }, + "postalCode": "40206", + "state": "KY" + }, + "macAddress": "F8:04:9E:ED:C0:68", + "university": "Estonian University of Life Sciences", + "bank": { + "cardExpire": "01/24", + "cardNumber": "4917245076693618", + "cardType": "visa-electron", + "currency": "Euro", + "iban": "IT41 T114 5127 716J RGYB ZRUX DSJ" + }, + "company": { + "address": { + "address": "8398 West Denton Lane", + "city": "Glendale", + "coordinates": { "lat": 33.515353, "lng": -112.240812 }, + "postalCode": "85305", + "state": "AZ" + }, + "department": "Support", + "name": "Adams Inc", + "title": "Web Developer I" + }, + "ein": "18-7178563", + "ssn": "667-98-5357", + "userAgent": "Mozilla/5.0 (Windows NT 6.0) AppleWebKit/535.1 (KHTML, like Gecko) Chrome/13.0.782.1 Safari/535.1" + }, + { + "id": 6, + "firstName": "Alison", + "lastName": "Reichert", + "maidenName": "Franecki", + "age": 21, + "gender": "female", + "email": "jtreleven5@nhs.uk", + "phone": "+351 527 735 3642", + "username": "jtreleven5", + "password": "zY1nE46Zm", + "birthDate": "1969-07-21", + "image": "https://robohash.org/laboriosamfacilisrem.png", + "bloodGroup": "A+", + "height": 149, + "weight": 105.7, + "eyeColor": "Amber", + "hair": { "color": "Blond", "type": "Straight" }, + "domain": "bandcamp.com", + "ip": "49.201.206.36", + "address": { + "address": "18 Densmore Drive", + "city": "Essex", + "coordinates": { "lat": 44.492953, "lng": -73.101883 }, + "postalCode": "05452", + "state": "VT" + }, + "macAddress": "6C:34:D0:4B:4E:81", + "university": "Universidade da Beira Interior", + "bank": { + "cardExpire": "03/22", + "cardNumber": "345675888286047", + "cardType": "americanexpress", + "currency": "Euro", + "iban": "LB69 1062 QCY5 XS5T VOKU KJFG XP4S" + }, + "company": { + "address": { + "address": "6231 North 67th Avenue", + "city": "Glendale", + "coordinates": { "lat": 33.5279666, "lng": -112.2022551 }, + "postalCode": "85301", + "state": "AZ" + }, + "department": "Accounting", + "name": "D'Amore and Sons", + "title": "Civil Engineer" + }, + "ein": "78-3192791", + "ssn": "158-68-0184", + "userAgent": "Mozilla/5.0 (Windows; U; Windows NT 6.0; nb-NO) AppleWebKit/533.18.1 (KHTML, like Gecko) Version/5.0.2 Safari/533.18.5" + }, + { + "id": 7, + "firstName": "Oleta", + "lastName": "Abbott", + "maidenName": "Wyman", + "age": 31, + "gender": "female", + "email": "dpettegre6@columbia.edu", + "phone": "+62 640 802 7111", + "username": "dpettegre6", + "password": "YVmhktgYVS", + "birthDate": "1982-02-21", + "image": "https://robohash.org/cupiditatererumquos.png", + "bloodGroup": "B−", + "height": 172, + "weight": 78.1, + "eyeColor": "Blue", + "hair": { "color": "Chestnut", "type": "Wavy" }, + "domain": "ovh.net", + "ip": "25.207.107.146", + "address": { + "address": "637 Britannia Drive", + "city": "Vallejo", + "coordinates": { "lat": 38.10476999999999, "lng": -122.193849 }, + "postalCode": "94591", + "state": "CA" + }, + "macAddress": "48:2D:A0:67:19:E0", + "university": "Institut Sains dan Teknologi Al Kamal", + "bank": { + "cardExpire": "10/23", + "cardNumber": "3589640949470047", + "cardType": "jcb", + "currency": "Rupiah", + "iban": "GI97 IKPF 9DUO X25M FG8D UXY" + }, + "company": { + "address": { + "address": "1407 Walden Court", + "city": "Crofton", + "coordinates": { "lat": 39.019306, "lng": -76.660653 }, + "postalCode": "21114", + "state": "MD" + }, + "department": "Product Management", + "name": "Schimmel, Wilderman and Orn", + "title": "Sales Associate" + }, + "ein": "29-1568401", + "ssn": "478-11-2206", + "userAgent": "Mozilla/5.0 (Windows; U; Windows NT 5.1; ru-RU) AppleWebKit/533.18.1 (KHTML, like Gecko) Version/5.0.2 Safari/533.18.5" + }, + { + "id": 8, + "firstName": "Ewell", + "lastName": "Mueller", + "maidenName": "Durgan", + "age": 29, + "gender": "male", + "email": "ggude7@chron.com", + "phone": "+86 946 297 2275", + "username": "ggude7", + "password": "MWwlaeWcOoF6", + "birthDate": "1964-08-24", + "image": "https://robohash.org/quiaharumsapiente.png", + "bloodGroup": "A−", + "height": 146, + "weight": 52.1, + "eyeColor": "Blue", + "hair": { "color": "Chestnut", "type": "Wavy" }, + "domain": "homestead.com", + "ip": "91.200.56.127", + "address": { + "address": "5601 West Crocus Drive", + "city": "Glendale", + "coordinates": { "lat": 33.6152469, "lng": -112.179737 }, + "postalCode": "85306", + "state": "AZ" + }, + "macAddress": "72:DA:1B:D7:30:E9", + "university": "Wenzhou Medical College", + "bank": { + "cardExpire": "09/23", + "cardNumber": "30549925358905", + "cardType": "diners-club-carte-blanche", + "currency": "Yuan Renminbi", + "iban": "CY02 9914 5346 0PMT G6XW TP0R AWRZ" + }, + "company": { + "address": { + "address": "81 Seaton Place Northwest", + "city": "Washington", + "coordinates": { "lat": 38.9149499, "lng": -77.01170259999999 }, + "postalCode": "20001", + "state": "DC" + }, + "department": "Services", + "name": "Corkery, Reichert and Hodkiewicz", + "title": "Clinical Specialist" + }, + "ein": "88-4396827", + "ssn": "238-41-5528", + "userAgent": "Mozilla/5.0 (X11; Linux amd64) AppleWebKit/534.36 (KHTML, like Gecko) Chrome/13.0.766.0 Safari/534.36" + }, + { + "id": 9, + "firstName": "Demetrius", + "lastName": "Corkery", + "maidenName": "Gleason", + "age": 22, + "gender": "male", + "email": "nloiterton8@aol.com", + "phone": "+86 356 590 9727", + "username": "nloiterton8", + "password": "HTQxxXV9Bq4", + "birthDate": "1971-03-11", + "image": "https://robohash.org/excepturiiuremolestiae.png", + "bloodGroup": "A+", + "height": 170, + "weight": 97.1, + "eyeColor": "Green", + "hair": { "color": "Brown", "type": "Strands" }, + "domain": "goodreads.com", + "ip": "78.170.185.120", + "address": { + "address": "5403 Illinois Avenue", + "city": "Nashville", + "coordinates": { "lat": 36.157077, "lng": -86.853827 }, + "postalCode": "37209", + "state": "TN" + }, + "macAddress": "98:EE:94:A2:91:C4", + "university": "Nanjing University of Economics", + "bank": { + "cardExpire": "02/24", + "cardNumber": "5372664789004621", + "cardType": "mastercard", + "currency": "Yuan Renminbi", + "iban": "BR68 9829 0581 3669 5088 5533 025N V" + }, + "company": { + "address": { + "address": "12245 West 71st Place", + "city": "Arvada", + "coordinates": { "lat": 39.8267078, "lng": -105.1366798 }, + "postalCode": "80004", + "state": "CO" + }, + "department": "Human Resources", + "name": "Gorczany Group", + "title": "Community Outreach Specialist" + }, + "ein": "14-1066382", + "ssn": "717-26-3759", + "userAgent": "Mozilla/5.0 (Macintosh; U; PPC Mac OS X 10_4_11; de) AppleWebKit/528.4+ (KHTML, like Gecko) Version/4.0dp1 Safari/526.11.2" + }, + { + "id": 10, + "firstName": "Eleanora", + "lastName": "Price", + "maidenName": "Cummings", + "age": 37, + "gender": "female", + "email": "umcgourty9@jalbum.net", + "phone": "+60 184 408 0824", + "username": "umcgourty9", + "password": "i0xzpX", + "birthDate": "1958-08-11", + "image": "https://robohash.org/aliquamcumqueiure.png", + "bloodGroup": "O+", + "height": 198, + "weight": 48, + "eyeColor": "Blue", + "hair": { "color": "Chestnut", "type": "Wavy" }, + "domain": "alibaba.com", + "ip": "73.15.179.178", + "address": { + "address": "8821 West Myrtle Avenue", + "city": "Glendale", + "coordinates": { "lat": 33.5404296, "lng": -112.2488391 }, + "postalCode": "85305", + "state": "AZ" + }, + "macAddress": "BC:A9:D8:98:CB:0B", + "university": "Melaka City Polytechnic", + "bank": { + "cardExpire": "01/24", + "cardNumber": "3557806620295254", + "cardType": "jcb", + "currency": "Ringgit", + "iban": "GT40 DWAD 9UHA VEOZ ZF4J 2Y0F OOFD" + }, + "company": { + "address": { + "address": "1649 Timberridge Court", + "city": "Fayetteville", + "coordinates": { "lat": 36.084563, "lng": -94.206082 }, + "postalCode": "72704", + "state": "AR" + }, + "department": "Marketing", + "name": "Bins Group", + "title": "Senior Sales Associate" + }, + "ein": "21-5278484", + "ssn": "544-66-0745", + "userAgent": "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_8_2) AppleWebKit/537.11 (KHTML, like Gecko) Chrome/23.0.1271.6 Safari/537.11" + }, + { + "id": 11, + "firstName": "Marcel", + "lastName": "Jones", + "maidenName": "Smith", + "age": 39, + "gender": "male", + "email": "acharlota@liveinternet.ru", + "phone": "+967 253 210 0344", + "username": "acharlota", + "password": "M9lbMdydMN", + "birthDate": "1961-09-12", + "image": "https://robohash.org/impeditautest.png", + "bloodGroup": "B−", + "height": 203, + "weight": 63.7, + "eyeColor": "Amber", + "hair": { "color": "Black", "type": "Straight" }, + "domain": "feedburner.com", + "ip": "137.235.164.173", + "address": { + "address": "2203 7th Street Road", + "city": "Louisville", + "coordinates": { "lat": 38.218107, "lng": -85.779006 }, + "postalCode": "40208", + "state": "KY" + }, + "macAddress": "59:E8:70:5A:E5:D6", + "university": "Hodeidah University", + "bank": { + "cardExpire": "05/24", + "cardNumber": "5893925889459720", + "cardType": "maestro", + "currency": "Rial", + "iban": "NL97 UWMY 2503 2999 43" + }, + "company": { + "address": { + "address": "308 Woodleaf Court", + "city": "Glen Burnie", + "coordinates": { "lat": 39.1425931, "lng": -76.6238441 }, + "postalCode": "21061", + "state": "MD" + }, + "department": "Business Development", + "name": "Kuhn-Harber", + "title": "Account Executive" + }, + "ein": "09-3791007", + "ssn": "342-54-8422", + "userAgent": "Mozilla/5.0 (Windows NT 5.2) AppleWebKit/535.1 (KHTML, like Gecko) Chrome/14.0.792.0 Safari/535.1" + }, + { + "id": 12, + "firstName": "Assunta", + "lastName": "Rath", + "maidenName": "Heller", + "age": 42, + "gender": "female", + "email": "rhallawellb@dropbox.com", + "phone": "+380 962 542 6549", + "username": "rhallawellb", + "password": "esTkitT1r", + "birthDate": "1990-12-14", + "image": "https://robohash.org/namquaerataut.png", + "bloodGroup": "O−", + "height": 168, + "weight": 96.8, + "eyeColor": "Gray", + "hair": { "color": "Black", "type": "Very curly" }, + "domain": "123-reg.co.uk", + "ip": "74.80.53.208", + "address": { + "address": "6463 Vrain Street", + "city": "Arvada", + "coordinates": { "lat": 39.814056, "lng": -105.046913 }, + "postalCode": "80003", + "state": "CO" + }, + "macAddress": "9B:DC:21:C2:30:A3", + "university": "Kiev Slavonic University", + "bank": { + "cardExpire": "09/22", + "cardNumber": "5602230671060360", + "cardType": "bankcard", + "currency": "Hryvnia", + "iban": "KW76 VNLA LX0Y DMDE PFS8 FVKP VMDF AV" + }, + "company": { + "address": { + "address": "388 East Main Street", + "coordinates": { "lat": 43.9727945, "lng": -73.1023187 }, + "postalCode": "05753", + "state": "VT" + }, + "department": "Product Management", + "name": "Goodwin-Skiles", + "title": "Developer II" + }, + "ein": "14-1242349", + "ssn": "116-51-6131", + "userAgent": "Mozilla/5.0 (X11; CrOS i686 4319.74.0) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/29.0.1547.57 Safari/537.36" + }, + { + "id": 13, + "firstName": "Trace", + "lastName": "Douglas", + "maidenName": "Lemke", + "age": 26, + "gender": "male", + "email": "lgribbinc@posterous.com", + "phone": "+1 609 937 3468", + "username": "lgribbinc", + "password": "ftGj8LZTtv9g", + "birthDate": "1967-07-23", + "image": "https://robohash.org/voluptatemsintnulla.png", + "bloodGroup": "O+", + "height": 181, + "weight": 56.5, + "eyeColor": "Amber", + "hair": { "color": "Auburn", "type": "Straight" }, + "domain": "histats.com", + "ip": "163.245.232.27", + "address": { + "address": "87 Horseshoe Drive", + "city": "West Windsor", + "coordinates": { "lat": 43.4731793, "lng": -72.4967532 }, + "postalCode": "05037", + "state": "VT" + }, + "macAddress": "B9:21:ED:9F:B8:9E", + "university": "Dallas Christian College", + "bank": { + "cardExpire": "01/23", + "cardNumber": "3556299106119514", + "cardType": "jcb", + "currency": "Dollar", + "iban": "AE47 4194 4544 3401 3419 286" + }, + "company": { + "address": { + "address": "310 Timrod Road", + "city": "Manchester", + "coordinates": { "lat": 41.756758, "lng": -72.493501 }, + "postalCode": "06040", + "state": "CT" + }, + "department": "Research and Development", + "name": "Casper Inc", + "title": "Sales Associate" + }, + "ein": "94-0648182", + "ssn": "217-05-3082", + "userAgent": "Mozilla/5.0 (Windows; U; Windows NT 5.1; ru-RU) AppleWebKit/533.19.4 (KHTML, like Gecko) Version/5.0.3 