You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Auto merge of #1913 - DSpeckhals:email-preference-front-end, r=carols10cents
front-end: Add email notification preferences
This commit adds the front-end capabilities to coincide with the API endpoint that was built on the server. There's also a small bug fixed in the api-token-row; it was throwing an error every time the "/me" route was loaded.
This PR addresses the second item in #1895.
I like how the UI ended up, but it may very well be creators bias 😄. If this doesn't work, then I'll be happy to iterate!
### Screenshot of a crate with notifications on and one that's off
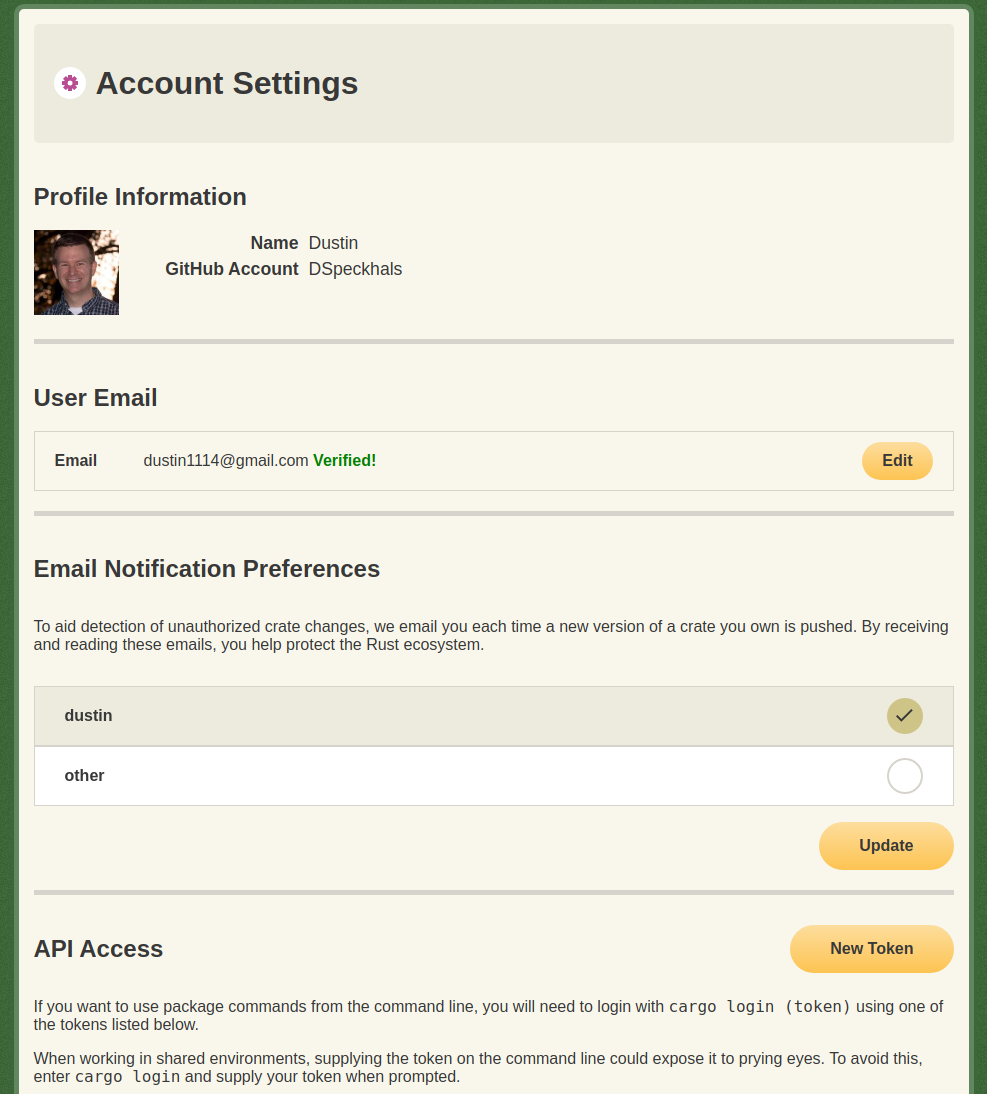
0 commit comments