Safari/533.19.4" + }, + { + "id": 14, + "firstName": "Enoch", + "lastName": "Lynch", + "maidenName": "Heidenreich", + "age": 21, + "gender": "male", + "email": "mturleyd@tumblr.com", + "phone": "+94 912 100 5118", + "username": "mturleyd", + "password": "GyLnCB8gNIp", + "birthDate": "1979-08-25", + "image": "https://robohash.org/quisequienim.png", + "bloodGroup": "O+", + "height": 150, + "weight": 100.3, + "eyeColor": "Green", + "hair": { "color": "Auburn", "type": "Strands" }, + "domain": "icio.us", + "ip": "174.238.43.126", + "address": { + "address": "60 Desousa Drive", + "city": "Manchester", + "coordinates": { "lat": 41.7409259, "lng": -72.5619104 }, + "postalCode": "06040", + "state": "CT" + }, + "macAddress": "52:11:E1:31:35:C1", + "university": "University of Sri Jayawardenapura", + "bank": { + "cardExpire": "11/23", + "cardNumber": "5339467937996728", + "cardType": "mastercard", + "currency": "Rupee", + "iban": "SI28 0812 7967 0952 944" + }, + "company": { + "address": { + "address": "21950 Arnold Center Road", + "city": "Carson", + "coordinates": { "lat": 33.8272706, "lng": -118.2302826 }, + "postalCode": "90810", + "state": "CA" + }, + "department": "Sales", + "name": "Schoen Inc", + "title": "Professor" + }, + "ein": "61-8316825", + "ssn": "742-81-1714", + "userAgent": "Mozilla/5.0 (Windows; U; Windows NT 5.0; en-en) AppleWebKit/533.16 (KHTML, like Gecko) Version/4.1 Safari/533.16" + }, + { + "id": 15, + "firstName": "Jeanne", + "lastName": "Halvorson", + "maidenName": "Cummerata", + "age": 26, + "gender": "female", + "email": "kminchelle@qq.com", + "phone": "+86 581 108 7855", + "username": "kminchelle", + "password": "0lelplR", + "birthDate": "1996-02-02", + "image": "https://robohash.org/autquiaut.png", + "bloodGroup": "A+", + "height": 176, + "weight": 45.7, + "eyeColor": "Amber", + "hair": { "color": "Blond", "type": "Straight" }, + "domain": "google.co.uk", + "ip": "78.43.74.226", + "address": { + "address": "4 Old Colony Way", + "city": "Yarmouth", + "coordinates": { "lat": 41.697168, "lng": -70.189992 }, + "postalCode": "02664", + "state": "MA" + }, + "macAddress": "D9:DB:D9:5A:01:09", + "university": "Donghua University, Shanghai", + "bank": { + "cardExpire": "10/23", + "cardNumber": "3588859507772914", + "cardType": "jcb", + "currency": "Yuan Renminbi", + "iban": "FO12 1440 0396 8902 56" + }, + "company": { + "address": { + "address": "22572 Toreador Drive", + "city": "Salinas", + "coordinates": { "lat": 36.602449, "lng": -121.699071 }, + "postalCode": "93908", + "state": "CA" + }, + "department": "Marketing", + "name": "Hahn-MacGyver", + "title": "Software Test Engineer IV" + }, + "ein": "62-0561095", + "ssn": "855-43-8639", + "userAgent": "Mozilla/5.0 (X11; Linux i686) AppleWebKit/534.24 (KHTML, like Gecko) Chrome/11.0.696.14 Safari/534.24" + }, + { + "id": 16, + "firstName": "Trycia", + "lastName": "Fadel", + "maidenName": "Rosenbaum", + "age": 41, + "gender": "female", + "email": "dpierrof@vimeo.com", + "phone": "+420 833 708 0340", + "username": "dpierrof", + "password": "Vru55Y4tufI4", + "birthDate": "1963-07-03", + "image": "https://robohash.org/porronumquamid.png", + "bloodGroup": "B+", + "height": 166, + "weight": 87.2, + "eyeColor": "Gray", + "hair": { "color": "Black", "type": "Very curly" }, + "domain": "tamu.edu", + "ip": "82.170.69.15", + "address": { + "address": "314 South 17th Street", + "city": "Nashville", + "coordinates": { "lat": 36.1719075, "lng": -86.740228 }, + "postalCode": "37206", + "state": "TN" + }, + "macAddress": "3D:21:5B:9F:76:FF", + "university": "Technical University of Mining and Metallurgy Ostrava", + "bank": { + "cardExpire": "07/23", + "cardNumber": "6378941710246212", + "cardType": "instapayment", + "currency": "Koruna", + "iban": "CH94 4961 5QY1 VPV1 NGIP P" + }, + "company": { + "address": { + "address": "1407 Walden Court", + "city": "Crofton", + "coordinates": { "lat": 39.019306, "lng": -76.660653 }, + "postalCode": "21114", + "state": "MD" + }, + "department": "Research and Development", + "name": "Steuber, Considine and Padberg", + "title": "Geological Engineer" + }, + "ein": "75-1816504", + "ssn": "677-73-1525", + "userAgent": "Mozilla/5.0 (Windows NT 5.1) AppleWebKit/535.2 (KHTML, like Gecko) Chrome/15.0.872.0 Safari/535.2" + }, + { + "id": 17, + "firstName": "Bradford", + "lastName": "Prohaska", + "maidenName": "Bins", + "age": 43, + "gender": "male", + "email": "vcholdcroftg@ucoz.com", + "phone": "+420 874 628 3710", + "username": "vcholdcroftg", + "password": "mSPzYZfR", + "birthDate": "1975-10-20", + "image": "https://robohash.org/accusantiumvoluptateseos.png", + "bloodGroup": "O−", + "height": 199, + "weight": 94.3, + "eyeColor": "Brown", + "hair": { "color": "Black", "type": "Curly" }, + "domain": "wix.com", + "ip": "75.75.234.243", + "address": { + "address": "1649 Timberridge Court", + "city": "Fayetteville", + "coordinates": { "lat": 36.084563, "lng": -94.206082 }, + "postalCode": "72704", + "state": "AR" + }, + "macAddress": "47:FA:F7:94:7B:5D", + "university": "Technical University of Mining and Metallurgy Ostrava", + "bank": { + "cardExpire": "05/24", + "cardNumber": "3574627048005672", + "cardType": "jcb", + "currency": "Koruna", + "iban": "SI81 7221 0344 9088 864" + }, + "company": { + "address": { + "address": "20930 Todd Valley Road", + "city": "Foresthill", + "coordinates": { "lat": 38.989466, "lng": -120.883108 }, + "postalCode": "95631", + "state": "CA" + }, + "department": "Sales", + "name": "Bogisich and Sons", + "title": "Operator" + }, + "ein": "92-8837697", + "ssn": "795-36-7752", + "userAgent": "Mozilla/5.0 (Windows NT 5.2) AppleWebKit/535.1 (KHTML, like Gecko) Chrome/14.0.813.0 Safari/535.1" + }, + { + "id": 18, + "firstName": "Arely", + "lastName": "Skiles", + "maidenName": "Monahan", + "age": 42, + "gender": "male", + "email": "sberminghamh@chron.com", + "phone": "+55 886 766 8617", + "username": "sberminghamh", + "password": "cAjfb8vg", + "birthDate": "1958-02-05", + "image": "https://robohash.org/nihilharumqui.png", + "bloodGroup": "AB−", + "height": 192, + "weight": 97, + "eyeColor": "Amber", + "hair": { "color": "Brown", "type": "Straight" }, + "domain": "seesaa.net", + "ip": "29.82.54.30", + "address": { + "address": "5461 West Shades Valley Drive", + "city": "Montgomery", + "coordinates": { "lat": 32.296422, "lng": -86.34280299999999 }, + "postalCode": "36108", + "state": "AL" + }, + "macAddress": "61:0C:8F:92:48:D5", + "university": "Universidade Estadual do Ceará", + "bank": { + "cardExpire": "09/24", + "cardNumber": "3578078357052002", + "cardType": "jcb", + "currency": "Real", + "iban": "FR79 7925 2903 77HF 2ZY6 TU4M T84" + }, + "company": { + "address": { + "address": "3162 Martin Luther King Junior Boulevard", + "city": "Fayetteville", + "coordinates": { "lat": 36.05233310000001, "lng": -94.2056987 }, + "postalCode": "72704", + "state": "AR" + }, + "department": "Support", + "name": "Metz Group", + "title": "VP Accounting" + }, + "ein": "55-4062919", + "ssn": "551-74-1349", + "userAgent": "Mozilla/5.0 (Windows NT 5.1; rv:31.0) Gecko/20100101 Firefox/31.0" + }, + { + "id": 19, + "firstName": "Gust", + "lastName": "Purdy", + "maidenName": "Abshire", + "age": 46, + "gender": "male", + "email": "bleveragei@so-net.ne.jp", + "phone": "+86 886 889 0258", + "username": "bleveragei", + "password": "UZGAiqPqWQHQ", + "birthDate": "1989-10-15", + "image": "https://robohash.org/delenitipraesentiumvoluptatum.png", + "bloodGroup": "A−", + "height": 167, + "weight": 65.3, + "eyeColor": "Amber", + "hair": { "color": "Black", "type": "Straight" }, + "domain": "homestead.com", + "ip": "90.202.216.39", + "address": { + "address": "629 Debbie Drive", + "city": "Nashville", + "coordinates": { "lat": 36.208114, "lng": -86.58621199999999 }, + "postalCode": "37076", + "state": "TN" + }, + "macAddress": "22:98:8D:97:2D:AE", + "university": "Xinjiang University", + "bank": { + "cardExpire": "05/22", + "cardNumber": "5602214306858976", + "cardType": "bankcard", + "currency": "Yuan Renminbi", + "iban": "GB94 MOIU 1274 8449 9733 05" + }, + "company": { + "address": { + "address": "6463 Vrain Street", + "city": "Arvada", + "coordinates": { "lat": 39.814056, "lng": -105.046913 }, + "postalCode": "80003", + "state": "CO" + }, + "department": "Sales", + "name": "Bahringer, Auer and Wehner", + "title": "Financial Analyst" + }, + "ein": "53-7190545", + "ssn": "809-93-2422", + "userAgent": "Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/534.24 (KHTML, like Gecko) Chrome/11.0.696.3 Safari/534.24" + }, + { + "id": 20, + "firstName": "Lenna", + "lastName": "Renner", + "maidenName": "Schumm", + "age": 41, + "gender": "female", + "email": "aeatockj@psu.edu", + "phone": "+1 904 601 7177", + "username": "aeatockj", + "password": "szWAG6hc", + "birthDate": "1980-01-19", + "image": "https://robohash.org/ipsumutofficiis.png", + "bloodGroup": "O−", + "height": 175, + "weight": 68, + "eyeColor": "Green", + "hair": { "color": "Black", "type": "Strands" }, + "domain": "sourceforge.net", + "ip": "59.43.194.22", + "address": { + "address": "22572 Toreador Drive", + "city": "Salinas", + "coordinates": { "lat": 36.602449, "lng": -121.699071 }, + "postalCode": "93908", + "state": "CA" + }, + "macAddress": "ED:64:AE:91:49:C9", + "university": "Moraine Valley Community College", + "bank": { + "cardExpire": "07/22", + "cardNumber": "3565173055875732", + "cardType": "jcb", + "currency": "Dollar", + "iban": "GT39 KL9Z CZYV XF26 UPYW SFPT H74U" + }, + "company": { + "address": { + "address": "491 Arabian Way", + "city": "Grand Junction", + "coordinates": { "lat": 39.07548999999999, "lng": -108.474785 }, + "postalCode": "81504", + "state": "CO" + }, + "department": "Support", + "name": "Hoppe Group", + "title": "Geologist III" + }, + "ein": "88-6715551", + "ssn": "389-03-0381", + "userAgent": "Mozilla/5.0 (X11; Linux i686) AppleWebKit/534.24 (KHTML, like Gecko) Ubuntu/10.10 Chromium/12.0.702.0 Chrome/12.0.702.0 Safari/534.24" + }, + { + "id": 21, + "firstName": "Doyle", + "lastName": "Ernser", + "maidenName": "Feeney", + "age": 23, + "gender": "male", + "email": "ckensleyk@pen.io", + "phone": "+86 634 419 6839", + "username": "ckensleyk", + "password": "tq7kPXyf", + "birthDate": "1983-01-22", + "image": "https://robohash.org/providenttemporadelectus.png", + "bloodGroup": "A−", + "height": 173, + "weight": 69.9, + "eyeColor": "Brown", + "hair": { "color": "Black", "type": "Curly" }, + "domain": "free.fr", + "ip": "87.213.156.73", + "address": { + "address": "3034 Mica Street", + "city": "Fayetteville", + "coordinates": { "lat": 36.0807929, "lng": -94.2066449 }, + "postalCode": "72704", + "state": "AR" + }, + "macAddress": "E2:5A:A5:85:9B:6D", + "university": "Nanjing University of Traditional Chinese Medicine", + "bank": { + "cardExpire": "06/24", + "cardNumber": "30464640811198", + "cardType": "diners-club-carte-blanche", + "currency": "Yuan Renminbi", + "iban": "BE41 7150 0766 2980" + }, + "company": { + "address": { + "address": "5906 Milton Avenue", + "city": "Deale", + "coordinates": { "lat": 38.784451, "lng": -76.54125499999999 }, + "postalCode": "20751", + "state": "MD" + }, + "department": "Product Management", + "name": "Brekke Group", + "title": "Programmer Analyst I" + }, + "ein": "23-4116115", + "ssn": "562-46-9709", + "userAgent": "Mozilla/5.0 (Windows NT 6.2) AppleWebKit/536.3 (KHTML, like Gecko) Chrome/19.0.1061.0 Safari/536.3" + }, + { + "id": 22, + "firstName": "Tressa", + "lastName": "Weber", + "maidenName": "Williamson", + "age": 41, + "gender": "female", + "email": "froachel@howstuffworks.com", + "phone": "+34 517 104 6248", + "username": "froachel", + "password": "rfVSKImC", + "birthDate": "1987-11-11", + "image": "https://robohash.org/temporarecusandaeest.png", + "bloodGroup": "B−", + "height": 164, + "weight": 87.1, + "eyeColor": "Green", + "hair": { "color": "Black", "type": "Strands" }, + "domain": "indiatimes.com", + "ip": "71.57.235.192", + "address": { + "address": "3729 East Mission Boulevard", + "city": "Fayetteville", + "coordinates": { "lat": 36.0919353, "lng": -94.10654219999999 }, + "postalCode": "72703", + "state": "AR" + }, + "macAddress": "A4:8B:56:BC:ED:98", + "university": "Universitat Rámon Llull", + "bank": { + "cardExpire": "12/21", + "cardNumber": "342220243660686", + "cardType": "americanexpress", + "currency": "Euro", + "iban": "CY09 2675 2653 QNEJ JNSA 0E2V ONMM" + }, + "company": { + "address": { + "address": "8800 Cordell Circle", + "city": "Anchorage", + "coordinates": { "lat": 61.1409305, "lng": -149.9437822 }, + "postalCode": "99502", + "state": "AK" + }, + "department": "Research and Development", + "name": "Durgan Group", + "title": "VP Quality Control" + }, + "ein": "78-2846180", + "ssn": "155-87-0243", + "userAgent": "Mozilla/5.0 (Macintosh; U; PPC Mac OS X 10_4_11; de-de) AppleWebKit/533.16 (KHTML, like Gecko) Version/4.1 Safari/533.16" + }, + { + "id": 23, + "firstName": "Felicity", + "lastName": "O'Reilly", + "maidenName": "Rosenbaum", + "age": 46, + "gender": "female", + "email": "beykelhofm@wikispaces.com", + "phone": "+63 919 564 1690", + "username": "beykelhofm", + "password": "zQwaHTHbuZyr", + "birthDate": "1967-10-05", + "image": "https://robohash.org/odioquivero.png", + "bloodGroup": "O−", + "height": 151, + "weight": 96.7, + "eyeColor": "Brown", + "hair": { "color": "Brown", "type": "Curly" }, + "domain": "tamu.edu", + "ip": "141.14.53.176", + "address": { + "address": "5114 Greentree Drive", + "city": "Nashville", + "coordinates": { "lat": 36.0618539, "lng": -86.738508 }, + "postalCode": "37211", + "state": "TN" + }, + "macAddress": "4D:AB:8D:9A:E5:02", + "university": "University of lloilo", + "bank": { + "cardExpire": "06/22", + "cardNumber": "6333837222395642", + "cardType": "switch", + "currency": "Peso", + "iban": "FR40 3929 7903 26S5 QL9A HUSV Z09" + }, + "company": { + "address": { + "address": "1770 Colony Way", + "city": "Fayetteville", + "coordinates": { "lat": 36.0867, "lng": -94.229754 }, + "postalCode": "72704", + "state": "AR" + }, + "department": "Legal", + "name": "Romaguera, Williamson and Kessler", + "title": "Assistant Manager" + }, + "ein": "92-4814248", + "ssn": "441-72-1229", + "userAgent": "Mozilla/5.0 (Windows NT 5.1) AppleWebKit/535.2 (KHTML, like Gecko) Chrome/15.0.872.0 Safari/535.2" + }, + { + "id": 24, + "firstName": "Jocelyn", + "lastName": "Schuster", + "maidenName": "Dooley", + "age": 19, + "gender": "male", + "email": "brickeardn@fema.gov", + "phone": "+7 968 462 1292", + "username": "brickeardn", + "password": "bMQnPttV", + "birthDate": "1966-06-02", + "image": "https://robohash.org/odiomolestiaealias.png", + "bloodGroup": "O+", + "height": 166, + "weight": 93.3, + "eyeColor": "Brown", + "hair": { "color": "Brown", "type": "Curly" }, + "domain": "pen.io", + "ip": "116.92.198.102", + "address": { + "address": "3466 Southview Avenue", + "city": "Montgomery", + "coordinates": { "lat": 32.341227, "lng": -86.2846859 }, + "postalCode": "36111", + "state": "AL" + }, + "macAddress": "AF:AA:20:8E:CA:CD", + "university": "Bashkir State Medical University", + "bank": { + "cardExpire": "11/21", + "cardNumber": "5007666357943463", + "cardType": "mastercard", + "currency": "Ruble", + "iban": "NL22 YBPM 0101 6695 08" + }, + "company": { + "address": { + "address": "80 North East Street", + "city": "Holyoke", + "coordinates": { "lat": 42.2041219, "lng": -72.5977704 }, + "postalCode": "01040", + "state": "MA" + }, + "department": "Product Management", + "name": "Wintheiser-Boehm", + "title": "Research Nurse" + }, + "ein": "77-6259466", + "ssn": "291-72-5526", + "userAgent": "Mozilla/5.0 (Macintosh; U; Intel Mac OS X 10_6_7; ja-jp) AppleWebKit/533.20.25 (KHTML, like Gecko) Version/5.0.4 Safari/533.20.27" + }, + { + "id": 25, + "firstName": "Edwina", + "lastName": "Ernser", + "maidenName": "Kiehn", + "age": 21, + "gender": "female", + "email": "dfundello@amazon.co.jp", + "phone": "+86 376 986 8945", + "username": "dfundello", + "password": "k9zgV68UKw8m", + "birthDate": "2000-09-28", + "image": "https://robohash.org/doloremautdolores.png", + "bloodGroup": "O+", + "height": 180, + "weight": 102.1, + "eyeColor": "Blue", + "hair": { "color": "Brown", "type": "Wavy" }, + "domain": "apple.com", + "ip": "48.30.193.203", + "address": { + "address": "1513 Cathy Street", + "city": "Savannah", + "coordinates": { "lat": 32.067416, "lng": -81.125331 }, + "postalCode": "31415", + "state": "GA" + }, + "macAddress": "EC:59:D3:FC:65:92", + "university": "Wuhan University of Technology", + "bank": { + "cardExpire": "10/23", + "cardNumber": "3558628665594956", + "cardType": "jcb", + "currency": "Yuan Renminbi", + "iban": "RS85 6347 5884 2820 5764 23" + }, + "company": { + "address": { + "address": "125 John Street", + "city": "Santa Cruz", + "coordinates": { "lat": 36.950901, "lng": -122.046881 }, + "postalCode": "95060", + "state": "CA" + }, + "department": "Marketing", + "name": "Volkman Group", + "title": "Cost Accountant" + }, + "ein": "14-6307509", + "ssn": "266-43-5297", + "userAgent": "Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/536.3 (KHTML, like Gecko) Chrome/19.0.1063.0 Safari/536.3" + }, + { + "id": 26, + "firstName": "Griffin", + "lastName": "Braun", + "maidenName": "Deckow", + "age": 35, + "gender": "male", + "email": "lgronaverp@cornell.edu", + "phone": "+62 511 790 0161", + "username": "lgronaverp", + "password": "4a1dAKDv9KB9", + "birthDate": "1965-09-06", + "image": "https://robohash.org/laboriosammollitiaut.png", + "bloodGroup": "O−", + "height": 146, + "weight": 65.5, + "eyeColor": "Blue", + "hair": { "color": "Blond", "type": "Wavy" }, + "domain": "foxnews.com", + "ip": "93.246.47.59", + "address": { + "address": "600 West 19th Avenue", + "city": "Anchorage", + "coordinates": { "lat": 61.203115, "lng": -149.894107 }, + "postalCode": "99503", + "state": "AK" + }, + "macAddress": "34:06:26:95:37:D6", + "university": "Universitas Bojonegoro", + "bank": { + "cardExpire": "07/24", + "cardNumber": "3587188969123346", + "cardType": "jcb", + "currency": "Rupiah", + "iban": "AD24 9240 6903 OD2X OW1Y WD1K" + }, + "company": { + "address": { + "address": "1508 Massachusetts Avenue Southeast", + "city": "Washington", + "coordinates": { "lat": 38.887255, "lng": -76.98318499999999 }, + "postalCode": "20003", + "state": "DC" + }, + "department": "Engineering", + "name": "Boyle, Boyer and Lang", + "title": "Senior Cost Accountant" + }, + "ein": "38-0997138", + "ssn": "407-02-8915", + "userAgent": "Mozilla/5.0 (iPad; CPU OS 6_0 like Mac OS X) AppleWebKit/536.26 (KHTML, like Gecko) Version/6.0 Mobile/10A5355d Safari/8536.25" + }, + { + "id": 27, + "firstName": "Piper", + "lastName": "Schowalter", + "maidenName": "Wuckert", + "age": 47, + "gender": "female", + "email": "fokillq@amazon.co.jp", + "phone": "+60 785 960 7918", + "username": "fokillq", + "password": "xZnWSWnqH", + "birthDate": "1983-06-07", + "image": "https://robohash.org/nequeodiosapiente.png", + "bloodGroup": "A−", + "height": 197, + "weight": 71.5, + "eyeColor": "Brown", + "hair": { "color": "Black", "type": "Curly" }, + "domain": "toplist.cz", + "ip": "100.159.51.104", + "address": { + "address": "1208 Elkader Court North", + "city": "Nashville", + "coordinates": { "lat": 36.080049, "lng": -86.60116099999999 }, + "postalCode": "37013", + "state": "TN" + }, + "macAddress": "1F:42:5D:8C:66:3D", + "university": "Sultanah Bahiyah Polytechnic", + "bank": { + "cardExpire": "09/22", + "cardNumber": "6762169351744592", + "cardType": "maestro", + "currency": "Ringgit", + "iban": "BH05 STDW HECU HD4S L8U1 C6" + }, + "company": { + "address": { + "address": "600 West 19th Avenue", + "city": "Anchorage", + "coordinates": { "lat": 61.203115, "lng": -149.894107 }, + "postalCode": "99503", + "state": "AK" + }, + "department": "Human Resources", + "name": "O'Hara and Sons", + "title": "Sales Representative" + }, + "ein": "11-3129153", + "ssn": "408-90-5986", + "userAgent": "Mozilla/5.0 (Windows NT 5.1) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/41.0.2224.3 Safari/537.36" + }, + { + "id": 28, + "firstName": "Kody", + "lastName": "Terry", + "maidenName": "Larkin", + "age": 28, + "gender": "male", + "email": "xisherwoodr@ask.com", + "phone": "+81 859 545 8951", + "username": "xisherwoodr", + "password": "HLDqN5vCF", + "birthDate": "1979-01-09", + "image": "https://robohash.org/consequunturabnon.png", + "bloodGroup": "B−", + "height": 172, + "weight": 90.2, + "eyeColor": "Blue", + "hair": { "color": "Brown", "type": "Wavy" }, + "domain": "elpais.com", + "ip": "51.102.180.216", + "address": { + "address": "210 Green Road", + "city": "Manchester", + "coordinates": { "lat": 41.7909099, "lng": -72.51195129999999 }, + "postalCode": "06042", + "state": "CT" + }, + "macAddress": "B4:B6:17:3C:41:E5", + "university": "Science University of Tokyo", + "bank": { + "cardExpire": "05/23", + "cardNumber": "201443655632569", + "cardType": "diners-club-enroute", + "currency": "Yen", + "iban": "GT70 4NNE RDSR 0AJV 6AQI 4XH1 RWOC" + }, + "company": { + "address": { + "address": "109 Summit Street", + "city": "Burlington", + "coordinates": { "lat": 44.4729749, "lng": -73.2026566 }, + "postalCode": "05401", + "state": "VT" + }, + "department": "Support", + "name": "Leffler, Beatty and Kilback", + "title": "Recruiting Manager" + }, + "ein": "09-1129306", + "ssn": "389-74-9456", + "userAgent": "Mozilla/6.0 (Macintosh; I; Intel Mac OS X 11_7_9; de-LI; rv:1.9b4) Gecko/2012010317 Firefox/10.0a4" + }, + { + "id": 29, + "firstName": "Macy", + "lastName": "Greenfelder", + "maidenName": "Koepp", + "age": 45, + "gender": "female", + "email": "jissetts@hostgator.com", + "phone": "+81 915 649 2384", + "username": "jissetts", + "password": "ePawWgrnZR8L", + "birthDate": "1976-09-07", + "image": "https://robohash.org/amettemporeea.png", + "bloodGroup": "A−", + "height": 166, + "weight": 93.7, + "eyeColor": "Amber", + "hair": { "color": "Black", "type": "Straight" }, + "domain": "ibm.com", + "ip": "197.37.13.163", + "address": { + "address": "49548 Road 200", + "city": "O'Neals", + "coordinates": { "lat": 37.153463, "lng": -119.648192 }, + "postalCode": "93645", + "state": "CA" + }, + "macAddress": "D7:14:C5:45:69:C1", + "university": "Fuji Women's College", + "bank": { + "cardExpire": "04/24", + "cardNumber": "633413352570887921", + "cardType": "solo", + "currency": "Yen", + "iban": "IS23 8410 4605 1294 9479 5900 11" + }, + "company": { + "address": { + "address": "5403 Illinois Avenue", + "city": "Nashville", + "coordinates": { "lat": 36.157077, "lng": -86.853827 }, + "postalCode": "37209", + "state": "TN" + }, + "department": "Product Management", + "name": "Bruen and Sons", + "title": "Structural Analysis Engineer" + }, + "ein": "31-6688179", + "ssn": "391-33-1550", + "userAgent": "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_7_2) AppleWebKit/535.19 (KHTML, like Gecko) Chrome/18.0.1025.45 Safari/535.19" + }, + { + "id": 30, + "firstName": "Maurine", + "lastName": "Stracke", + "maidenName": "Abshire", + "age": 31, + "gender": "female", + "email": "kdulyt@umich.edu", + "phone": "+48 143 590 6847", + "username": "kdulyt", + "password": "5t6q4KC7O", + "birthDate": "1964-12-18", + "image": "https://robohash.org/perferendisideveniet.png", + "bloodGroup": "O−", + "height": 170, + "weight": 107.2, + "eyeColor": "Blue", + "hair": { "color": "Blond", "type": "Wavy" }, + "domain": "ow.ly", + "ip": "97.11.116.84", + "address": { + "address": "81 Seaton Place Northwest", + "city": "Washington", + "coordinates": { "lat": 38.9149499, "lng": -77.01170259999999 }, + "postalCode": "20001", + "state": "DC" + }, + "macAddress": "42:87:72:A1:4D:9A", + "university": "Poznan School of Banking", + "bank": { + "cardExpire": "02/24", + "cardNumber": "6331108070510590026", + "cardType": "switch", + "currency": "Zloty", + "iban": "MT70 MKRC 8244 59Z4 9UG1 1HY7 TKM6 1YX" + }, + "company": { + "address": { + "address": "816 West 19th Avenue", + "city": "Anchorage", + "coordinates": { "lat": 61.203221, "lng": -149.898655 }, + "postalCode": "99503", + "state": "AK" + }, + "department": "Support", + "name": "Balistreri-Kshlerin", + "title": "Quality Engineer" + }, + "ein": "51-7727524", + "ssn": "534-76-0952", + "userAgent": "Mozilla/5.0 (X11; Linux i686) AppleWebKit/535.11 (KHTML, like Gecko) Chrome/17.0.963.66 Safari/535.11" + } + ] +} diff --git a/index.html b/index.html new file mode 100644 index 0000000..1826a18 --- /dev/null +++ b/index.html @@ -0,0 +1,256 @@ +<link + rel="stylesheet" + href="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css" +/> +<link + rel="stylesheet" + href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" +/> +<style> + body { + background-color: #eee; + } + .container { + width: 900px; + } + + .card { + background-color: #fff; + border: none; + border-radius: 10px; + width: 250px; + box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19); + } + + .image-container { + position: relative; + } + + .thumbnail-image { + border-radius: 10px !important; + } + + .discount { + background-color: red; + padding-top: 1px; + padding-bottom: 1px; + padding-left: 4px; + padding-right: 4px; + font-size: 10px; + border-radius: 6px; + color: #fff; + } + + .wishlist { + height: 25px; + width: 25px; + background-color: #eee; + display: flex; + justify-content: center; + align-items: center; + border-radius: 50%; + box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19); + } + + .first { + position: absolute; + width: 100%; + padding: 9px; + } + + .dress-name { + font-size: 13px; + font-weight: bold; + width: 75%; + } + + .new-price { + font-size: 13px; + font-weight: bold; + color: red; + } + .old-price { + font-size: 8px; + font-weight: bold; + color: grey; + } + + .btn { + width: 14px; + height: 14px; + border-radius: 50%; + padding: 3px; + } + + .creme { + background-color: #fff; + border: 2px solid grey; + } + .creme:hover { + border: 3px solid grey; + } + + .creme:focus { + background-color: grey; + } + + .red { + background-color: #fff; + border: 2px solid red; + } + + .red:hover { + border: 3px solid red; + } + .red:focus { + background-color: red; + } + + .blue { + background-color: #fff; + border: 2px solid #40c4ff; + } + + .blue:hover { + border: 3px solid #40c4ff; + } + .blue:focus { + background-color: #40c4ff; + } + .darkblue { + background-color: #fff; + border: 2px solid #01579b; + } + .darkblue:hover { + border: 3px solid #01579b; + } + .darkblue:focus { + background-color: #01579b; + } + .yellow { + background-color: #fff; + border: 2px solid #ffca28; + } + .yellow:hover { + border-radius: 3px solid #ffca28; + } + .yellow:focus { + background-color: #ffca28; + } + + .item-size { + width: 15px; + height: 15px; + border-radius: 50%; + background: #fff; + border: 1px solid grey; + color: grey; + font-size: 10px; + text-align: center; + align-items: center; + display: flex; + justify-content: center; + } + + .rating-star { + font-size: 10px !important; + } + .rating-number { + font-size: 10px; + color: grey; + } + .buy { + font-size: 12px; + color: purple; + font-weight: 500; + } + + .voutchers { + background-color: #fff; + border: none; + border-radius: 10px; + width: 190px; + box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19); + overflow: hidden; + } + .voutcher-divider { + display: flex; + } + .voutcher-left { + width: 60%; + } + .voutcher-name { + color: grey; + font-size: 9px; + font-weight: 500; + } + .voutcher-code { + color: red; + font-size: 11px; + font-weight: bold; + } + .voutcher-right { + width: 40%; + background-color: purple; + color: #fff; + } + + .discount-percent { + font-size: 12px; + font-weight: bold; + position: relative; + top: 5px; + } + .off { + font-size: 14px; + position: relative; + bottom: 5px; + } +</style> + +<div class="container mt-5"> + <div class="row"> + <div class="col-md-3"> + <div class="card"> + <div class="image-container"> + <div class="first"> + <div class="d-flex justify-content-between align-items-center"> + <span class="discount">-25%</span> + <span class="wishlist"><i class="fa fa-heart-o"></i></span> + </div> + </div> + + <img + src="**url**" + class="img-fluid rounded thumbnail-image" + /> + </div> + + <div class="product-detail-container p-2"> + <div class="d-flex justify-content-between align-items-center"> + <h5 class="dress-name">**title**</h5> + + <div class="d-flex flex-column mb-2"> + <span class="new-price">₹ **price**</span> + <small class="old-price text-right">₹ 700</small> + </div> + </div> + + + + <div class="d-flex justify-content-between align-items-center pt-1"> + <div> + <i class="fa fa-star-o rating-star"></i> + <span class="rating-number">**rating**</span> + </div> + + <span class="buy">BUY +</span> + </div> + </div> + </div> + + + </div> + + </div> +</div> diff --git a/index.js b/index.js new file mode 100644 index 0000000..6bf5cbe --- /dev/null +++ b/index.js @@ -0,0 +1,28 @@ +require('dotenv').config(); +const express = require('express'); +const morgan = require('morgan'); +const mongoose = require('mongoose'); +const server = express(); +const productRouter = require('./routes/product') +const userRouter = require('./routes/user') + +main().catch((err) => console.log(err)) + +async function main() { + await mongoose.connect('mongodb+srv://haider:Haiderali_560@cluster0.otwulzw.mongodb.net/e-commerce?retryWrites=true&w=majority'); + console.log('connected') +} + + + +//bodyParser +server.use(express.json()); +server.use(morgan('default')); +server.use(express.static('public')); +server.use('/products', productRouter.router); +server.use('/users', userRouter.router); + +console.log(process.env.MONGO_PASSWORD) +server.listen(8080, () => { + console.log('server started'); +}); diff --git a/json-placeholder.png b/json-placeholder.png deleted file mode 100644 index 14a0b6e..0000000 Binary files a/json-placeholder.png and /dev/null differ diff --git a/model/product.js b/model/product.js new file mode 100644 index 0000000..ac8d418 --- /dev/null +++ b/model/product.js @@ -0,0 +1,17 @@ +const mongoose = require('mongoose'); + +const { Schema } = mongoose; + +const ProductSchema = new Schema({ + title: { type: String, required: true }, + description: String, + price: { type: Number, min: [0, 'Price should be greater than zero.'], required: true }, + discountPercentage: { type: Number, min: [0, 'wrong min value',], max: [50, 'wrong max value'] }, + rating: { type: Number, min: [0, 'wrong min rating'], max: [5, 'wrong max rating'] }, + brand: { type: String, required: true }, + category: { type: String, required: true }, + thumbnail: { type: String, required: true }, + images: [String] +}); + +exports.Product = mongoose.model('Product', ProductSchema) \ No newline at end of file diff --git a/package-lock.json b/package-lock.json new file mode 100644 index 0000000..4d39720 --- /dev/null +++ b/package-lock.json @@ -0,0 +1,921 @@ +{ + "name": "app", + "version": "1.0.0", + "lockfileVersion": 3, + "requires": true, + "packages": { + "": { + "name": "app", + "version": "1.0.0", + "license": "ISC", + "dependencies": { + "dotenv": "^16.3.1", + "express": "^4.18.2", + "index": "^0.4.0", + "mongoose": "^7.4.3", + "morgan": "^1.10.0" + } + }, + "node_modules/@types/node": { + "version": "20.5.1", + "resolved": "https://registry.npmjs.org/@types/node/-/node-20.5.1.tgz", + "integrity": "sha512-4tT2UrL5LBqDwoed9wZ6N3umC4Yhz3W3FloMmiiG4JwmUJWpie0c7lcnUNd4gtMKuDEO4wRVS8B6Xa0uMRsMKg==" + }, + "node_modules/@types/webidl-conversions": { + "version": "7.0.0", + "resolved": "https://registry.npmjs.org/@types/webidl-conversions/-/webidl-conversions-7.0.0.tgz", + "integrity": "sha512-xTE1E+YF4aWPJJeUzaZI5DRntlkY3+BCVJi0axFptnjGmAoWxkyREIh/XMrfxVLejwQxMCfDXdICo0VLxThrog==" + }, + "node_modules/@types/whatwg-url": { + "version": "8.2.2", + "resolved": "https://registry.npmjs.org/@types/whatwg-url/-/whatwg-url-8.2.2.tgz", + "integrity": "sha512-FtQu10RWgn3D9U4aazdwIE2yzphmTJREDqNdODHrbrZmmMqI0vMheC/6NE/J1Yveaj8H+ela+YwWTjq5PGmuhA==", + "dependencies": { + "@types/node": "*", + "@types/webidl-conversions": "*" + } + }, + "node_modules/accepts": { + "version": "1.3.8", + "resolved": "https://registry.npmjs.org/accepts/-/accepts-1.3.8.tgz", + "integrity": "sha512-PYAthTa2m2VKxuvSD3DPC/Gy+U+sOA1LAuT8mkmRuvw+NACSaeXEQ+NHcVF7rONl6qcaxV3Uuemwawk+7+SJLw==", + "dependencies": { + "mime-types": "~2.1.34", + "negotiator": "0.6.3" + }, + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/array-flatten": { + "version": "1.1.1", + "resolved": "https://registry.npmjs.org/array-flatten/-/array-flatten-1.1.1.tgz", + "integrity": "sha512-PCVAQswWemu6UdxsDFFX/+gVeYqKAod3D3UVm91jHwynguOwAvYPhx8nNlM++NqRcK6CxxpUafjmhIdKiHibqg==" + }, + "node_modules/basic-auth": { + "version": "2.0.1", + "resolved": "https://registry.npmjs.org/basic-auth/-/basic-auth-2.0.1.tgz", + "integrity": "sha512-NF+epuEdnUYVlGuhaxbbq+dvJttwLnGY+YixlXlME5KpQ5W3CnXA5cVTneY3SPbPDRkcjMbifrwmFYcClgOZeg==", + "dependencies": { + "safe-buffer": "5.1.2" + }, + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/basic-auth/node_modules/safe-buffer": { + "version": "5.1.2", + "resolved": "https://registry.npmjs.org/safe-buffer/-/safe-buffer-5.1.2.tgz", + "integrity": "sha512-Gd2UZBJDkXlY7GbJxfsE8/nvKkUEU1G38c1siN6QP6a9PT9MmHB8GnpscSmMJSoF8LOIrt8ud/wPtojys4G6+g==" + }, + "node_modules/body-parser": { + "version": "1.20.1", + "resolved": "https://registry.npmjs.org/body-parser/-/body-parser-1.20.1.tgz", + "integrity": "sha512-jWi7abTbYwajOytWCQc37VulmWiRae5RyTpaCyDcS5/lMdtwSz5lOpDE67srw/HYe35f1z3fDQw+3txg7gNtWw==", + "dependencies": { + "bytes": "3.1.2", + "content-type": "~1.0.4", + "debug": "2.6.9", + "depd": "2.0.0", + "destroy": "1.2.0", + "http-errors": "2.0.0", + "iconv-lite": "0.4.24", + "on-finished": "2.4.1", + "qs": "6.11.0", + "raw-body": "2.5.1", + "type-is": "~1.6.18", + "unpipe": "1.0.0" + }, + "engines": { + "node": ">= 0.8", + "npm": "1.2.8000 || >= 1.4.16" + } + }, + "node_modules/bson": { + "version": "5.4.0", + "resolved": "https://registry.npmjs.org/bson/-/bson-5.4.0.tgz", + "integrity": "sha512-WRZ5SQI5GfUuKnPTNmAYPiKIof3ORXAF4IRU5UcgmivNIon01rWQlw5RUH954dpu8yGL8T59YShVddIPaU/gFA==", + "engines": { + "node": ">=14.20.1" + } + }, + "node_modules/bytes": { + "version": "3.1.2", + "resolved": "https://registry.npmjs.org/bytes/-/bytes-3.1.2.tgz", + "integrity": "sha512-/Nf7TyzTx6S3yRJObOAV7956r8cr2+Oj8AC5dt8wSP3BQAoeX58NoHyCU8P8zGkNXStjTSi6fzO6F0pBdcYbEg==", + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/call-bind": { + "version": "1.0.2", + "resolved": "https://registry.npmjs.org/call-bind/-/call-bind-1.0.2.tgz", + "integrity": "sha512-7O+FbCihrB5WGbFYesctwmTKae6rOiIzmz1icreWJ+0aA7LJfuqhEso2T9ncpcFtzMQtzXf2QGGueWJGTYsqrA==", + "dependencies": { + "function-bind": "^1.1.1", + "get-intrinsic": "^1.0.2" + }, + "funding": { + "url": "https://github.com/sponsors/ljharb" + } + }, + "node_modules/content-disposition": { + "version": "0.5.4", + "resolved": "https://registry.npmjs.org/content-disposition/-/content-disposition-0.5.4.tgz", + "integrity": "sha512-FveZTNuGw04cxlAiWbzi6zTAL/lhehaWbTtgluJh4/E95DqMwTmha3KZN1aAWA8cFIhHzMZUvLevkw5Rqk+tSQ==", + "dependencies": { + "safe-buffer": "5.2.1" + }, + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/content-type": { + "version": "1.0.5", + "resolved": "https://registry.npmjs.org/content-type/-/content-type-1.0.5.tgz", + "integrity": "sha512-nTjqfcBFEipKdXCv4YDQWCfmcLZKm81ldF0pAopTvyrFGVbcR6P/VAAd5G7N+0tTr8QqiU0tFadD6FK4NtJwOA==", + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/cookie": { + "version": "0.5.0", + "resolved": "https://registry.npmjs.org/cookie/-/cookie-0.5.0.tgz", + "integrity": "sha512-YZ3GUyn/o8gfKJlnlX7g7xq4gyO6OSuhGPKaaGssGB2qgDUS0gPgtTvoyZLTt9Ab6dC4hfc9dV5arkvc/OCmrw==", + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/cookie-signature": { + "version": "1.0.6", + "resolved": "https://registry.npmjs.org/cookie-signature/-/cookie-signature-1.0.6.tgz", + "integrity": "sha512-QADzlaHc8icV8I7vbaJXJwod9HWYp8uCqf1xa4OfNu1T7JVxQIrUgOWtHdNDtPiywmFbiS12VjotIXLrKM3orQ==" + }, + "node_modules/debug": { + "version": "2.6.9", + "resolved": "https://registry.npmjs.org/debug/-/debug-2.6.9.tgz", + "integrity": "sha512-bC7ElrdJaJnPbAP+1EotYvqZsb3ecl5wi6Bfi6BJTUcNowp6cvspg0jXznRTKDjm/E7AdgFBVeAPVMNcKGsHMA==", + "dependencies": { + "ms": "2.0.0" + } + }, + "node_modules/depd": { + "version": "2.0.0", + "resolved": "https://registry.npmjs.org/depd/-/depd-2.0.0.tgz", + "integrity": "sha512-g7nH6P6dyDioJogAAGprGpCtVImJhpPk/roCzdb3fIh61/s/nPsfR6onyMwkCAR/OlC3yBC0lESvUoQEAssIrw==", + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/destroy": { + "version": "1.2.0", + "resolved": "https://registry.npmjs.org/destroy/-/destroy-1.2.0.tgz", + "integrity": "sha512-2sJGJTaXIIaR1w4iJSNoN0hnMY7Gpc/n8D4qSCJw8QqFWXf7cuAgnEHxBpweaVcPevC2l3KpjYCx3NypQQgaJg==", + "engines": { + "node": ">= 0.8", + "npm": "1.2.8000 || >= 1.4.16" + } + }, + "node_modules/dotenv": { + "version": "16.3.1", + "resolved": "https://registry.npmjs.org/dotenv/-/dotenv-16.3.1.tgz", + "integrity": "sha512-IPzF4w4/Rd94bA9imS68tZBaYyBWSCE47V1RGuMrB94iyTOIEwRmVL2x/4An+6mETpLrKJ5hQkB8W4kFAadeIQ==", + "engines": { + "node": ">=12" + }, + "funding": { + "url": "https://github.com/motdotla/dotenv?sponsor=1" + } + }, + "node_modules/ee-first": { + "version": "1.1.1", + "resolved": "https://registry.npmjs.org/ee-first/-/ee-first-1.1.1.tgz", + "integrity": "sha512-WMwm9LhRUo+WUaRN+vRuETqG89IgZphVSNkdFgeb6sS/E4OrDIN7t48CAewSHXc6C8lefD8KKfr5vY61brQlow==" + }, + "node_modules/encodeurl": { + "version": "1.0.2", + "resolved": "https://registry.npmjs.org/encodeurl/-/encodeurl-1.0.2.tgz", + "integrity": "sha512-TPJXq8JqFaVYm2CWmPvnP2Iyo4ZSM7/QKcSmuMLDObfpH5fi7RUGmd/rTDf+rut/saiDiQEeVTNgAmJEdAOx0w==", + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/escape-html": { + "version": "1.0.3", + "resolved": "https://registry.npmjs.org/escape-html/-/escape-html-1.0.3.tgz", + "integrity": "sha512-NiSupZ4OeuGwr68lGIeym/ksIZMJodUGOSCZ/FSnTxcrekbvqrgdUxlJOMpijaKZVjAJrWrGs/6Jy8OMuyj9ow==" + }, + "node_modules/etag": { + "version": "1.8.1", + "resolved": "https://registry.npmjs.org/etag/-/etag-1.8.1.tgz", + "integrity": "sha512-aIL5Fx7mawVa300al2BnEE4iNvo1qETxLrPI/o05L7z6go7fCw1J6EQmbK4FmJ2AS7kgVF/KEZWufBfdClMcPg==", + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/express": { + "version": "4.18.2", + "resolved": "https://registry.npmjs.org/express/-/express-4.18.2.tgz", + "integrity": "sha512-5/PsL6iGPdfQ/lKM1UuielYgv3BUoJfz1aUwU9vHZ+J7gyvwdQXFEBIEIaxeGf0GIcreATNyBExtalisDbuMqQ==", + "dependencies": { + "accepts": "~1.3.8", + "array-flatten": "1.1.1", + "body-parser": "1.20.1", + "content-disposition": "0.5.4", + "content-type": "~1.0.4", + "cookie": "0.5.0", + "cookie-signature": "1.0.6", + "debug": "2.6.9", + "depd": "2.0.0", + "encodeurl": "~1.0.2", + "escape-html": "~1.0.3", + "etag": "~1.8.1", + "finalhandler": "1.2.0", + "fresh": "0.5.2", + "http-errors": "2.0.0", + "merge-descriptors": "1.0.1", + "methods": "~1.1.2", + "on-finished": "2.4.1", + "parseurl": "~1.3.3", + "path-to-regexp": "0.1.7", + "proxy-addr": "~2.0.7", + "qs": "6.11.0", + "range-parser": "~1.2.1", + "safe-buffer": "5.2.1", + "send": "0.18.0", + "serve-static": "1.15.0", + "setprototypeof": "1.2.0", + "statuses": "2.0.1", + "type-is": "~1.6.18", + "utils-merge": "1.0.1", + "vary": "~1.1.2" + }, + "engines": { + "node": ">= 0.10.0" + } + }, + "node_modules/finalhandler": { + "version": "1.2.0", + "resolved": "https://registry.npmjs.org/finalhandler/-/finalhandler-1.2.0.tgz", + "integrity": "sha512-5uXcUVftlQMFnWC9qu/svkWv3GTd2PfUhK/3PLkYNAe7FbqJMt3515HaxE6eRL74GdsriiwujiawdaB1BpEISg==", + "dependencies": { + "debug": "2.6.9", + "encodeurl": "~1.0.2", + "escape-html": "~1.0.3", + "on-finished": "2.4.1", + "parseurl": "~1.3.3", + "statuses": "2.0.1", + "unpipe": "~1.0.0" + }, + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/forwarded": { + "version": "0.2.0", + "resolved": "https://registry.npmjs.org/forwarded/-/forwarded-0.2.0.tgz", + "integrity": "sha512-buRG0fpBtRHSTCOASe6hD258tEubFoRLb4ZNA6NxMVHNw2gOcwHo9wyablzMzOA5z9xA9L1KNjk/Nt6MT9aYow==", + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/fresh": { + "version": "0.5.2", + "resolved": "https://registry.npmjs.org/fresh/-/fresh-0.5.2.tgz", + "integrity": "sha512-zJ2mQYM18rEFOudeV4GShTGIQ7RbzA7ozbU9I/XBpm7kqgMywgmylMwXHxZJmkVoYkna9d2pVXVXPdYTP9ej8Q==", + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/function-bind": { + "version": "1.1.1", + "resolved": "https://registry.npmjs.org/function-bind/-/function-bind-1.1.1.tgz", + "integrity": "sha512-yIovAzMX49sF8Yl58fSCWJ5svSLuaibPxXQJFLmBObTuCr0Mf1KiPopGM9NiFjiYBCbfaa2Fh6breQ6ANVTI0A==" + }, + "node_modules/get-intrinsic": { + "version": "1.2.0", + "resolved": "https://registry.npmjs.org/get-intrinsic/-/get-intrinsic-1.2.0.tgz", + "integrity": "sha512-L049y6nFOuom5wGyRc3/gdTLO94dySVKRACj1RmJZBQXlbTMhtNIgkWkUHq+jYmZvKf14EW1EoJnnjbmoHij0Q==", + "dependencies": { + "function-bind": "^1.1.1", + "has": "^1.0.3", + "has-symbols": "^1.0.3" + }, + "funding": { + "url": "https://github.com/sponsors/ljharb" + } + }, + "node_modules/has": { + "version": "1.0.3", + "resolved": "https://registry.npmjs.org/has/-/has-1.0.3.tgz", + "integrity": "sha512-f2dvO0VU6Oej7RkWJGrehjbzMAjFp5/VKPp5tTpWIV4JHHZK1/BxbFRtf/siA2SWTe09caDmVtYYzWEIbBS4zw==", + "dependencies": { + "function-bind": "^1.1.1" + }, + "engines": { + "node": ">= 0.4.0" + } + }, + "node_modules/has-symbols": { + "version": "1.0.3", + "resolved": "https://registry.npmjs.org/has-symbols/-/has-symbols-1.0.3.tgz", + "integrity": "sha512-l3LCuF6MgDNwTDKkdYGEihYjt5pRPbEg46rtlmnSPlUbgmB8LOIrKJbYYFBSbnPaJexMKtiPO8hmeRjRz2Td+A==", + "engines": { + "node": ">= 0.4" + }, + "funding": { + "url": "https://github.com/sponsors/ljharb" + } + }, + "node_modules/http-errors": { + "version": "2.0.0", + "resolved": "https://registry.npmjs.org/http-errors/-/http-errors-2.0.0.tgz", + "integrity": "sha512-FtwrG/euBzaEjYeRqOgly7G0qviiXoJWnvEH2Z1plBdXgbyjv34pHTSb9zoeHMyDy33+DWy5Wt9Wo+TURtOYSQ==", + "dependencies": { + "depd": "2.0.0", + "inherits": "2.0.4", + "setprototypeof": "1.2.0", + "statuses": "2.0.1", + "toidentifier": "1.0.1" + }, + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/iconv-lite": { + "version": "0.4.24", + "resolved": "https://registry.npmjs.org/iconv-lite/-/iconv-lite-0.4.24.tgz", + "integrity": "sha512-v3MXnZAcvnywkTUEZomIActle7RXXeedOR31wwl7VlyoXO4Qi9arvSenNQWne1TcRwhCL1HwLI21bEqdpj8/rA==", + "dependencies": { + "safer-buffer": ">= 2.1.2 < 3" + }, + "engines": { + "node": ">=0.10.0" + } + }, + "node_modules/index": { + "version": "0.4.0", + "resolved": "https://registry.npmjs.org/index/-/index-0.4.0.tgz", + "integrity": "sha512-48AIN2XNXFXDb7muQD+4RZvXSy862VkXu5vkLh2zjikLOBWjI68OBUaRxt8ng1tolPlK9vrwMP/5th0VY53oNg==", + "dependencies": { + "step": ">= 0.0.4" + }, + "engines": { + "node": "*" + } + }, + "node_modules/inherits": { + "version": "2.0.4", + "resolved": "https://registry.npmjs.org/inherits/-/inherits-2.0.4.tgz", + "integrity": "sha512-k/vGaX4/Yla3WzyMCvTQOXYeIHvqOKtnqBduzTHpzpQZzAskKMhZ2K+EnBiSM9zGSoIFeMpXKxa4dYeZIQqewQ==" + }, + "node_modules/ip": { + "version": "2.0.0", + "resolved": "https://registry.npmjs.org/ip/-/ip-2.0.0.tgz", + "integrity": "sha512-WKa+XuLG1A1R0UWhl2+1XQSi+fZWMsYKffMZTTYsiZaUD8k2yDAj5atimTUD2TZkyCkNEeYE5NhFZmupOGtjYQ==" + }, + "node_modules/ipaddr.js": { + "version": "1.9.1", + "resolved": "https://registry.npmjs.org/ipaddr.js/-/ipaddr.js-1.9.1.tgz", + "integrity": "sha512-0KI/607xoxSToH7GjN1FfSbLoU0+btTicjsQSWQlh/hZykN8KpmMf7uYwPW3R+akZ6R/w18ZlXSHBYXiYUPO3g==", + "engines": { + "node": ">= 0.10" + } + }, + "node_modules/kareem": { + "version": "2.5.1", + "resolved": "https://registry.npmjs.org/kareem/-/kareem-2.5.1.tgz", + "integrity": "sha512-7jFxRVm+jD+rkq3kY0iZDJfsO2/t4BBPeEb2qKn2lR/9KhuksYk5hxzfRYWMPV8P/x2d0kHD306YyWLzjjH+uA==", + "engines": { + "node": ">=12.0.0" + } + }, + "node_modules/media-typer": { + "version": "0.3.0", + "resolved": "https://registry.npmjs.org/media-typer/-/media-typer-0.3.0.tgz", + "integrity": "sha512-dq+qelQ9akHpcOl/gUVRTxVIOkAJ1wR3QAvb4RsVjS8oVoFjDGTc679wJYmUmknUF5HwMLOgb5O+a3KxfWapPQ==", + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/memory-pager": { + "version": "1.5.0", + "resolved": "https://registry.npmjs.org/memory-pager/-/memory-pager-1.5.0.tgz", + "integrity": "sha512-ZS4Bp4r/Zoeq6+NLJpP+0Zzm0pR8whtGPf1XExKLJBAczGMnSi3It14OiNCStjQjM6NU1okjQGSxgEZN8eBYKg==", + "optional": true + }, + "node_modules/merge-descriptors": { + "version": "1.0.1", + "resolved": "https://registry.npmjs.org/merge-descriptors/-/merge-descriptors-1.0.1.tgz", + "integrity": "sha512-cCi6g3/Zr1iqQi6ySbseM1Xvooa98N0w31jzUYrXPX2xqObmFGHJ0tQ5u74H3mVh7wLouTseZyYIq39g8cNp1w==" + }, + "node_modules/methods": { + "version": "1.1.2", + "resolved": "https://registry.npmjs.org/methods/-/methods-1.1.2.tgz", + "integrity": "sha512-iclAHeNqNm68zFtnZ0e+1L2yUIdvzNoauKU4WBA3VvH/vPFieF7qfRlwUZU+DA9P9bPXIS90ulxoUoCH23sV2w==", + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/mime": { + "version": "1.6.0", + "resolved": "https://registry.npmjs.org/mime/-/mime-1.6.0.tgz", + "integrity": "sha512-x0Vn8spI+wuJ1O6S7gnbaQg8Pxh4NNHb7KSINmEWKiPE4RKOplvijn+NkmYmmRgP68mc70j2EbeTFRsrswaQeg==", + "bin": { + "mime": "cli.js" + }, + "engines": { + "node": ">=4" + } + }, + "node_modules/mime-db": { + "version": "1.52.0", + "resolved": "https://registry.npmjs.org/mime-db/-/mime-db-1.52.0.tgz", + "integrity": "sha512-sPU4uV7dYlvtWJxwwxHD0PuihVNiE7TyAbQ5SWxDCB9mUYvOgroQOwYQQOKPJ8CIbE+1ETVlOoK1UC2nU3gYvg==", + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/mime-types": { + "version": "2.1.35", + "resolved": "https://registry.npmjs.org/mime-types/-/mime-types-2.1.35.tgz", + "integrity": "sha512-ZDY+bPm5zTTF+YpCrAU9nK0UgICYPT0QtT1NZWFv4s++TNkcgVaT0g6+4R2uI4MjQjzysHB1zxuWL50hzaeXiw==", + "dependencies": { + "mime-db": "1.52.0" + }, + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/mongodb": { + "version": "5.7.0", + "resolved": "https://registry.npmjs.org/mongodb/-/mongodb-5.7.0.tgz", + "integrity": "sha512-zm82Bq33QbqtxDf58fLWBwTjARK3NSvKYjyz997KSy6hpat0prjeX/kxjbPVyZY60XYPDNETaHkHJI2UCzSLuw==", + "dependencies": { + "bson": "^5.4.0", + "mongodb-connection-string-url": "^2.6.0", + "socks": "^2.7.1" + }, + "engines": { + "node": ">=14.20.1" + }, + "optionalDependencies": { + "saslprep": "^1.0.3" + }, + "peerDependencies": { + "@aws-sdk/credential-providers": "^3.201.0", + "@mongodb-js/zstd": "^1.1.0", + "kerberos": "^2.0.1", + "mongodb-client-encryption": ">=2.3.0 <3", + "snappy": "^7.2.2" + }, + "peerDependenciesMeta": { + "@aws-sdk/credential-providers": { + "optional": true + }, + "@mongodb-js/zstd": { + "optional": true + }, + "kerberos": { + "optional": true + }, + "mongodb-client-encryption": { + "optional": true + }, + "snappy": { + "optional": true + } + } + }, + "node_modules/mongodb-connection-string-url": { + "version": "2.6.0", + "resolved": "https://registry.npmjs.org/mongodb-connection-string-url/-/mongodb-connection-string-url-2.6.0.tgz", + "integrity": "sha512-WvTZlI9ab0QYtTYnuMLgobULWhokRjtC7db9LtcVfJ+Hsnyr5eo6ZtNAt3Ly24XZScGMelOcGtm7lSn0332tPQ==", + "dependencies": { + "@types/whatwg-url": "^8.2.1", + "whatwg-url": "^11.0.0" + } + }, + "node_modules/mongoose": { + "version": "7.4.3", + "resolved": "https://registry.npmjs.org/mongoose/-/mongoose-7.4.3.tgz", + "integrity": "sha512-eok0lW6mZJHK2vVSWyJb9tUfPMUuRF3h7YC4pU2K2/YSZBlNDUwvKsHgftMOANbokP2Ry+4ylvzAdW4KjkRFjw==", + "dependencies": { + "bson": "^5.4.0", + "kareem": "2.5.1", + "mongodb": "5.7.0", + "mpath": "0.9.0", + "mquery": "5.0.0", + "ms": "2.1.3", + "sift": "16.0.1" + }, + "engines": { + "node": ">=14.20.1" + }, + "funding": { + "type": "opencollective", + "url": "https://opencollective.com/mongoose" + } + }, + "node_modules/mongoose/node_modules/ms": { + "version": "2.1.3", + "resolved": "https://registry.npmjs.org/ms/-/ms-2.1.3.tgz", + "integrity": "sha512-6FlzubTLZG3J2a/NVCAleEhjzq5oxgHyaCU9yYXvcLsvoVaHJq/s5xXI6/XXP6tz7R9xAOtHnSO/tXtF3WRTlA==" + }, + "node_modules/morgan": { + "version": "1.10.0", + "resolved": "https://registry.npmjs.org/morgan/-/morgan-1.10.0.tgz", + "integrity": "sha512-AbegBVI4sh6El+1gNwvD5YIck7nSA36weD7xvIxG4in80j/UoK8AEGaWnnz8v1GxonMCltmlNs5ZKbGvl9b1XQ==", + "dependencies": { + "basic-auth": "~2.0.1", + "debug": "2.6.9", + "depd": "~2.0.0", + "on-finished": "~2.3.0", + "on-headers": "~1.0.2" + }, + "engines": { + "node": ">= 0.8.0" + } + }, + "node_modules/morgan/node_modules/on-finished": { + "version": "2.3.0", + "resolved": "https://registry.npmjs.org/on-finished/-/on-finished-2.3.0.tgz", + "integrity": "sha512-ikqdkGAAyf/X/gPhXGvfgAytDZtDbr+bkNUJ0N9h5MI/dmdgCs3l6hoHrcUv41sRKew3jIwrp4qQDXiK99Utww==", + "dependencies": { + "ee-first": "1.1.1" + }, + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/mpath": { + "version": "0.9.0", + "resolved": "https://registry.npmjs.org/mpath/-/mpath-0.9.0.tgz", + "integrity": "sha512-ikJRQTk8hw5DEoFVxHG1Gn9T/xcjtdnOKIU1JTmGjZZlg9LST2mBLmcX3/ICIbgJydT2GOc15RnNy5mHmzfSew==", + "engines": { + "node": ">=4.0.0" + } + }, + "node_modules/mquery": { + "version": "5.0.0", + "resolved": "https://registry.npmjs.org/mquery/-/mquery-5.0.0.tgz", + "integrity": "sha512-iQMncpmEK8R8ncT8HJGsGc9Dsp8xcgYMVSbs5jgnm1lFHTZqMJTUWTDx1LBO8+mK3tPNZWFLBghQEIOULSTHZg==", + "dependencies": { + "debug": "4.x" + }, + "engines": { + "node": ">=14.0.0" + } + }, + "node_modules/mquery/node_modules/debug": { + "version": "4.3.4", + "resolved": "https://registry.npmjs.org/debug/-/debug-4.3.4.tgz", + "integrity": "sha512-PRWFHuSU3eDtQJPvnNY7Jcket1j0t5OuOsFzPPzsekD52Zl8qUfFIPEiswXqIvHWGVHOgX+7G/vCNNhehwxfkQ==", + "dependencies": { + "ms": "2.1.2" + }, + "engines": { + "node": ">=6.0" + }, + "peerDependenciesMeta": { + "supports-color": { + "optional": true + } + } + }, + "node_modules/mquery/node_modules/ms": { + "version": "2.1.2", + "resolved": "https://registry.npmjs.org/ms/-/ms-2.1.2.tgz", + "integrity": "sha512-sGkPx+VjMtmA6MX27oA4FBFELFCZZ4S4XqeGOXCv68tT+jb3vk/RyaKWP0PTKyWtmLSM0b+adUTEvbs1PEaH2w==" + }, + "node_modules/ms": { + "version": "2.0.0", + "resolved": "https://registry.npmjs.org/ms/-/ms-2.0.0.tgz", + "integrity": "sha512-Tpp60P6IUJDTuOq/5Z8cdskzJujfwqfOTkrwIwj7IRISpnkJnT6SyJ4PCPnGMoFjC9ddhal5KVIYtAt97ix05A==" + }, + "node_modules/negotiator": { + "version": "0.6.3", + "resolved": "https://registry.npmjs.org/negotiator/-/negotiator-0.6.3.tgz", + "integrity": "sha512-+EUsqGPLsM+j/zdChZjsnX51g4XrHFOIXwfnCVPGlQk/k5giakcKsuxCObBRu6DSm9opw/O6slWbJdghQM4bBg==", + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/object-inspect": { + "version": "1.12.3", + "resolved": "https://registry.npmjs.org/object-inspect/-/object-inspect-1.12.3.tgz", + "integrity": "sha512-geUvdk7c+eizMNUDkRpW1wJwgfOiOeHbxBR/hLXK1aT6zmVSO0jsQcs7fj6MGw89jC/cjGfLcNOrtMYtGqm81g==", + "funding": { + "url": "https://github.com/sponsors/ljharb" + } + }, + "node_modules/on-finished": { + "version": "2.4.1", + "resolved": "https://registry.npmjs.org/on-finished/-/on-finished-2.4.1.tgz", + "integrity": "sha512-oVlzkg3ENAhCk2zdv7IJwd/QUD4z2RxRwpkcGY8psCVcCYZNq4wYnVWALHM+brtuJjePWiYF/ClmuDr8Ch5+kg==", + "dependencies": { + "ee-first": "1.1.1" + }, + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/on-headers": { + "version": "1.0.2", + "resolved": "https://registry.npmjs.org/on-headers/-/on-headers-1.0.2.tgz", + "integrity": "sha512-pZAE+FJLoyITytdqK0U5s+FIpjN0JP3OzFi/u8Rx+EV5/W+JTWGXG8xFzevE7AjBfDqHv/8vL8qQsIhHnqRkrA==", + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/parseurl": { + "version": "1.3.3", + "resolved": "https://registry.npmjs.org/parseurl/-/parseurl-1.3.3.tgz", + "integrity": "sha512-CiyeOxFT/JZyN5m0z9PfXw4SCBJ6Sygz1Dpl0wqjlhDEGGBP1GnsUVEL0p63hoG1fcj3fHynXi9NYO4nWOL+qQ==", + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/path-to-regexp": { + "version": "0.1.7", + "resolved": "https://registry.npmjs.org/path-to-regexp/-/path-to-regexp-0.1.7.tgz", + "integrity": "sha512-5DFkuoqlv1uYQKxy8omFBeJPQcdoE07Kv2sferDCrAq1ohOU+MSDswDIbnx3YAM60qIOnYa53wBhXW0EbMonrQ==" + }, + "node_modules/proxy-addr": { + "version": "2.0.7", + "resolved": "https://registry.npmjs.org/proxy-addr/-/proxy-addr-2.0.7.tgz", + "integrity": "sha512-llQsMLSUDUPT44jdrU/O37qlnifitDP+ZwrmmZcoSKyLKvtZxpyV0n2/bD/N4tBAAZ/gJEdZU7KMraoK1+XYAg==", + "dependencies": { + "forwarded": "0.2.0", + "ipaddr.js": "1.9.1" + }, + "engines": { + "node": ">= 0.10" + } + }, + "node_modules/punycode": { + "version": "2.3.0", + "resolved": "https://registry.npmjs.org/punycode/-/punycode-2.3.0.tgz", + "integrity": "sha512-rRV+zQD8tVFys26lAGR9WUuS4iUAngJScM+ZRSKtvl5tKeZ2t5bvdNFdNHBW9FWR4guGHlgmsZ1G7BSm2wTbuA==", + "engines": { + "node": ">=6" + } + }, + "node_modules/qs": { + "version": "6.11.0", + "resolved": "https://registry.npmjs.org/qs/-/qs-6.11.0.tgz", + "integrity": "sha512-MvjoMCJwEarSbUYk5O+nmoSzSutSsTwF85zcHPQ9OrlFoZOYIjaqBAJIqIXjptyD5vThxGq52Xu/MaJzRkIk4Q==", + "dependencies": { + "side-channel": "^1.0.4" + }, + "engines": { + "node": ">=0.6" + }, + "funding": { + "url": "https://github.com/sponsors/ljharb" + } + }, + "node_modules/range-parser": { + "version": "1.2.1", + "resolved": "https://registry.npmjs.org/range-parser/-/range-parser-1.2.1.tgz", + "integrity": "sha512-Hrgsx+orqoygnmhFbKaHE6c296J+HTAQXoxEF6gNupROmmGJRoyzfG3ccAveqCBrwr/2yxQ5BVd/GTl5agOwSg==", + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/raw-body": { + "version": "2.5.1", + "resolved": "https://registry.npmjs.org/raw-body/-/raw-body-2.5.1.tgz", + "integrity": "sha512-qqJBtEyVgS0ZmPGdCFPWJ3FreoqvG4MVQln/kCgF7Olq95IbOp0/BWyMwbdtn4VTvkM8Y7khCQ2Xgk/tcrCXig==", + "dependencies": { + "bytes": "3.1.2", + "http-errors": "2.0.0", + "iconv-lite": "0.4.24", + "unpipe": "1.0.0" + }, + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/safe-buffer": { + "version": "5.2.1", + "resolved": "https://registry.npmjs.org/safe-buffer/-/safe-buffer-5.2.1.tgz", + "integrity": "sha512-rp3So07KcdmmKbGvgaNxQSJr7bGVSVk5S9Eq1F+ppbRo70+YeaDxkw5Dd8NPN+GD6bjnYm2VuPuCXmpuYvmCXQ==", + "funding": [ + { + "type": "github", + "url": "https://github.com/sponsors/feross" + }, + { + "type": "patreon", + "url": "https://www.patreon.com/feross" + }, + { + "type": "consulting", + "url": "https://feross.org/support" + } + ] + }, + "node_modules/safer-buffer": { + "version": "2.1.2", + "resolved": "https://registry.npmjs.org/safer-buffer/-/safer-buffer-2.1.2.tgz", + "integrity": "sha512-YZo3K82SD7Riyi0E1EQPojLz7kpepnSQI9IyPbHHg1XXXevb5dJI7tpyN2ADxGcQbHG7vcyRHk0cbwqcQriUtg==" + }, + "node_modules/saslprep": { + "version": "1.0.3", + "resolved": "https://registry.npmjs.org/saslprep/-/saslprep-1.0.3.tgz", + "integrity": "sha512-/MY/PEMbk2SuY5sScONwhUDsV2p77Znkb/q3nSVstq/yQzYJOH/Azh29p9oJLsl3LnQwSvZDKagDGBsBwSooag==", + "optional": true, + "dependencies": { + "sparse-bitfield": "^3.0.3" + }, + "engines": { + "node": ">=6" + } + }, + "node_modules/send": { + "version": "0.18.0", + "resolved": "https://registry.npmjs.org/send/-/send-0.18.0.tgz", + "integrity": "sha512-qqWzuOjSFOuqPjFe4NOsMLafToQQwBSOEpS+FwEt3A2V3vKubTquT3vmLTQpFgMXp8AlFWFuP1qKaJZOtPpVXg==", + "dependencies": { + "debug": "2.6.9", + "depd": "2.0.0", + "destroy": "1.2.0", + "encodeurl": "~1.0.2", + "escape-html": "~1.0.3", + "etag": "~1.8.1", + "fresh": "0.5.2", + "http-errors": "2.0.0", + "mime": "1.6.0", + "ms": "2.1.3", + "on-finished": "2.4.1", + "range-parser": "~1.2.1", + "statuses": "2.0.1" + }, + "engines": { + "node": ">= 0.8.0" + } + }, + "node_modules/send/node_modules/ms": { + "version": "2.1.3", + "resolved": "https://registry.npmjs.org/ms/-/ms-2.1.3.tgz", + "integrity": "sha512-6FlzubTLZG3J2a/NVCAleEhjzq5oxgHyaCU9yYXvcLsvoVaHJq/s5xXI6/XXP6tz7R9xAOtHnSO/tXtF3WRTlA==" + }, + "node_modules/serve-static": { + "version": "1.15.0", + "resolved": "https://registry.npmjs.org/serve-static/-/serve-static-1.15.0.tgz", + "integrity": "sha512-XGuRDNjXUijsUL0vl6nSD7cwURuzEgglbOaFuZM9g3kwDXOWVTck0jLzjPzGD+TazWbboZYu52/9/XPdUgne9g==", + "dependencies": { + "encodeurl": "~1.0.2", + "escape-html": "~1.0.3", + "parseurl": "~1.3.3", + "send": "0.18.0" + }, + "engines": { + "node": ">= 0.8.0" + } + }, + "node_modules/setprototypeof": { + "version": "1.2.0", + "resolved": "https://registry.npmjs.org/setprototypeof/-/setprototypeof-1.2.0.tgz", + "integrity": "sha512-E5LDX7Wrp85Kil5bhZv46j8jOeboKq5JMmYM3gVGdGH8xFpPWXUMsNrlODCrkoxMEeNi/XZIwuRvY4XNwYMJpw==" + }, + "node_modules/side-channel": { + "version": "1.0.4", + "resolved": "https://registry.npmjs.org/side-channel/-/side-channel-1.0.4.tgz", + "integrity": "sha512-q5XPytqFEIKHkGdiMIrY10mvLRvnQh42/+GoBlFW3b2LXLE2xxJpZFdm94we0BaoV3RwJyGqg5wS7epxTv0Zvw==", + "dependencies": { + "call-bind": "^1.0.0", + "get-intrinsic": "^1.0.2", + "object-inspect": "^1.9.0" + }, + "funding": { + "url": "https://github.com/sponsors/ljharb" + } + }, + "node_modules/sift": { + "version": "16.0.1", + "resolved": "https://registry.npmjs.org/sift/-/sift-16.0.1.tgz", + "integrity": "sha512-Wv6BjQ5zbhW7VFefWusVP33T/EM0vYikCaQ2qR8yULbsilAT8/wQaXvuQ3ptGLpoKx+lihJE3y2UTgKDyyNHZQ==" + }, + "node_modules/smart-buffer": { + "version": "4.2.0", + "resolved": "https://registry.npmjs.org/smart-buffer/-/smart-buffer-4.2.0.tgz", + "integrity": "sha512-94hK0Hh8rPqQl2xXc3HsaBoOXKV20MToPkcXvwbISWLEs+64sBq5kFgn2kJDHb1Pry9yrP0dxrCI9RRci7RXKg==", + "engines": { + "node": ">= 6.0.0", + "npm": ">= 3.0.0" + } + }, + "node_modules/socks": { + "version": "2.7.1", + "resolved": "https://registry.npmjs.org/socks/-/socks-2.7.1.tgz", + "integrity": "sha512-7maUZy1N7uo6+WVEX6psASxtNlKaNVMlGQKkG/63nEDdLOWNbiUMoLK7X4uYoLhQstau72mLgfEWcXcwsaHbYQ==", + "dependencies": { + "ip": "^2.0.0", + "smart-buffer": "^4.2.0" + }, + "engines": { + "node": ">= 10.13.0", + "npm": ">= 3.0.0" + } + }, + "node_modules/sparse-bitfield": { + "version": "3.0.3", + "resolved": "https://registry.npmjs.org/sparse-bitfield/-/sparse-bitfield-3.0.3.tgz", + "integrity": "sha512-kvzhi7vqKTfkh0PZU+2D2PIllw2ymqJKujUcyPMd9Y75Nv4nPbGJZXNhxsgdQab2BmlDct1YnfQCguEvHr7VsQ==", + "optional": true, + "dependencies": { + "memory-pager": "^1.0.2" + } + }, + "node_modules/statuses": { + "version": "2.0.1", + "resolved": "https://registry.npmjs.org/statuses/-/statuses-2.0.1.tgz", + "integrity": "sha512-RwNA9Z/7PrK06rYLIzFMlaF+l73iwpzsqRIFgbMLbTcLD6cOao82TaWefPXQvB2fOC4AjuYSEndS7N/mTCbkdQ==", + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/step": { + "version": "1.0.0", + "resolved": "https://registry.npmjs.org/step/-/step-1.0.0.tgz", + "integrity": "sha512-sqFPUYBKa+XDdUz76UbHwUYP9eJ4i4aj/pm+4GkF1UQs5CcIdifZsGpyO3FASFv/ET2DtWLcrL8KfhjWwwwkfg==" + }, + "node_modules/toidentifier": { + "version": "1.0.1", + "resolved": "https://registry.npmjs.org/toidentifier/-/toidentifier-1.0.1.tgz", + "integrity": "sha512-o5sSPKEkg/DIQNmH43V0/uerLrpzVedkUh8tGNvaeXpfpuwjKenlSox/2O/BTlZUtEe+JG7s5YhEz608PlAHRA==", + "engines": { + "node": ">=0.6" + } + }, + "node_modules/tr46": { + "version": "3.0.0", + "resolved": "https://registry.npmjs.org/tr46/-/tr46-3.0.0.tgz", + "integrity": "sha512-l7FvfAHlcmulp8kr+flpQZmVwtu7nfRV7NZujtN0OqES8EL4O4e0qqzL0DC5gAvx/ZC/9lk6rhcUwYvkBnBnYA==", + "dependencies": { + "punycode": "^2.1.1" + }, + "engines": { + "node": ">=12" + } + }, + "node_modules/type-is": { + "version": "1.6.18", + "resolved": "https://registry.npmjs.org/type-is/-/type-is-1.6.18.tgz", + "integrity": "sha512-TkRKr9sUTxEH8MdfuCSP7VizJyzRNMjj2J2do2Jr3Kym598JVdEksuzPQCnlFPW4ky9Q+iA+ma9BGm06XQBy8g==", + "dependencies": { + "media-typer": "0.3.0", + "mime-types": "~2.1.24" + }, + "engines": { + "node": ">= 0.6" + } + }, + "node_modules/unpipe": { + "version": "1.0.0", + "resolved": "https://registry.npmjs.org/unpipe/-/unpipe-1.0.0.tgz", + "integrity": "sha512-pjy2bYhSsufwWlKwPc+l3cN7+wuJlK6uz0YdJEOlQDbl6jo/YlPi4mb8agUkVC8BF7V8NuzeyPNqRksA3hztKQ==", + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/utils-merge": { + "version": "1.0.1", + "resolved": "https://registry.npmjs.org/utils-merge/-/utils-merge-1.0.1.tgz", + "integrity": "sha512-pMZTvIkT1d+TFGvDOqodOclx0QWkkgi6Tdoa8gC8ffGAAqz9pzPTZWAybbsHHoED/ztMtkv/VoYTYyShUn81hA==", + "engines": { + "node": ">= 0.4.0" + } + }, + "node_modules/vary": { + "version": "1.1.2", + "resolved": "https://registry.npmjs.org/vary/-/vary-1.1.2.tgz", + "integrity": "sha512-BNGbWLfd0eUPabhkXUVm0j8uuvREyTh5ovRa/dyow/BqAbZJyC+5fU+IzQOzmAKzYqYRAISoRhdQr3eIZ/PXqg==", + "engines": { + "node": ">= 0.8" + } + }, + "node_modules/webidl-conversions": { + "version": "7.0.0", + "resolved": "https://registry.npmjs.org/webidl-conversions/-/webidl-conversions-7.0.0.tgz", + "integrity": "sha512-VwddBukDzu71offAQR975unBIGqfKZpM+8ZX6ySk8nYhVoo5CYaZyzt3YBvYtRtO+aoGlqxPg/B87NGVZ/fu6g==", + "engines": { + "node": ">=12" + } + }, + "node_modules/whatwg-url": { + "version": "11.0.0", + "resolved": "https://registry.npmjs.org/whatwg-url/-/whatwg-url-11.0.0.tgz", + "integrity": "sha512-RKT8HExMpoYx4igMiVMY83lN6UeITKJlBQ+vR/8ZJ8OCdSiN3RwCq+9gH0+Xzj0+5IrM6i4j/6LuvzbZIQgEcQ==", + "dependencies": { + "tr46": "^3.0.0", + "webidl-conversions": "^7.0.0" + }, + "engines": { + "node": ">=12" + } + } + } +} diff --git a/package.json b/package.json new file mode 100644 index 0000000..86ef1d5 --- /dev/null +++ b/package.json @@ -0,0 +1,21 @@ +{ + "name": "app", + "version": "1.0.0", + "description": "", + "main": "index.js", + "scripts": { + "start": "node index.js", + "dev": "nodemon index.js", + "test": "echo \"Error: no test specified\" && exit 1" + }, + "keywords": [], + "author": "", + "license": "ISC", + "dependencies": { + "dotenv": "^16.3.1", + "express": "^4.18.2", + "index": "^0.4.0", + "mongoose": "^7.4.3", + "morgan": "^1.10.0" + } +} diff --git a/public/data.json b/public/data.json new file mode 100644 index 0000000..894407d --- /dev/null +++ b/public/data.json @@ -0,0 +1,555 @@ +{ + "products": [ + { + "id": 1, + "title": "iPhone 9", + "description": "An apple mobile which is nothing like apple", + "price": 549, + "discountPercentage": 12.96, + "rating": 4.69, + "stock": 94, + "brand": "Apple", + "category": "smartphones", + "thumbnail": "https://i.dummyjson.com/data/products/1/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/1/1.jpg", + "https://i.dummyjson.com/data/products/1/2.jpg", + "https://i.dummyjson.com/data/products/1/3.jpg", + "https://i.dummyjson.com/data/products/1/4.jpg", + "https://i.dummyjson.com/data/products/1/thumbnail.jpg" + ] + }, + { + "id": 2, + "title": "iPhone X", + "description": "SIM-Free, Model A19211 6.5-inch Super Retina HD display with OLED technology A12 Bionic chip with ...", + "price": 899, + "discountPercentage": 17.94, + "rating": 4.44, + "stock": 34, + "brand": "Apple", + "category": "smartphones", + "thumbnail": "https://i.dummyjson.com/data/products/2/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/2/1.jpg", + "https://i.dummyjson.com/data/products/2/2.jpg", + "https://i.dummyjson.com/data/products/2/3.jpg", + "https://i.dummyjson.com/data/products/2/thumbnail.jpg" + ] + }, + { + "id": 3, + "title": "Samsung Universe 9", + "description": "Samsung's new variant which goes beyond Galaxy to the Universe", + "price": 1249, + "discountPercentage": 15.46, + "rating": 4.09, + "stock": 36, + "brand": "Samsung", + "category": "smartphones", + "thumbnail": "https://i.dummyjson.com/data/products/3/thumbnail.jpg", + "images": ["https://i.dummyjson.com/data/products/3/1.jpg"] + }, + { + "id": 4, + "title": "OPPOF19", + "description": "OPPO F19 is officially announced on April 2021.", + "price": 280, + "discountPercentage": 17.91, + "rating": 4.3, + "stock": 123, + "brand": "OPPO", + "category": "smartphones", + "thumbnail": "https://i.dummyjson.com/data/products/4/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/4/1.jpg", + "https://i.dummyjson.com/data/products/4/2.jpg", + "https://i.dummyjson.com/data/products/4/3.jpg", + "https://i.dummyjson.com/data/products/4/4.jpg", + "https://i.dummyjson.com/data/products/4/thumbnail.jpg" + ] + }, + { + "id": 5, + "title": "Huawei P30", + "description": "Huawei’s re-badged P30 Pro New Edition was officially unveiled yesterday in Germany and now the device has made its way to the UK.", + "price": 499, + "discountPercentage": 10.58, + "rating": 4.09, + "stock": 32, + "brand": "Huawei", + "category": "smartphones", + "thumbnail": "https://i.dummyjson.com/data/products/5/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/5/1.jpg", + "https://i.dummyjson.com/data/products/5/2.jpg", + "https://i.dummyjson.com/data/products/5/3.jpg" + ] + }, + { + "id": 6, + "title": "MacBook Pro", + "description": "MacBook Pro 2021 with mini-LED display may launch between September, November", + "price": 1749, + "discountPercentage": 11.02, + "rating": 4.57, + "stock": 83, + "brand": "Apple", + "category": "laptops", + "thumbnail": "https://i.dummyjson.com/data/products/6/thumbnail.png", + "images": [ + "https://i.dummyjson.com/data/products/6/1.png", + "https://i.dummyjson.com/data/products/6/2.jpg", + "https://i.dummyjson.com/data/products/6/3.png", + "https://i.dummyjson.com/data/products/6/4.jpg" + ] + }, + { + "id": 7, + "title": "Samsung Galaxy Book", + "description": "Samsung Galaxy Book S (2020) Laptop With Intel Lakefield Chip, 8GB of RAM Launched", + "price": 1499, + "discountPercentage": 4.15, + "rating": 4.25, + "stock": 50, + "brand": "Samsung", + "category": "laptops", + "thumbnail": "https://i.dummyjson.com/data/products/7/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/7/1.jpg", + "https://i.dummyjson.com/data/products/7/2.jpg", + "https://i.dummyjson.com/data/products/7/3.jpg", + "https://i.dummyjson.com/data/products/7/thumbnail.jpg" + ] + }, + { + "id": 8, + "title": "Microsoft Surface Laptop 4", + "description": "Style and speed. Stand out on HD video calls backed by Studio Mics. Capture ideas on the vibrant touchscreen.", + "price": 1499, + "discountPercentage": 10.23, + "rating": 4.43, + "stock": 68, + "brand": "Microsoft Surface", + "category": "laptops", + "thumbnail": "https://i.dummyjson.com/data/products/8/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/8/1.jpg", + "https://i.dummyjson.com/data/products/8/2.jpg", + "https://i.dummyjson.com/data/products/8/3.jpg", + "https://i.dummyjson.com/data/products/8/4.jpg", + "https://i.dummyjson.com/data/products/8/thumbnail.jpg" + ] + }, + { + "id": 9, + "title": "Infinix INBOOK", + "description": "Infinix Inbook X1 Ci3 10th 8GB 256GB 14 Win10 Grey – 1 Year Warranty", + "price": 1099, + "discountPercentage": 11.83, + "rating": 4.54, + "stock": 96, + "brand": "Infinix", + "category": "laptops", + "thumbnail": "https://i.dummyjson.com/data/products/9/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/9/1.jpg", + "https://i.dummyjson.com/data/products/9/2.png", + "https://i.dummyjson.com/data/products/9/3.png", + "https://i.dummyjson.com/data/products/9/4.jpg", + "https://i.dummyjson.com/data/products/9/thumbnail.jpg" + ] + }, + { + "id": 10, + "title": "HP Pavilion 15-DK1056WM", + "description": "HP Pavilion 15-DK1056WM Gaming Laptop 10th Gen Core i5, 8GB, 256GB SSD, GTX 1650 4GB, Windows 10", + "price": 1099, + "discountPercentage": 6.18, + "rating": 4.43, + "stock": 89, + "brand": "HP Pavilion", + "category": "laptops", + "thumbnail": "https://i.dummyjson.com/data/products/10/thumbnail.jpeg", + "images": [ + "https://i.dummyjson.com/data/products/10/1.jpg", + "https://i.dummyjson.com/data/products/10/2.jpg", + "https://i.dummyjson.com/data/products/10/3.jpg", + "https://i.dummyjson.com/data/products/10/thumbnail.jpeg" + ] + }, + { + "id": 11, + "title": "perfume Oil", + "description": "Mega Discount, Impression of Acqua Di Gio by GiorgioArmani concentrated attar perfume Oil", + "price": 13, + "discountPercentage": 8.4, + "rating": 4.26, + "stock": 65, + "brand": "Impression of Acqua Di Gio", + "category": "fragrances", + "thumbnail": "https://i.dummyjson.com/data/products/11/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/11/1.jpg", + "https://i.dummyjson.com/data/products/11/2.jpg", + "https://i.dummyjson.com/data/products/11/3.jpg", + "https://i.dummyjson.com/data/products/11/thumbnail.jpg" + ] + }, + { + "id": 12, + "title": "Brown Perfume", + "description": "Royal_Mirage Sport Brown Perfume for Men & Women - 120ml", + "price": 40, + "discountPercentage": 15.66, + "rating": 4, + "stock": 52, + "brand": "Royal_Mirage", + "category": "fragrances", + "thumbnail": "https://i.dummyjson.com/data/products/12/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/12/1.jpg", + "https://i.dummyjson.com/data/products/12/2.jpg", + "https://i.dummyjson.com/data/products/12/3.png", + "https://i.dummyjson.com/data/products/12/4.jpg", + "https://i.dummyjson.com/data/products/12/thumbnail.jpg" + ] + }, + { + "id": 13, + "title": "Fog Scent Xpressio Perfume", + "description": "Product details of Best Fog Scent Xpressio Perfume 100ml For Men cool long lasting perfumes for Men", + "price": 13, + "discountPercentage": 8.14, + "rating": 4.59, + "stock": 61, + "brand": "Fog Scent Xpressio", + "category": "fragrances", + "thumbnail": "https://i.dummyjson.com/data/products/13/thumbnail.webp", + "images": [ + "https://i.dummyjson.com/data/products/13/1.jpg", + "https://i.dummyjson.com/data/products/13/2.png", + "https://i.dummyjson.com/data/products/13/3.jpg", + "https://i.dummyjson.com/data/products/13/4.jpg", + "https://i.dummyjson.com/data/products/13/thumbnail.webp" + ] + }, + { + "id": 14, + "title": "Non-Alcoholic Concentrated Perfume Oil", + "description": "Original Al Munakh® by Mahal Al Musk | Our Impression of Climate | 6ml Non-Alcoholic Concentrated Perfume Oil", + "price": 120, + "discountPercentage": 15.6, + "rating": 4.21, + "stock": 114, + "brand": "Al Munakh", + "category": "fragrances", + "thumbnail": "https://i.dummyjson.com/data/products/14/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/14/1.jpg", + "https://i.dummyjson.com/data/products/14/2.jpg", + "https://i.dummyjson.com/data/products/14/3.jpg", + "https://i.dummyjson.com/data/products/14/thumbnail.jpg" + ] + }, + { + "id": 15, + "title": "Eau De Perfume Spray", + "description": "Genuine Al-Rehab spray perfume from UAE/Saudi Arabia/Yemen High Quality", + "price": 30, + "discountPercentage": 10.99, + "rating": 4.7, + "stock": 105, + "brand": "Lord - Al-Rehab", + "category": "fragrances", + "thumbnail": "https://i.dummyjson.com/data/products/15/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/15/1.jpg", + "https://i.dummyjson.com/data/products/15/2.jpg", + "https://i.dummyjson.com/data/products/15/3.jpg", + "https://i.dummyjson.com/data/products/15/4.jpg", + "https://i.dummyjson.com/data/products/15/thumbnail.jpg" + ] + }, + { + "id": 16, + "title": "Hyaluronic Acid Serum", + "description": "L'Oréal Paris introduces Hyaluron Expert Replumping Serum formulated with 1.5% Hyaluronic Acid", + "price": 19, + "discountPercentage": 13.31, + "rating": 4.83, + "stock": 110, + "brand": "L'Oreal Paris", + "category": "skincare", + "thumbnail": "https://i.dummyjson.com/data/products/16/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/16/1.png", + "https://i.dummyjson.com/data/products/16/2.webp", + "https://i.dummyjson.com/data/products/16/3.jpg", + "https://i.dummyjson.com/data/products/16/4.jpg", + "https://i.dummyjson.com/data/products/16/thumbnail.jpg" + ] + }, + { + "id": 17, + "title": "Tree Oil 30ml", + "description": "Tea tree oil contains a number of compounds, including terpinen-4-ol, that have been shown to kill certain bacteria,", + "price": 12, + "discountPercentage": 4.09, + "rating": 4.52, + "stock": 78, + "brand": "Hemani Tea", + "category": "skincare", + "thumbnail": "https://i.dummyjson.com/data/products/17/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/17/1.jpg", + "https://i.dummyjson.com/data/products/17/2.jpg", + "https://i.dummyjson.com/data/products/17/3.jpg", + "https://i.dummyjson.com/data/products/17/thumbnail.jpg" + ] + }, + { + "id": 18, + "title": "Oil Free Moisturizer 100ml", + "description": "Dermive Oil Free Moisturizer with SPF 20 is specifically formulated with ceramides, hyaluronic acid & sunscreen.", + "price": 40, + "discountPercentage": 13.1, + "rating": 4.56, + "stock": 88, + "brand": "Dermive", + "category": "skincare", + "thumbnail": "https://i.dummyjson.com/data/products/18/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/18/1.jpg", + "https://i.dummyjson.com/data/products/18/2.jpg", + "https://i.dummyjson.com/data/products/18/3.jpg", + "https://i.dummyjson.com/data/products/18/4.jpg", + "https://i.dummyjson.com/data/products/18/thumbnail.jpg" + ] + }, + { + "id": 19, + "title": "Skin Beauty Serum.", + "description": "Product name: rorec collagen hyaluronic acid white face serum riceNet weight: 15 m", + "price": 46, + "discountPercentage": 10.68, + "rating": 4.42, + "stock": 54, + "brand": "ROREC White Rice", + "category": "skincare", + "thumbnail": "https://i.dummyjson.com/data/products/19/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/19/1.jpg", + "https://i.dummyjson.com/data/products/19/2.jpg", + "https://i.dummyjson.com/data/products/19/3.png", + "https://i.dummyjson.com/data/products/19/thumbnail.jpg" + ] + }, + { + "id": 20, + "title": "Freckle Treatment Cream- 15gm", + "description": "Fair & Clear is Pakistan's only pure Freckle cream which helpsfade Freckles, Darkspots and pigments. Mercury level is 0%, so there are no side effects.", + "price": 70, + "discountPercentage": 16.99, + "rating": 4.06, + "stock": 140, + "brand": "Fair & Clear", + "category": "skincare", + "thumbnail": "https://i.dummyjson.com/data/products/20/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/20/1.jpg", + "https://i.dummyjson.com/data/products/20/2.jpg", + "https://i.dummyjson.com/data/products/20/3.jpg", + "https://i.dummyjson.com/data/products/20/4.jpg", + "https://i.dummyjson.com/data/products/20/thumbnail.jpg" + ] + }, + { + "id": 21, + "title": "- Daal Masoor 500 grams", + "description": "Fine quality Branded Product Keep in a cool and dry place", + "price": 20, + "discountPercentage": 4.81, + "rating": 4.44, + "stock": 133, + "brand": "Saaf & Khaas", + "category": "groceries", + "thumbnail": "https://i.dummyjson.com/data/products/21/thumbnail.png", + "images": [ + "https://i.dummyjson.com/data/products/21/1.png", + "https://i.dummyjson.com/data/products/21/2.jpg", + "https://i.dummyjson.com/data/products/21/3.jpg" + ] + }, + { + "id": 22, + "title": "Elbow Macaroni - 400 gm", + "description": "Product details of Bake Parlor Big Elbow Macaroni - 400 gm", + "price": 14, + "discountPercentage": 15.58, + "rating": 4.57, + "stock": 146, + "brand": "Bake Parlor Big", + "category": "groceries", + "thumbnail": "https://i.dummyjson.com/data/products/22/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/22/1.jpg", + "https://i.dummyjson.com/data/products/22/2.jpg", + "https://i.dummyjson.com/data/products/22/3.jpg" + ] + }, + { + "id": 23, + "title": "Orange Essence Food Flavou", + "description": "Specifications of Orange Essence Food Flavour For Cakes and Baking Food Item", + "price": 14, + "discountPercentage": 8.04, + "rating": 4.85, + "stock": 26, + "brand": "Baking Food Items", + "category": "groceries", + "thumbnail": "https://i.dummyjson.com/data/products/23/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/23/1.jpg", + "https://i.dummyjson.com/data/products/23/2.jpg", + "https://i.dummyjson.com/data/products/23/3.jpg", + "https://i.dummyjson.com/data/products/23/4.jpg", + "https://i.dummyjson.com/data/products/23/thumbnail.jpg" + ] + }, + { + "id": 24, + "title": "cereals muesli fruit nuts", + "description": "original fauji cereal muesli 250gm box pack original fauji cereals muesli fruit nuts flakes breakfast cereal break fast faujicereals cerels cerel foji fouji", + "price": 46, + "discountPercentage": 16.8, + "rating": 4.94, + "stock": 113, + "brand": "fauji", + "category": "groceries", + "thumbnail": "https://i.dummyjson.com/data/products/24/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/24/1.jpg", + "https://i.dummyjson.com/data/products/24/2.jpg", + "https://i.dummyjson.com/data/products/24/3.jpg", + "https://i.dummyjson.com/data/products/24/4.jpg", + "https://i.dummyjson.com/data/products/24/thumbnail.jpg" + ] + }, + { + "id": 25, + "title": "Gulab Powder 50 Gram", + "description": "Dry Rose Flower Powder Gulab Powder 50 Gram • Treats Wounds", + "price": 70, + "discountPercentage": 13.58, + "rating": 4.87, + "stock": 47, + "brand": "Dry Rose", + "category": "groceries", + "thumbnail": "https://i.dummyjson.com/data/products/25/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/25/1.png", + "https://i.dummyjson.com/data/products/25/2.jpg", + "https://i.dummyjson.com/data/products/25/3.png", + "https://i.dummyjson.com/data/products/25/4.jpg", + "https://i.dummyjson.com/data/products/25/thumbnail.jpg" + ] + }, + { + "id": 26, + "title": "Plant Hanger For Home", + "description": "Boho Decor Plant Hanger For Home Wall Decoration Macrame Wall Hanging Shelf", + "price": 41, + "discountPercentage": 17.86, + "rating": 4.08, + "stock": 131, + "brand": "Boho Decor", + "category": "home-decoration", + "thumbnail": "https://i.dummyjson.com/data/products/26/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/26/1.jpg", + "https://i.dummyjson.com/data/products/26/2.jpg", + "https://i.dummyjson.com/data/products/26/3.jpg", + "https://i.dummyjson.com/data/products/26/4.jpg", + "https://i.dummyjson.com/data/products/26/5.jpg", + "https://i.dummyjson.com/data/products/26/thumbnail.jpg" + ] + }, + { + "id": 27, + "title": "Flying Wooden Bird", + "description": "Package Include 6 Birds with Adhesive Tape Shape: 3D Shaped Wooden Birds Material: Wooden MDF, Laminated 3.5mm", + "price": 51, + "discountPercentage": 15.58, + "rating": 4.41, + "stock": 17, + "brand": "Flying Wooden", + "category": "home-decoration", + "thumbnail": "https://i.dummyjson.com/data/products/27/thumbnail.webp", + "images": [ + "https://i.dummyjson.com/data/products/27/1.jpg", + "https://i.dummyjson.com/data/products/27/2.jpg", + "https://i.dummyjson.com/data/products/27/3.jpg", + "https://i.dummyjson.com/data/products/27/4.jpg", + "https://i.dummyjson.com/data/products/27/thumbnail.webp" + ] + }, + { + "id": 28, + "title": "3D Embellishment Art Lamp", + "description": "3D led lamp sticker Wall sticker 3d wall art light on/off button cell operated (included)", + "price": 20, + "discountPercentage": 16.49, + "rating": 4.82, + "stock": 54, + "brand": "LED Lights", + "category": "home-decoration", + "thumbnail": "https://i.dummyjson.com/data/products/28/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/28/1.jpg", + "https://i.dummyjson.com/data/products/28/2.jpg", + "https://i.dummyjson.com/data/products/28/3.png", + "https://i.dummyjson.com/data/products/28/4.jpg", + "https://i.dummyjson.com/data/products/28/thumbnail.jpg" + ] + }, + { + "id": 29, + "title": "Handcraft Chinese style", + "description": "Handcraft Chinese style art luxury palace hotel villa mansion home decor ceramic vase with brass fruit plate", + "price": 60, + "discountPercentage": 15.34, + "rating": 4.44, + "stock": 7, + "brand": "luxury palace", + "category": "home-decoration", + "thumbnail": "https://i.dummyjson.com/data/products/29/thumbnail.webp", + "images": [ + "https://i.dummyjson.com/data/products/29/1.jpg", + "https://i.dummyjson.com/data/products/29/2.jpg", + "https://i.dummyjson.com/data/products/29/3.webp", + "https://i.dummyjson.com/data/products/29/4.webp", + "https://i.dummyjson.com/data/products/29/thumbnail.webp" + ] + }, + { + "id": 30, + "title": "Key Holder", + "description": "Attractive DesignMetallic materialFour key hooksReliable & DurablePremium Quality", + "price": 30, + "discountPercentage": 2.92, + "rating": 4.92, + "stock": 54, + "brand": "Golden", + "category": "home-decoration", + "thumbnail": "https://i.dummyjson.com/data/products/30/thumbnail.jpg", + "images": [ + "https://i.dummyjson.com/data/products/30/1.jpg", + "https://i.dummyjson.com/data/products/30/2.jpg", + "https://i.dummyjson.com/data/products/30/3.jpg", + "https://i.dummyjson.com/data/products/30/thumbnail.jpg" + ] + } + ] + +} diff --git a/public/index.html b/public/index.html new file mode 100644 index 0000000..1826a18 --- /dev/null +++ b/public/index.html @@ -0,0 +1,256 @@ +<link + rel="stylesheet" + href="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css" +/> +<link + rel="stylesheet" + href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css" +/> +<style> + body { + background-color: #eee; + } + .container { + width: 900px; + } + + .card { + background-color: #fff; + border: none; + border-radius: 10px; + width: 250px; + box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19); + } + + .image-container { + position: relative; + } + + .thumbnail-image { + border-radius: 10px !important; + } + + .discount { + background-color: red; + padding-top: 1px; + padding-bottom: 1px; + padding-left: 4px; + padding-right: 4px; + font-size: 10px; + border-radius: 6px; + color: #fff; + } + + .wishlist { + height: 25px; + width: 25px; + background-color: #eee; + display: flex; + justify-content: center; + align-items: center; + border-radius: 50%; + box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19); + } + + .first { + position: absolute; + width: 100%; + padding: 9px; + } + + .dress-name { + font-size: 13px; + font-weight: bold; + width: 75%; + } + + .new-price { + font-size: 13px; + font-weight: bold; + color: red; + } + .old-price { + font-size: 8px; + font-weight: bold; + color: grey; + } + + .btn { + width: 14px; + height: 14px; + border-radius: 50%; + padding: 3px; + } + + .creme { + background-color: #fff; + border: 2px solid grey; + } + .creme:hover { + border: 3px solid grey; + } + + .creme:focus { + background-color: grey; + } + + .red { + background-color: #fff; + border: 2px solid red; + } + + .red:hover { + border: 3px solid red; + } + .red:focus { + background-color: red; + } + + .blue { + background-color: #fff; + border: 2px solid #40c4ff; + } + + .blue:hover { + border: 3px solid #40c4ff; + } + .blue:focus { + background-color: #40c4ff; + } + .darkblue { + background-color: #fff; + border: 2px solid #01579b; + } + .darkblue:hover { + border: 3px solid #01579b; + } + .darkblue:focus { + background-color: #01579b; + } + .yellow { + background-color: #fff; + border: 2px solid #ffca28; + } + .yellow:hover { + border-radius: 3px solid #ffca28; + } + .yellow:focus { + background-color: #ffca28; + } + + .item-size { + width: 15px; + height: 15px; + border-radius: 50%; + background: #fff; + border: 1px solid grey; + color: grey; + font-size: 10px; + text-align: center; + align-items: center; + display: flex; + justify-content: center; + } + + .rating-star { + font-size: 10px !important; + } + .rating-number { + font-size: 10px; + color: grey; + } + .buy { + font-size: 12px; + color: purple; + font-weight: 500; + } + + .voutchers { + background-color: #fff; + border: none; + border-radius: 10px; + width: 190px; + box-shadow: 0 4px 8px 0 rgba(0, 0, 0, 0.2), 0 6px 20px 0 rgba(0, 0, 0, 0.19); + overflow: hidden; + } + .voutcher-divider { + display: flex; + } + .voutcher-left { + width: 60%; + } + .voutcher-name { + color: grey; + font-size: 9px; + font-weight: 500; + } + .voutcher-code { + color: red; + font-size: 11px; + font-weight: bold; + } + .voutcher-right { + width: 40%; + background-color: purple; + color: #fff; + } + + .discount-percent { + font-size: 12px; + font-weight: bold; + position: relative; + top: 5px; + } + .off { + font-size: 14px; + position: relative; + bottom: 5px; + } +</style> + +<div class="container mt-5"> + <div class="row"> + <div class="col-md-3"> + <div class="card"> + <div class="image-container"> + <div class="first"> + <div class="d-flex justify-content-between align-items-center"> + <span class="discount">-25%</span> + <span class="wishlist"><i class="fa fa-heart-o"></i></span> + </div> + </div> + + <img + src="**url**" + class="img-fluid rounded thumbnail-image" + /> + </div> + + <div class="product-detail-container p-2"> + <div class="d-flex justify-content-between align-items-center"> + <h5 class="dress-name">**title**</h5> + + <div class="d-flex flex-column mb-2"> + <span class="new-price">₹ **price**</span> + <small class="old-price text-right">₹ 700</small> + </div> + </div> + + + + <div class="d-flex justify-content-between align-items-center pt-1"> + <div> + <i class="fa fa-star-o rating-star"></i> + <span class="rating-number">**rating**</span> + </div> + + <span class="buy">BUY +</span> + </div> + </div> + </div> + + + </div> + + </div> +</div> diff --git a/routes/product.js b/routes/product.js new file mode 100644 index 0000000..5abf881 --- /dev/null +++ b/routes/product.js @@ -0,0 +1,14 @@ +const express = require('express'); +const productController = require('../controller/product'); + +const router = express.Router(); + +router + .post('/', productController.createProduct) + .get('/', productController.getAllProducts) + .get('/:id', productController.getProduct) + .put('/:id', productController.replaceProduct) + .patch('/:id', productController.updateProduct) + .delete('/:id', productController.deleteProduct); + +exports.router = router; \ No newline at end of file diff --git a/routes/user.js b/routes/user.js new file mode 100644 index 0000000..66a8c11 --- /dev/null +++ b/routes/user.js @@ -0,0 +1,14 @@ +const express = require('express'); +const userController = require('../controller/user'); + +const router = express.Router(); + +router + .post('/', userController.createUser) + .get('/', userController.getAllUsers) + .get('/:id', userController.getUser) + .put('/:id', userController.replaceUser) + .patch('/:id', userController.updateUser) + .delete('/:id', userController.deleteUser); + +exports.router = router; \ No newline at end of file